Hello, Pythonistas Welcome Back. Today we will see how to make a fully functional modern Progress Bar in CustomTkinter.
We will use the CTkProgressBar Widget.
Contents
How Does CTkProgressBar Look?
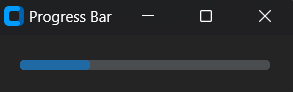
Basic Code
This is how you can make a simple progress bar in CustomTkinter (or CTk) using CTkProgressBar.
# Make necessary imports
from customtkinter import CTk, CTkProgressBar
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("Progress Bar")
# Create a Progress Bar
self.progress = CTkProgressBar(self)
self.progress.pack(padx=20, pady=20)
self.progress.set(0.25)
app = App()
app.mainloop()
Like any other widget in CTk, a button is first created and then it is pushed to the window.
It takes a compulsory argument master. This will specify where the CTkProgressBar will stay.
You can do many things with a CTkProgressBar. I recommend trying them out on your own. If you encounter any issues, let me know in the comment section.
A Sample Progress Bar
We will make a weather predictor that doesn’t predict weather.
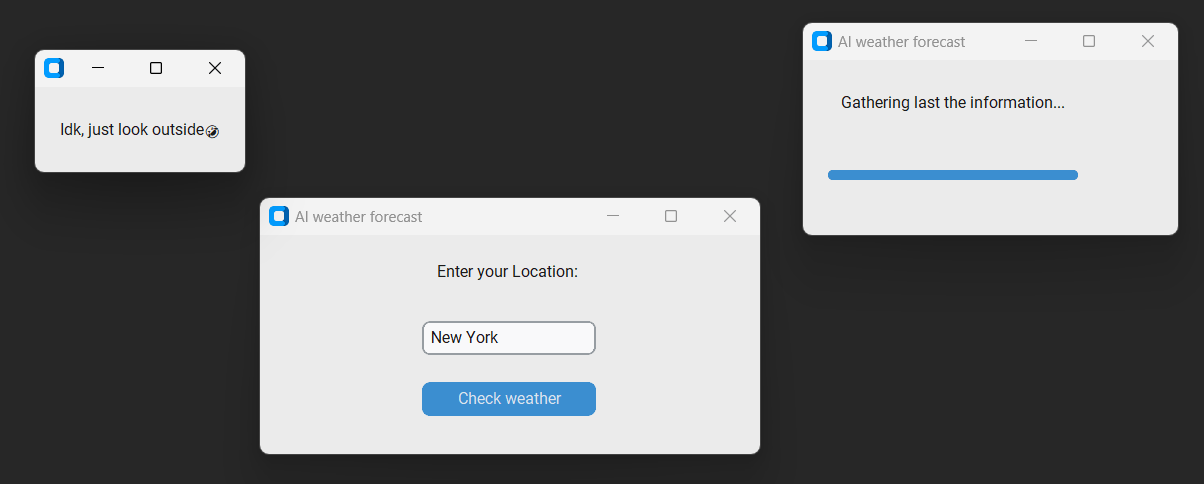
Yes, it will take up the city’s name.
Then, it will run a progress bar as if it were fetching current weather. Then, it will show an “I don’t know look out for yourself” message.
We will need to create 3 windows:
- One will display an entry widget and a button that will ask for location.
- Then on clicking the button, another window will open that will have a progress bar faking search.
- Finally, this will open another window that will display “I don’t know look out for yourself”.
That’s it!
Complete Source Code (Click Here For Complete Source Code):
from customtkinter import *
class Fooled(CTk):
"""A custom Tkinter Toplevel window to display a text label and a GIF image of Jerry from the 'Tom and Jerry' cartoon."""
def __init__(self):
"""Initialize the Fooled Toplevel window."""
super().__init__()
self.title("")
set_appearance_mode("light")
self.label = CTkLabel(self, text="Idk, just look outside🤣")
self.label.pack(padx=20, pady=20)
class ShowWhether(CTk):
"""A custom Tkinter Toplevel window that simulates a weather forecast process with text labels and a progress bar."""
def __init__(self):
"""Initialize the ShowWhether Toplevel window."""
super().__init__()
self.title("AI weather forecast")
set_appearance_mode("light")
self.geometry("300x140")
self.label = CTkLabel(self, text="Searching Location...")
self.label.grid(row=0, padx=20, pady=20)
self.label.after(1000, self.on_after)
self.pb = CTkProgressBar(self, orientation="horizontal", mode="determinate")
self.pb.grid(row=1, padx=20, pady=20)
self.pb.set(0)
def on_after(self):
"""Update the label text and progress bar after a delay."""
self.label = CTkLabel(self, text="Analyzing the clouds...")
self.label.grid(row=0, padx=20, pady=20)
self.label.after(1000, self.on_after1)
self.pb.set(0.25)
def on_after1(self):
"""Update the label text and progress bar after a delay."""
self.label = CTkLabel(self, text="Looking outside your window...")
self.label.grid(row=0, padx=20, pady=20)
self.label.after(1000, self.on_after2)
self.pb.set(0.50)
def on_after2(self):
"""Update the label text and progress bar after a delay."""
self.label = CTkLabel(self, text="Gathering last the information...")
self.label.grid(row=0, padx=20, pady=20)
self.label.after(1000, self.last)
self.pb.set(0.75)
def last(self):
"""Finalize the weather forecast process and display the Fooled Toplevel window."""
self.pb.set(1)
show = Fooled()
show.mainloop()
class Get_City(CTk):
"""A custom Tkinter main application class to get the user's location and initiate the weather forecast."""
def __init__(self):
"""Initialize the Get_City application."""
super().__init__()
set_appearance_mode("light")
self.title("AI weather forecast")
self.geometry("400x175")
self.label = CTkLabel(self, text="Enter your Location: ")
self.label.pack(padx=20, pady=15)
self.city = CTkEntry(self, placeholder_text="Enter Your city ")
self.city.pack(padx=20, pady=10)
self.check_weather = CTkButton(self, text="Check weather", command=self.weather)
self.check_weather.pack(padx=20, pady=10)
def weather(self):
"""Check if the city input is valid and initiate the weather forecast process."""
if self.city.get() == "":
print("Enter a city First")
else:
show = ShowWhether()
show.mainloop()
if __name__ == "__main__":
app = Get_City()
app.mainloop()
All Configurations
argument | value |
---|---|
master | root, tkinter.Frame or CTkFrame |
width | slider width in px |
height | slider height in px |
border_width | border width in px |
corner_radius | corner_radius in px |
fg_color | foreground color, tuple: (light_color, dark_color) or single color |
border_color | slider border color, tuple: (light_color, dark_color) or single color |
progress_color | progress color, tuple: (light_color, dark_color) or single color |
orientation | “horizontal” (default) or “vertical” |
mode | “determinate” for linear progress (default), “indeterminate” for unknown progress |
determinate_speed | speed for automatic progress in determinate mode started by .start(), default is 1 |
indeterminate_speed | speed for automatic progress in indeterminate mode started by .start(), default is 1 |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.
Just want to say your article is as amazing. The clarity in your post is simply spectacular and i can assume you are an expert on this subject. Fine with your permission allow me to grab your feed to keep up to date with forthcoming post. Thanks a million and please carry on the enjoyable work.
Thank you!
Super results your solving everything even it is a small problems good for learning. 🙂
Thank you very much stay tuned for more and keep learning…