Hello, Pythonistas Welcome Back. Today we will see how to make a modern OptionMenu in CustomTkinter.
We will use the CTkOptionMenu Widget.
Contents
How Does CTkOptionMenu Look?
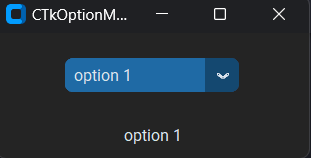
Basic Code
This is how you can make a simple OptionMenu in CustomTkinter (or CTk).
# Make necessary imports
from customtkinter import CTk, CTkOptionMenu, CTkLabel
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("CTkOptionMenu Example")
self.geometry("250x100")
# Create an optionmenu
optionmenu = CTkOptionMenu(self, values=["option 1", "option 2"],
command=self.optionmenu_callback)
optionmenu.pack(padx=20, pady=20)
# This function creates a label that displays current
# selected value in the option menu
def optionmenu_callback(self, choice):
self.label = CTkLabel(self, text=choice)
self.label.pack()
app = App()
app.mainloop()
Like any other widget in CTk, it is first created and then it is pushed to the window.
It takes a compulsory argument master. This will specify where it will stay.
Values argument holds the list of values that you want to give in the menu.
As it is like a button you can give it a command function to shoot when you select a particular option.
We have made an OptionMenu that has two options. Whenever you select an option it displays on the window.
A Sample Modern Option Menu Widget
We will see how you can make an option menu that allows you to enter phone no. based on your country.
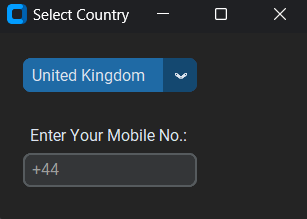
All we would need to do is:
- Create a dictionary with country names and phone codes.
- Create an OptionMenu that has a list of country names.
- Now, create a function that creates a label “Enter Your Mobile No.: ” and then create an entry widget that has a placeholder of the corresponding phone code.
- Connect this function to the OptionMenu created in step 2.
That’s it!
Complete Code(For Complete Code Click Here):
# Make necessary imports
from customtkinter import CTk, CTkOptionMenu, CTkEntry, CTkLabel
country_phone = {
"Australia": 61,
"India": 91,
"United States": 1,
"United Kingdom": 44
}
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("Select Country")
self.geometry("250x150")
# Create an optionmenu
optionmenu = CTkOptionMenu(self, values=list(country_phone.keys()),
command=self.optionmenu_callback)
optionmenu.grid(row=0, column=0, padx=20, pady=20)
# This function creates a label that displays current
# selected value in the option menu
def optionmenu_callback(self, choice):
self.label = CTkLabel(self, text="Enter Your Mobile No.: ")
self.label.grid(row=1, column=0)
self.entry = CTkEntry(self, placeholder_text=f"+{country_phone[choice]}")
self.entry.grid(row=2, column=0)
app = App()
app.mainloop()
Note: we will not put any checks on the entered number. It’s just to demonstrate OptionMenu.
All Configurations
argument | value |
---|---|
master | root, frame, top-level |
width | box width in px |
height | box height in px |
fg_color | foreground (inside) color, tuple: (light_color, dark_color) or single color |
text_color | text color, tuple: (light_color, dark_color) or single color |
hover | enable/disable hover effect: True, False |
state | “normal” (standard) or “disabled” (not clickable, darker color) |
command | function will be called when the dropdown is clicked manually |
variable | StringVar to control or get the current text |
values | list of strings with values that appear in the option menu dropdown |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.
Common Questions
When to use Variable classes? (BooleanVar, DoubleVar, IntVar, StringVar)
We mostly do not use these variables. However, if you want to know more about it you can read this StackOverflow answer.
What is the difference between OtpionMenu and ComboBox in Customtkinter?
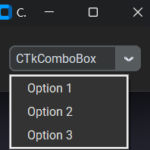
If configured adequately combobox allows users to enter their options in addition to the existing ones.
You can read more about it in this StackOverflow answer.