Hello Pythonistas, welcome back. Do you find it hard to remember🤔 the lists that your mom gives you for shopping?
Well, in that case, today is your lucky day, python can remember 🤓 these lists for you and can also easily tell you what are the tasks still left to be completed.
And best of all, this list can store any type of data inside it like numbers, characters, and even lists.
Sounds exciting? Let’s get started.
Contents
Previous post’s challenge’s solution
But, before we get started, let’s first look at the solution to the previous post’s challenge.
Madlib_logo ='''
, ; ,
.' '.
/ () () \\
; ;
; :. MADLIB .; ;
\\'.'-.....-'.'/
'.'.-.-,_.'.'
'( (..-'
'-'
'''
print(Madlib_logo)
#step1: Store story in a variable.
story = '''A teenage boy named John had just passed his driving test
and asked his Dad if he could start using the family car. The Dad
said he'd make a deal with John, "You bring your grades up from a C
to a B average, study your Bible a little, and get your hair cut.
Then we'll talk about the car". John thought about that for a
moment, decided he'd settle for the offer and they agreed on it.
After about six weeks, the Dad said, "John, you've brought your
grades up and I've observed that you have been studying your
Bible, but I'm disappointed you haven't had your hair cut."
John said, "You know, Dad, I've been thinking about that,
and I've noticed in my studies of the Bible that Samson had
long hair, John the Baptist had long hair, Moses had long hair,
and there's even strong evidence that Jesus had long hair." His
Dad replied,"Did you also notice that they all walked everywhere
they went?"'''
#step2: Input variables.
name = input("Enter a name:- ")
skill = input("Enter a skill:- ")
object_ = input("Enter any object:- ")
verb1 = input("Enter a verb:- ")
verb2 = input("Enter another verb:- ")
verb3 = input("Enter a verb again:- ")
adjective = input("Enter an adjective:- ")
#step3: Merge variable in story using an f-string
changed_story = f'''A {adjective} boy named {name} had just passed his {skill} test
and asked his Dad if he could start using the family {object_}. The Dad
said he'd make a {verb1} with {name}, "You bring your grades up from a C
to a B average, study your Bible a little, and get your hair cut.
Then we'll talk about the {object_}". {name} thought about that for a
moment, decided he'd settle for the offer and they agreed on it.
After about six weeks, the Dad said, "{name}, you've brought your
grades up and I've observed that you have been studying your Bible,
but I'm disappointed you haven't had your hair cut."
{name} said, "You know, Dad, I've been {verb2} about that, and I've
noticed in my studies of the Bible that Samson had long hair,
John the Baptist had long hair, Moses had long hair, and there's even
strong evidence that Jesus had long hair." His Dad replied,
"Did you also notice that they all {verb3} everywhere they went?"'''
#step4: print both the stories
print(f"\n\n-----------Original Story-----------\n{story}\n\n-----------Madlib Story-----------\n{changed_story}")
Output:-
, ; ,
.' '.
/ () () \
; ;
; :. MADLIB .; ;
\'.'-.....-'.'/
'.'.-.-,_.'.'
'( (..-'
'-'
Enter a name:- Emma
Enter a skill:- acting
Enter any object:- sword
Enter a verb:- crying
Enter another verb:- sleeping
Enter a verb again:- dancing
Enter an adjective:- magical
-----------Original Story-----------
A teenage boy named John had just passed his driving test
and asked his Dad if he could start using the family car. The Dad
said he'd make a deal with John, "You bring your grades up from a C
to a B average, study your Bible a little, and get your hair cut.
Then we'll talk about the car". John thought about that for a
moment, decided he'd settle for the offer and they agreed on it.
After about six weeks, the Dad said, "John, you've brought your
grades up and I've observed that you have been studying your
Bible, but I'm disappointed you haven't had your hair cut."
John said, "You know, Dad, I've been thinking about that,
and I've noticed in my studies of the Bible that Samson had
long hair, John the Baptist had long hair, Moses had long hair,
and there's even strong evidence that Jesus had long hair." His
Dad replied,"Did you also notice that they all walked everywhere
they went?"
-----------Madlib Story-----------
A magical boy named Emma had just passed his acting test
and asked his Dad if he could start using the family sword. The Dad
said he'd make a crying with Emma, "You bring your grades up from a C
to a B average, study your Bible a little, and get your hair cut.
