Almost every programmer has first written this code. Printing hello world.
We will write this program @ 2 places. VS Code and Python’s IDLE.
Contents
Creating a new Python project
First, create a new directory/folder called helloworld (or any other name) anywhere in your system e.g., C:\ drive.
Second, open the VS Code and open the helloworld directory.
Third, create a new app.py file, enter the following code, and save it, then press the run button:
print('Hello World!')
#o/p:
#Hello World
The print()
is a built-in function that displays a message on the screen.
Note: if the run button is not there then install the code runner extension. Go to the extensions tab, type the name, and install it.
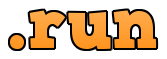
What is a function?
A function is one-word code that executes multiple lines of code for you. For example, you use the print() function:
print("Hello World!")
This single-word print() is a function. It takes an argument (i.e., some input). Executes some lines. Then, as a result, it prints the message as output.
input -> function() -> output
Whether you do a sum of two numbers, that’s a function. Or multiply two numbers, that’s also a function. In general, a function takes your inputs, applies some rules, and returns a result.
Python has many built-in functions like the print()
function to use them out of the box in your program.
In addition, Python allows you to define your functions, which you’ll learn how to do it later.
Executing the Code Using Terminal
To execute the app.py file, you first launch the Command Prompt on Windows or Terminal on macOS or Linux.
Then, navigate to the helloworld directory.
After that, type the following command to execute the app.py file:
python app.py
If you use macOS or Linux, you use python3
command instead:
python3 app.py
If everything is fine, you’ll see the following message on the screen:
Hello World!
If you use VS Code, you can also launch the Terminal within it by:
- Accessing the menu Terminal > New Terminal
- Or using the keyboard shortcut
Ctrl+Shift+`
.
Typically, the backtick key (`
) located under the Esc
key on the keyboard.
Python IDLE
The Python IDLE (Integrated Development and Learning Environment) comes with the Python distribution by default.
The Python IDLE is also known as an interactive interpreter.
In short, the Python IDLE helps you experiment with Python quickly in a trial-and-error manner.
Here’s how you launch the IDLE
First, launch the Python IDLE program:
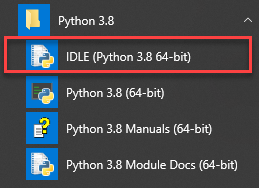
A new Python Shell window will display as follows:
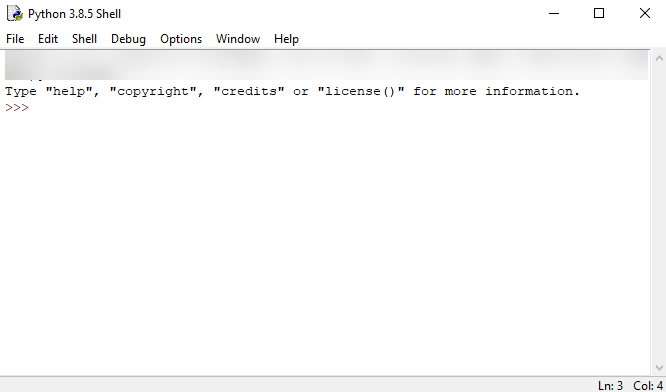
Now, you can enter the Python code after the cursor >>>
and press Enter
to execute it.
For example, you can type the previous print('Hello, World!')
and press Enter
, you’ll see the message Hello, World!
immediately on the screen:
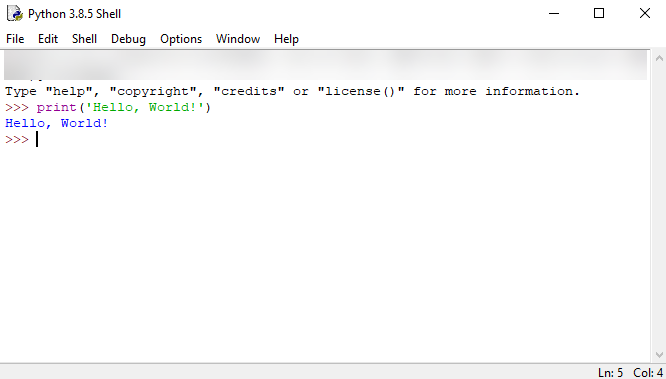
Conclusion
- 3 Ways to execute the Python program.
- Code Runner
- Terminal
- Python IDLE
- Printed Hello World!
- Understood What is a function.