Hello, Pythonistas Welcome Back to python-hub.com. Today we will see how to make a fully functional modern input dialog in CustomTkinter.
We will use the CTkInputDialog Widget.
Contents
How Does CTkInputDialog Look?
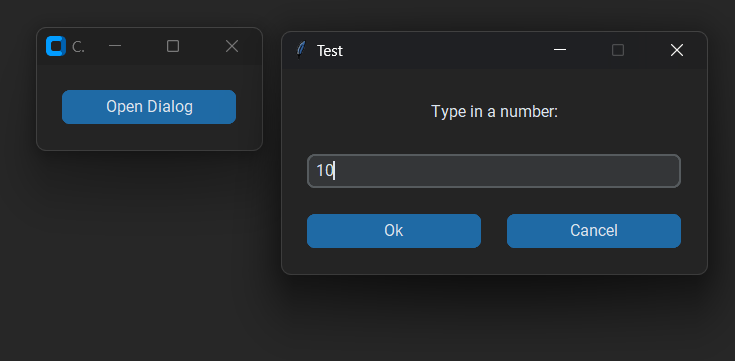
Basic Code
This is how you can make an input dialog in CustomTkinter (or CTk).
from customtkinter import CTk, CTkInputDialog, CTkButton, CTkLabel
class App(CTk):
def __init__(self):
super().__init__()
button = CTkButton(self, text="Open Dialog", command=self.button_click_event)
button.grid(row=0, column=0, padx=20, pady=20)
def button_click_event(self):
dialog = CTkInputDialog(text="Type in a number:", title="Test")
label = CTkLabel(self, text=f"User entered: {dialog.get_input()}")
label.grid(row=1, column=0)
if __name__ == "__main__":
app = App()
app.mainloop()
It is not like any other widget which is first created and then packed on the window. Why?
Well, because it is not to be there on the window. It is a free window in itself. As you can see in the image.
Its functionality resembles the CTkEntry widget.
You can directly use the input widget but it’s better to do it with a button to make the feel of the GUI hassle-free and stable.
So, we first created a button and connected it to a function. Inside the function, I have created an input dialog that pops up as soon as the button is clicked.
The get_input()
method gets us the value that the user provided in the dialog box.
get_input()
: It returns input and waits for the ‘Ok’ or ‘Cancel’ button to be pressed.
That’s it!
Usually, you should use an entry widget but at times this can be useful too.
Cause you know everything has its own space in programming.
All Configurations
argument | value |
---|---|
title | string for the dialog title |
text | text for the dialog itself |
fg_color | window color, tuple: (light_color, dark_color) or single color |
button_fg_color | color of buttons, tuple: (light_color, dark_color) or single color |
button_hover_color | hover color of buttons, tuple: (light_color, dark_color) or single color |
button_text_color | text color of buttons, tuple: (light_color, dark_color) or single color |
entry_fg_color | color of entry, tuple: (light_color, dark_color) or single color |
entry_border_color | border color of entry, tuple: (light_color, dark_color) or single color |
entry_text_color | text color of entry, tuple: (light_color, dark_color) or single color |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.