Then we'll talk about the sword". Emma thought about that for a
moment, decided he'd settle for the offer and they agreed on it.
After about six weeks, the Dad said, "Emma, you've brought your
grades up and I've observed that you have been studying your Bible,
but I'm disappointed you haven't had your hair cut."
Emma said, "You know, Dad, I've been sleeping about that, and I've
noticed in my studies of the Bible that Samson had long hair,
John the Baptist had long hair, Moses had long hair, and there's even
strong evidence that Jesus had long hair." His Dad replied,
"Did you also notice that they all dancing everywhere they went?"
- First of all, we created a variable to store our story;
- Then, we created different variables to act as blanks in our Madlib;
- Next, we created a new variable to store the changed story;
- Lastly, we printed both stories.
Now, this isn’t the best way to make a Madlib generator in python. But, yes with all the skills that you have acquired by now, this is the best possible way.
Also, in the solution, you can take any story and can also make any word a blank it’s completely your choice. As I said earlier from now on our solutions might be different.
Okay, it’s time to dive deeper into lists.
What are lists in python?
You know that numbers in python help you with math and finance stuff, and strings help you with stories, essays, and a lot more. A list too simply is a data type in python that will help you with your shopping list, to-do list, bucket list, and a lot more.
A list in python is a data type that stores data in an ordered manner. It is always enclosed inside square brackets [ ]. Here’s an example:-
shopping_list = ["fruits", "veggies", "nuts", "milk"]
print(shopping_list)
Output:-
['fruits', 'veggies', 'nuts', 'milk']
You can also make a list containing numbers(integer, float, complex, boolean) like the one below:-
marks = [98, 95, 97, 96, 89]
print(marks)
Output:-
[98, 95, 97, 96, 89]
You can also create lists having elements of more than one data type like this one👇:-
mixed = [98, "hi", 9.7, "happy", 89+3j]
print(mixed)
Output:-
[98, 'hi', 9.7, 'happy', (89+3j)]
NOTE:- indexes start from 0 in lists just like they did in strings. Python doesn’t view lists the same as we do it views them like this👇
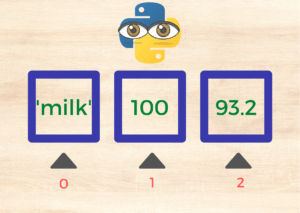
I hope you are clear about what is a list in python.
Let’s how can we access only a few elements of a list.
List Accessing And Slicing
Accessing lists
Accessing a list is the same as accessing a string. It simply means that elements of a list can be individually accessed.
lsit1 = ["milk", 100, 93.2]
print(list1[1])
Output:
100
You can also use negative indexes to access elements. Always remember negative indexes start from-1
, not 0
!!
lsit1 = ["milk", 100, 93.2]
print(list1[-2])
Output:
100
Slicing lists
Slicing a list is the same as slicing a string. It simply means that elements of a list can be accessed in small parts.
list2 = ["milk", 100, 93.2,"hello", "python"]
print(list2[1:4:2])
Output:
[100, 'hello']
First comes the start index number then, comes the stop index number, and lastly comes no. of steps, you want to take.
Here too, you can use negative indexes like this:-
list2 = ["milk", 100, 93.2,"hello", "python"]
print(list2[-2:-5:-2])
Output:
['hello', 100]
NOTE:- Anyone or two index values can also be negative it is in no way necessary to use all negative index numbers.
However, it is not suggested to use the negative values in step as there are chances of errors or unexpected output.
If this seems quite fast and confusing I strongly suggest you read string accessing and slicing. This would help you relate both concepts and would help you understand both of them better. Click here to read them.
Are lists mutable
In one word yes they are. Lists in python are mutable. This means that you can easily change the list once created.
Say for example you are a lazy, smart kid 👶👩💻 just like me, so you made a shopping list and stored it in python.
shopping_list = ["fruits", "veggies", "nuts", "milk"]
Now, you gave your drone that shopping list to give to the shopkeeper 🏬 as you usually do.
