Hello, Pythonistas🙋‍♀️ welcome back. Today, we are going to see👀 How to ask the user a question in Python.
Contents
How To Ask The User A Question In Python?
You can make python ask questions and take input using the input function. Super easy right?
Example:-
name = input()
print(name)
Output:-
your name
your name
**The default datatype of whatever you input using the input function will be a string.
Here’s how you How to ask the user a question in Python.
But, how will you get to know that python is asking you your name if you haven’t written this code?
The program’s user won’t read the program, he/she will just use it like you use your applications and games.
User Prompt
To solve this you can enter a prompt string.
Like in the following example:-
name = input("What is your name? ")
print(name)
Output:-
What is your name? your name
your name
The prompt string is nothing but a string you put inside the input function to be displayed to the user to let him/her know what the program is asking for.
How can we take multi-line user input In Python?
We can do this by using the split function.
You would have already done this if you would have solved the previous post’s challenge.
Let’s see this with an example.
names= input("What's your name?, What's your friend's name? ")
name1, name2 = names.split(",")
print(name1, name2)
Output:-
What's your name?, What's your friend's name? Me, My Friend
Me My Friend
Here, the split function separates the elements of the string by commas(Me
is one element and My Friend
is another).
And in the same line, we store both these elements into different variables using multiple assignment.
You can take any number of inputs using this.
There is another method for multiple inputs but for that, you will need to know about loops so that method is not explained here.
How can we take user input in other data types?
The default data type of whatever you input is always a string.
But what if you are making a calculator you can’t use strings for it?
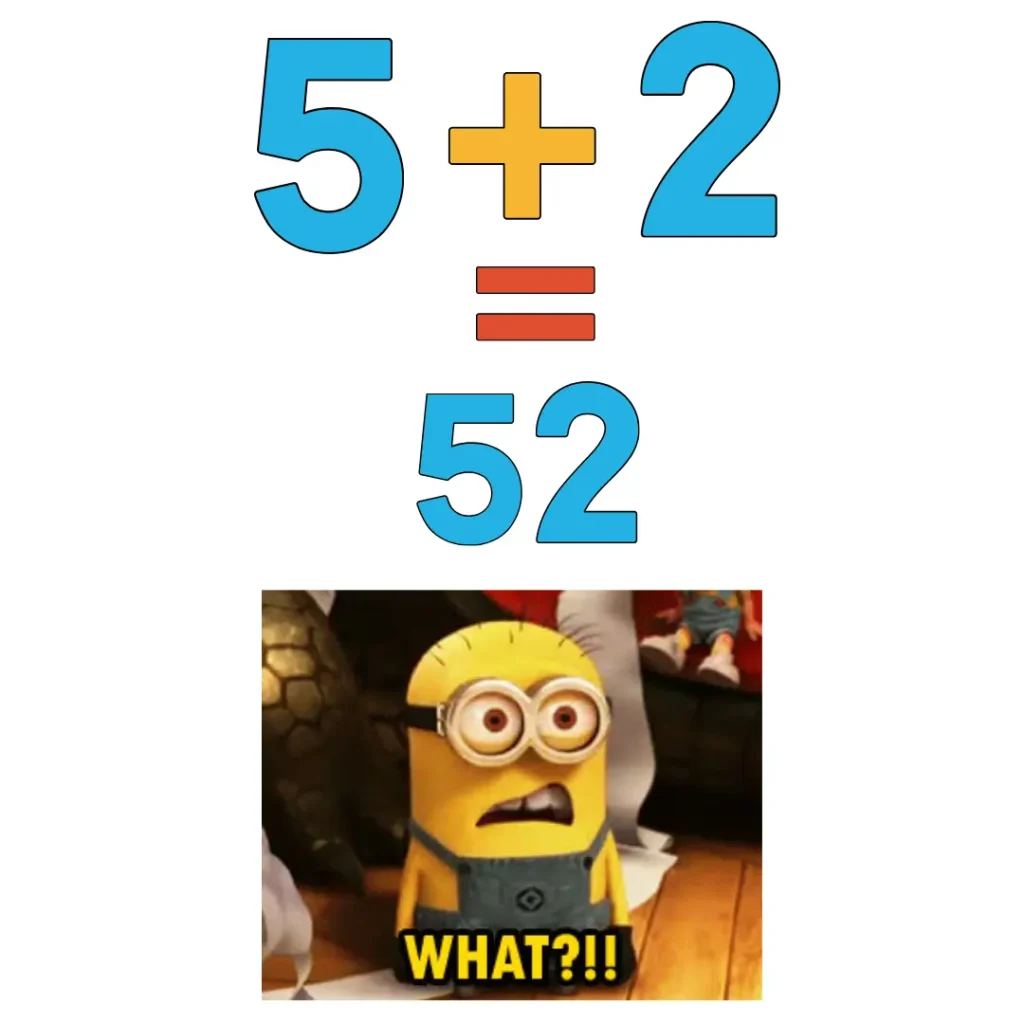
You will need to use integers or floats.
For this, you can use functions like int, float, list, etc. See the example to take an integer as an input.
n = int(input("Enter a number:- "))
print(n)
print(type(n))
Output:-
Enter a number:- 10
10
<class 'int'>
You can also write it this way:-
n = input("Enter a number:- ")
n = int(n)
print(n)
print(type(n))
Output:-
Enter a number:- 10
10
<class 'int'>
How To Ask The User A Question In Python
Here, I’ve read some really great content. It’s definitely worth bookmarking for future visits. I’m curious about the amount of work you put into creating such a top-notch educational website.
Thank you! Stay Tuned!!