Hello Pythonistas, welcome back. Today we will see how to use the CTkFrame widget in customtkinter.
A CTkFrame is a widget that displays as a simple rectangle. Typically, you use a frame to organize other widgets both visually and at the coding level.
Contents
How Does A Frame Look?
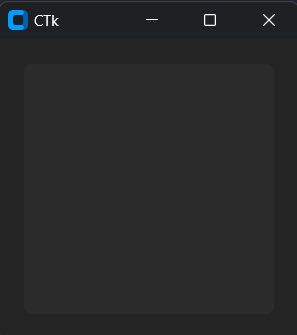
What Is A CTkFrame Used For?
Have you ever worked with some real and big applications? You might have observed that they have some partitions🖽 in them.
I mean the editing menu on one side and the buttons on the other.
Say for example the paint app or blender(a 3d editing software).
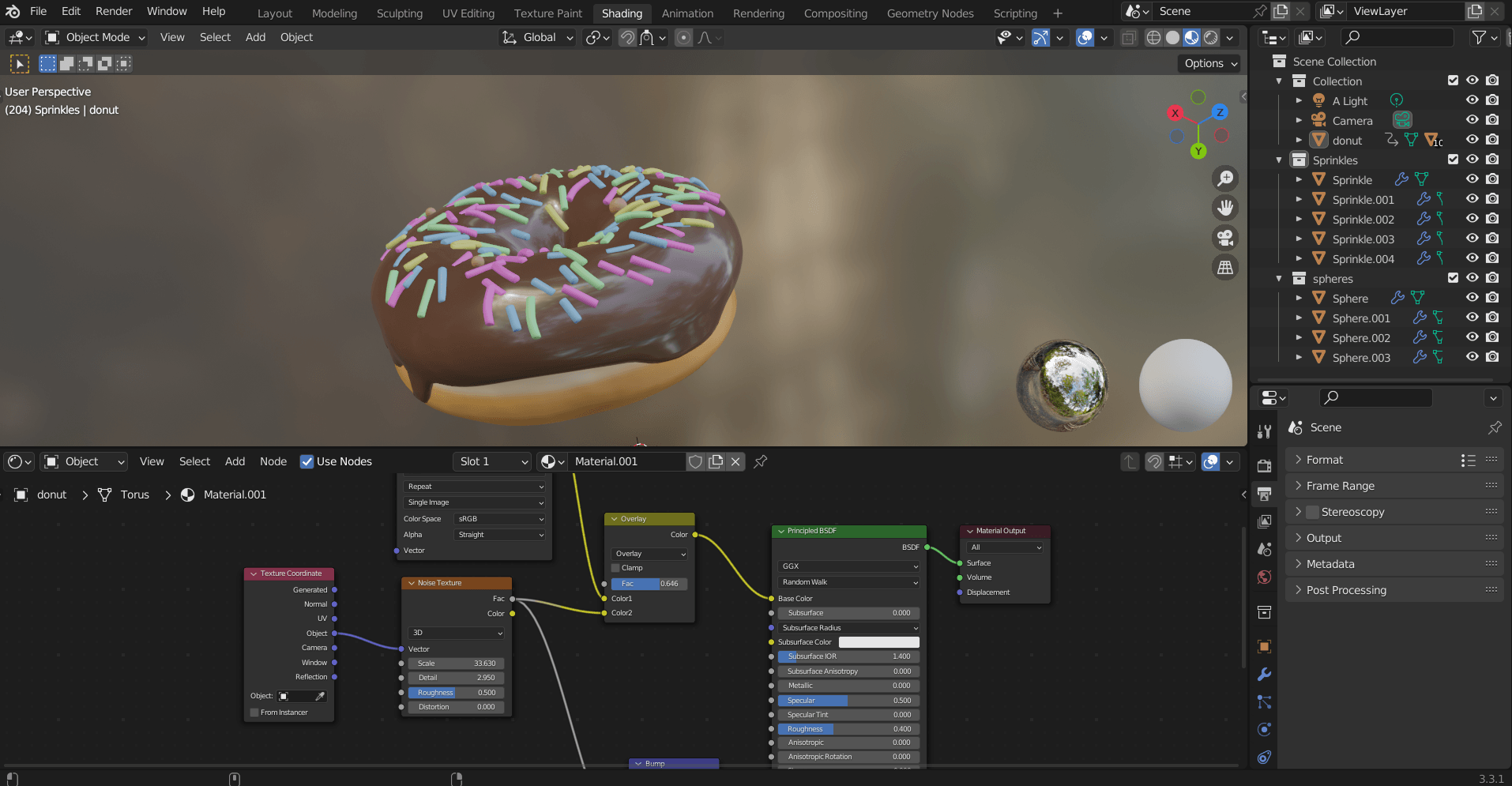
To add such partitions in customtkinter we need to use the frames widget.
Basic Code
There are two ways you can use frames. One is directly using them like you use other widgets.
For example:
from customtkinter import CTk, CTkFrame
class App(CTk):
def __init__(self):
super().__init__()
self.frame = CTkFrame(self)
self.frame.pack(padx=20, pady=20)
app = App()
app.mainloop()
You can add widgets to the frame. It can act as master
for any other widget including another frame.
Another way is making a frame. You can customize a frame and reuse it for multiple apps. You can do that using class.
For example:
from customtkinter import CTk, CTkFrame, CTkLabel, CTkButton
class MyFrame(CTkFrame):
def __init__(self, master):
super().__init__(master)
# add widgets onto the frame, for example:
self.label = CTkLabel(self)
self.label.grid(row=0, column=0, padx=20, pady=20)
self.b = CTkButton(self)
self.b.grid(row=1, column=0, padx=20, pady=20)
You can use it just as a normal frame. This frame has a label and a button(which does nothing😅)
class App(CTk):
def __init__(self):
super().__init__()
self.frame = MyFrame(self)
self.frame.pack(padx=20, pady=20)
app = App()
app.mainloop()
Output:-
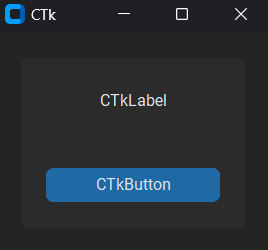
All Configurations
Argument | Value |
---|---|
master | root, Frame or Toplevel |
width | width in px |
height | height in px |
border_width | width of border in px |
fg_color | foreground color, tuple: (light_color, dark_color) or single color or “transparent” |
border_color | border color, tuple: (light_color, dark_color) or single color |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.