In this tutorial, we’ll explore how to create and customize a Tkinter window in Python.
You’ll learn how to change the window’s title, resize it, set transparency, modify the stacking order, and even update the window icon.
Let’s get started with some easy examples!
Tkinter Window in Python
Contents
Getting Started with a Basic Tkinter Window
To create a simple Tkinter window, you need to import the tkinter
module and initialize the main window.
Here’s a basic example:
import tkinter as tk
root = tk.Tk() # Create the root window
root.mainloop() # Run the application
When you run this code, a basic Tkinter window will pop up.
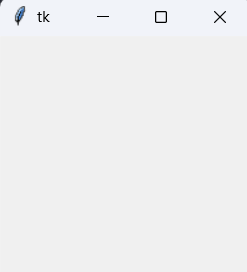
By default, the window will have the title “tk” and include the Minimize, Maximize, and Close buttons.
Changing the Window Title In Tkinter
You can easily change the window title using the title()
method:
root.title('My Tkinter Window')
Here’s a complete example:
import tkinter as tk
root = tk.Tk()
root.title('Tkinter Window Demo') # Set a new window title
root.mainloop()
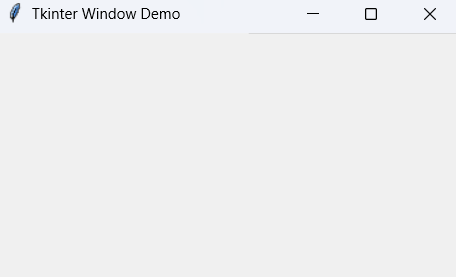
Now, the window title will be updated to “Tkinter Window Demo.”
Adjusting Window Size and Position In Tkinter
In Tkinter, you can control the window’s size and position using the geometry()
method. The syntax is as follows:window.geometry('widthxheight±x±y')
- width: Width of the window in pixels
- height: Height of the window in pixels
- x: Distance from the left edge of the screen
- y: Distance from the top of the screen
Example:
root.geometry('600x400+50+50') # Set size to 600x400 and position at (50, 50)
This will create a window that’s 600 pixels wide, 400 pixels tall, and placed 50 pixels from the top-left corner of the screen.
Centering the Window on the Screen In Tkinter
Want to center the window?
You can calculate the center position based on the screen’s dimensions.
Check out the code below:
import tkinter as tk
root = tk.Tk()
root.title('Centered Tkinter Window')
# Window dimensions
window_width = 300
window_height = 200
# Get the screen width and height
screen_width = root.winfo_screenwidth()
screen_height = root.winfo_screenheight()
# Calculate the center position
center_x = int(screen_width / 2 - window_width / 2)
center_y = int(screen_height / 2 - window_height / 2)
# Set the window geometry
root.geometry(f'{window_width}x{window_height}+{center_x}+{center_y}')
root.mainloop()
winfo_screenwidth()
and winfo_screenheight()
return the width and height of the user’s screen in pixels.
# Calculate the center position
center_x = int(screen_width / 2 - window_width / 2)
center_y = int(screen_height / 2 - window_height / 2)
These lines calculate where the top-left corner of the window should be positioned so that the window appears in the center of the screen. We subtract half of the window’s width and height from the screen’s center coordinates.
root.geometry(f'{window_width}x{window_height}+{center_x}+{center_y}')
This line combines the window size and calculated position to set the window’s geometry. The f-string
dynamically fills in the calculated width, height, and center position.
This will perfectly center the window on your screen!
Tkinter Window in Python Tkinter Window in Python Tkinter Window in Python
Preventing Window Resizing In Tkinter
By default, users can resize Tkinter windows.
To lock the window size, use the resizable()
method:
root.resizable(False, False)
False, False
: Prevents both horizontal and vertical resizingTrue, False
: Allows horizontal resizing onlyFalse, True
: Allows vertical resizing only
Example:
root.geometry('600x400')
root.resizable(False, False) # Fix the window size
Setting Window Transparency In Tkinter
You can make the window transparent using the attributes()
method with the -alpha
attribute.
The value ranges from 0.0 (fully transparent) to 1.0 (fully opaque).
Example:
root.attributes('-alpha', 0.5) # 50% transparency
Keeping the Window Always on Top In Tkinter
To keep the window on top of all other windows, use the -topmost
attribute:
root.attributes('-topmost', 1) # Keep window on top
Changing the Window Icon In Tkinter
The default Tkinter window icon can be changed using the iconbitmap()
method.
You’ll need an .ico image file.
Example:
root.iconbitmap('path/to/your_icon.ico') # Set custom icon
To convert other image formats (like PNG or JPG) to .ico, you can use online tools.
Changing Window Stacking Order In Tkinter
In Tkinter, windows follow a stacking order (like layers).
You can control this using lift()
and lower()
methods:
root.lift() # Move the window to the top of the stack
root.lower() # Move the window to the bottom of the stack
Conclusion
In this tutorial, you’ve learned how to manipulate various attributes of a Tkinter window in Python, including changing the title, resizing the window, setting transparency, and customizing the icon.
These tips will help you build more user-friendly GUI applications.
For more Python and Tkinter tips, keep exploring and practicing!
Happy coding! 🐍
Tkinter Window in Python Tkinter Window in Python Tkinter Window in Python