Hello, Pythonistas Welcome Back. Today we will see how to make a fully functional modern combobox in CustomTkinter.
We will use the CTkComboBox Widget.
Contents
How Does CTkComboBox Look?
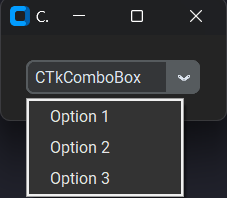
Basic Code
This is how you can make a simple ComboBox in CustomTkinter (or CTk).
from customtkinter import CTk, CTkComboBox
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("CTkComboBox Example")
# Create a CTkComboBox
self.combo_box = CTkComboBox(self)
self.combo_box.pack(padx=20, pady=20)
# Adding values
self.combo_box.configure(values=["Option 1", "Option 2", "Option 3"])
# Connecting it to a callback function to print the selected option on click event
self.combo_box.configure(command=self.combobox_callback)
def combobox_callback(self,choice):
print("combobox dropdown clicked:", choice)
app = App()
app.mainloop()
Like any other widget in CTk, it is first created, and then it is pushed to the window.
Instead of providing just one value a list of values is provided.
As it is like a button you can give it a command function to shoot when you select a particular option
It takes a compulsory argument master. This will specify where it will stay.
A Sample Modern ComboBox
We will see how we can make a simple dropdown that will allow you to change the appearance mode of the CTk app.
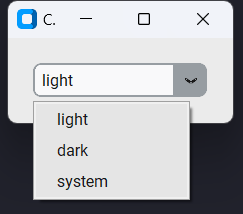
All we would need to do is:
- Set default appearance mode
- Create a ComboBox with values
- light
- dark
- system
- Define a function that changes the appearance mode based on the choice.
That’s it!
from customtkinter import CTk, CTkComboBox, set_appearance_mode
class App(CTk):
# Defaulting to light mode
set_appearance_mode("light")
def __init__(self):
super().__init__()
self.title('Change appearance')
# creating combobox
self.combo_box = CTkComboBox(self, values=["light", "dark", "system"], command=self.change_mode)
self.combo_box.pack(padx=20, pady=20)
def change_mode(self,choice):
set_appearance_mode(choice)
app = App()
app.mainloop()
All Configurations
argument | value |
---|---|
master | root, frame, top-level |
command | function will be called when the dropdown is clicked manually |
values | list of strings with values that appear in the dropdown menu |
state | border-width in px |
width | box width in px |
height | box height in px |
corner_radius | corner radius in px |
border_width | border width in px |
fg_color | foreground (inside) color, tuple: (light_color, dark_color) or single color |
border_color | border color, tuple: (light_color, dark_color) or single color |
button_color | right button color, tuple: (light_color, dark_color) or single color |
button_hover_color | hover color of the button, tuple: (light_color, dark_color) or single color |
dropdown_fg_color | dropdown fg color, tuple: (light_color, dark_color) or single color |
dropdown_hover_color | dropdown button hover color, tuple: (light_color, dark_color) or single color |
dropdown_text_color | dropdown text color, tuple: (light_color, dark_color) or single color |
text_color | text color, tuple: (light_color, dark_color) or single color |
text_color_disabled | text color when disabled, tuple: (light_color, dark_color) or single color |
font | button text font, tuple: (font_name, size) |
dropdown_font | button text font, tuple: (font_name, size) |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.