Hello Pythonistas, welcome back. Today we will create a password authentication functionality using Python.
This is not a typical project that would show up some results.
This is the one that would be useful in various other projects in the future.
Contents
Library For Hasing
First, let’s get the modules for hashing and other needs.:
import hashlib, os
hashlib: A module that provides hashing functions.
hashlib
is a Python module that provides functions to create secure hashes.
Think of a hash as a unique digital fingerprint for data.
When you put some data (like a password) into a hashing function, it gives you a unique string of characters (the hash) that represents that data.
os: A module that provides functions for interacting with the operating system, such as generating random numbers.
Hashing Function
Now, let’s create a method to hash the password.
This is important to keep the password safe from hackers.
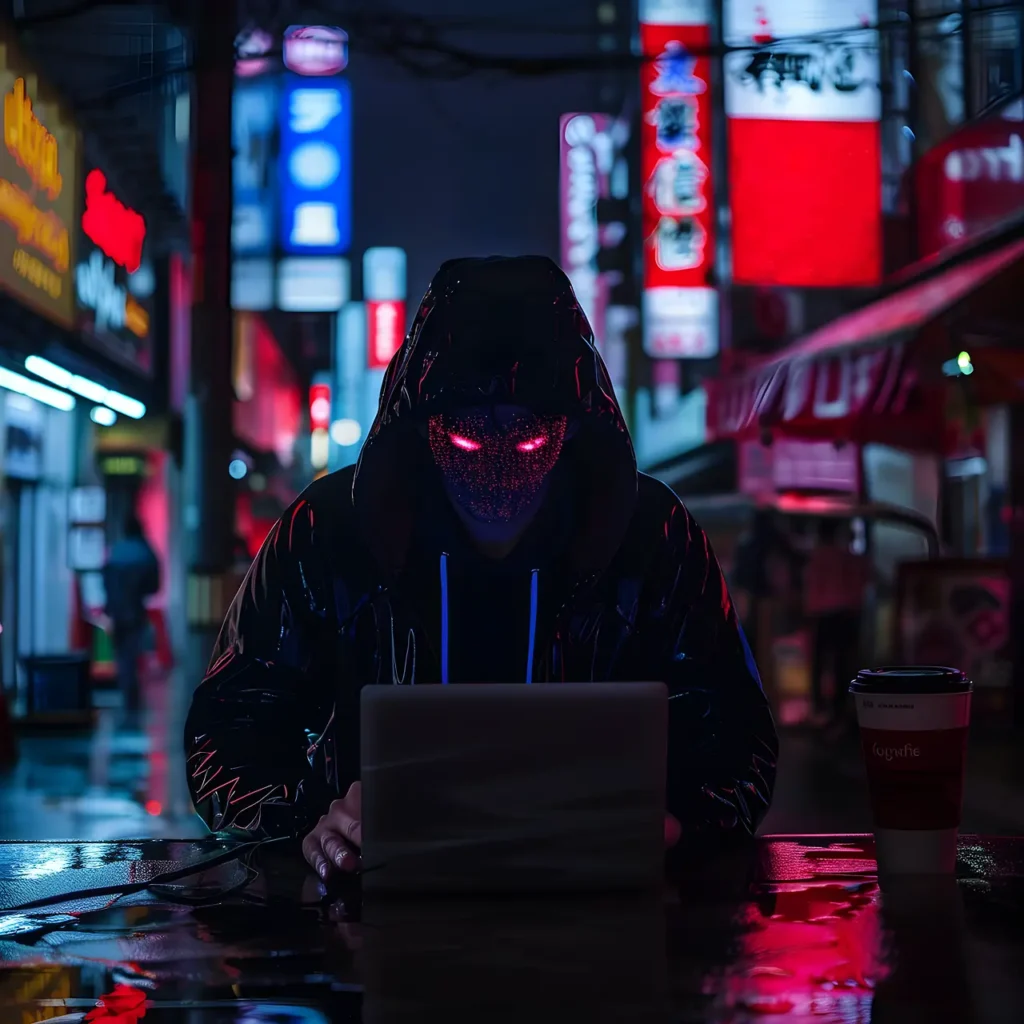
def hash_psd(psd: str) -> str:
salt = os.urandom(16)
hashed_psd = hashlib.pbkdf2_hmac('sha256', psd.encode(), salt, 100000)
return salt.hex() + hashed_psd.hex()
- def hash_psd(psd: str) -> str: Defines a function named
hash_psd
that takes a password (psd) as input and returns a string. - salt = os.urandom(16): Generates a 16-byte random value called “salt” to make the hash more secure.
