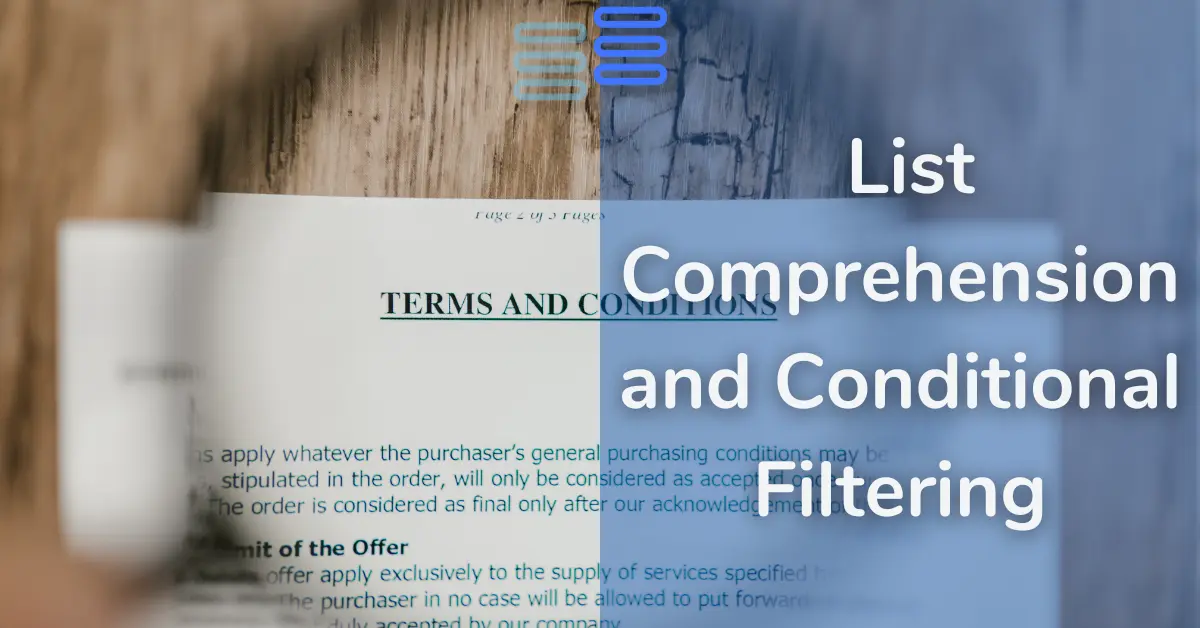
Test your understanding of list comprehension and conditional filtering in Python with this quiz question.
You’ll analyze a function that filters a list of numbers based on a specified target value.
Contents
Question: List Comprehension and Conditional Filtering
What will be the output of the following Python code snippet?
def func(nums, target):
return [i for i in nums if i > target]
nums = [1, 2, 3, 4, 5]
target = 3
print(func(nums, target))
Answer: B
Explanation:
In this quiz question, a Python function named func
is defined to filter a list of numbers (nums
) by keeping only the elements greater than a specified target
. The function uses a list comprehension, which is a concise way to create lists in Python.
Here’s the code breakdown:
def func(nums, target):
return [i for i in nums if i > target]
nums
is the list of numbers to be filtered.target
is the threshold value.- The list comprehension
[i for i in nums if i > target]
iterates over each elementi
innums
and includes it in the new list only if it is greater thantarget
.
When the function is called with nums = [1, 2, 3, 4, 5]
and target = 3
, the list comprehension filters out the numbers that are not greater than 3, resulting in the list [4, 5]
.
The correct answer is B) [4, 5]
.
This question helps to reinforce your understanding of list comprehensions and how they can be used to filter data in Python.