- hashed_psd = hashlib.pbkdf2_hmac(‘sha256’, psd.encode(), salt, 100000): Uses the PBKDF2 HMAC (Password-Based Key Derivation Function 2) with the SHA-256 hash algorithm to create a hashed version of the password.
- The password is encoded to bytes, and the salt and 100,000 iterations are used to strengthen the hash.
- return salt.hex() + hashed_psd.hex(): Converts the salt and hashed password to hexadecimal strings and concatenates them. This combined string is returned as the hashed password.
Verification Function
Next, let’s create a method for verifying if the provided password is correct or not.
def verify_psd(stored_psd: str, provided_psd: str) -> bool:
salt = bytes.fromhex(stored_psd[:32])
stored_hash = stored_psd[32:]
hashed_psd = hashlib.pbkdf2_hmac('sha256', provided_psd.encode(), salt, 100000)
return hashed_psd.hex() == stored_hash
- def verify_psd(stored_psd: str, provided_psd: str) -> bool: Defines a function named
verify_psd
that takes a stored password hash and a provided password as input and returns a boolean value indicating if they match. - salt = bytes.fromhex(stored_psd[:32]): Extracts the salt from the stored password hash by taking the first 32 characters and converting them back from hexadecimal to bytes.
- stored_hash = stored_psd[32:]: Extracts the actually stored hash from the stored password hash by taking the characters from the 33rd position onward.
- hashed_psd = hashlib.pbkdf2_hmac(‘sha256’, provided_psd.encode(), salt, 100000): Hashes the provided password using the extracted salt and the same PBKDF2 HMAC algorithm with 100,000 iterations.
- return hashed_psd.hex() == stored_hash: Converts the hashed version of the provided password to a hexadecimal string and compares it to the stored hash. Returns
True
if they match,False
otherwise.
Main Block
Finally, let’s test both our methods.
if __name__ == "__main__":
psd_to_store = input("Enter a Password: ")
stored_psd = hash_psd(psd_to_store)
print(f'Stored Password: {stored_psd}')
psd_attempt = 'python-hub'
is_valid = verify_psd(stored_psd, psd_attempt)
print(f'Password is valid: {is_valid}')
- if name == “main”: This line checks if the script is being run directly (not imported as a module).
- psd_to_store = input(“Enter a Password: “): Prompts the user to enter a password and stores it in the variable
psd_to_store
. - stored_psd = hash_psd(psd_to_store): Calls the
hash_psd
function to hash the entered password and stores the result instored_psd
. - print(f’Stored Password: {stored_psd}’): Prints the stored hashed password.
- psd_attempt = ‘python-hub’: Sets the
psd_attempt
variable to ‘python-hub’ as the password attempt. - is_valid = verify_psd(stored_psd, psd_attempt): Calls the
verify_psd
function to check if the password attempt matches the stored hash and stores the result inis_valid
. - print(f’Password is valid: {is_valid}’): Prints whether the password attempt is valid or not.
Full Source Code: Password Authentication Functionality using Python.
import hashlib, os
def hash_psd(psd: str) -> str:
salt = os.urandom(16)
hashed_psd = hashlib.pbkdf2_hmac('sha256', psd.encode(), salt, 100000)
return salt.hex() + hashed_psd.hex()
def verify_psd(stored_psd: str, provided_psd: str) -> bool:
salt = bytes.fromhex(stored_psd[:32])
stored_hash = stored_psd[32:]
hashed_psd = hashlib.pbkdf2_hmac('sha256', provided_psd.encode(),salt,100000)
return hashed_psd.hex() == stored_hash
if __name__ == "__main__":
psd_to_store = input("Enter a Password: ")
stored_psd = hash_psd(psd_to_store)
print(f'Stored Password: {stored_psd}')
psd_attempt = 'python-hub'
is_valid = verify_psd(stored_psd, psd_attempt)
print(f'Password is valid: {is_valid}')
password authentication functionality using Python. password authentication functionality using Python. password authentication functionality using Python. password authentication functionality using Python.