But, you added milk to the list instead of mayonnaise. You are not worried about this because lists in python are mutable so you just did this:-👇
#shopping list you created
shopping_list = ["fruits", "veggies", "nuts", "milk"]
print(f"list before:- {shopping_list}")
#changing the list
shopping_list[3]= "mayonnaise" #this simply means that you
#are changing the value of index 3 from milk to mayonnaise
print(f"list after:- {shopping_list}")
Output:-
list before:- ['fruits', 'veggies', 'nuts', 'milk']
list after:- ['fruits', 'veggies', 'nuts', 'mayonnaise']
Functions/Methods On Lists
reverse()
Let’s assume that you want the list that is going to the shopkeeper reversed. Like this:-
shopping_list = ['mayonnaise', 'nuts', 'veggies', 'fruits']
Here’s where the reverse()
function will give you a helping hand and save you from the typing efforts (because you are a lazy, smart kid 👶👩💻 ).
This is how to use this function:-
#shopping list you created
shopping_list = ["fruits", "veggies", "nuts", "mayonnaise"]
print(f"original list:- {shopping_list}")
#reversing the list
shopping_list.reverse()
print(f"reversed list:- {shopping_list}")
Output:-
original list:- ['fruits', 'veggies', 'nuts', 'mayonnaise']
reversed list:- ['mayonnaise', 'nuts', 'veggies', 'fruits']
append()
Now, you suddenly realize that you are not a vegan🥦🍽🥦 and can drink milk 🥛 and you want to add milk to your list.
The append()
function will help you out here.
You just need to type this:-
shopping_list.append("milk")
print(f"list after appending:- {shopping_list}")
Output:-
list after appending:- ['mayonnaise', 'nuts', 'veggies', 'fruits', 'milk']
pop()
Once again a problem you hate milk 🥛 and want to remove ❌ it from your list. (I hope 🤞 you don’t get these many mood swings in real life😜)
But, no need to worry our superhero 🦸♂️ pop()
is here
shopping_list.pop()
print(f"list after pop's attack:- {shopping_list}")
Output:-
list after pop's attack:- ['mayonnaise', 'nuts', 'veggies', 'fruits']
pop()
by default removes the last index but if you want to remove a specific value then write its index number inside the function.
Like, say you have a nut allergy and want to remove nuts. Then just type:-
shopping_list.pop(1)
print(f"list after pop's attack:- {shopping_list}")
Output:-
list after pop's attack:- ['mayonnaise', 'veggies', 'fruits']
insert()
After so many changes in the list, you are feeling that the list has become too short and you won’t get any discount for this small amount of shopping.
So, you decide to add one more item to be able to qualify ☑ the minimum amount after which you can get a discount.
insert()
comes to help you here:-
shopping_list.insert(2, "chocolate")
print(f"list after insert's aid:- {shopping_list}")
Output:-
list after insert's aid:- ['mayonnaise', 'veggies', 'chocolate', 'fruits']
Inside the insert()
function first, you need to put the index number at which you want to insert the value, and then, you need to put a comma, and then, give the value you want to insert.
sort()
You just struck that the shopkeeper always takes the list in a sorted manner(first a, then, b then, c and so on…). To solve this the sort()
function comes into the game.
shopping_list.sort()
print(f"list after sorting:- {shopping_list}")
Output:-
list after sorting:- ['chocolate', 'fruits', 'mayonnaise', 'veggies']
If you want to know more functions on lists read the official documentation.
Conclusion
Here’s what your drone has brought in the shopping basket.
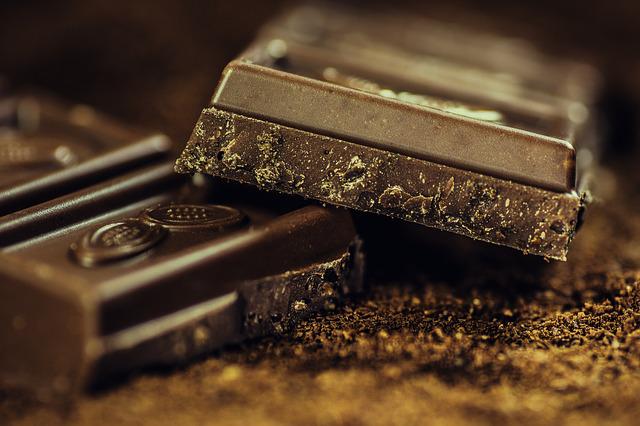
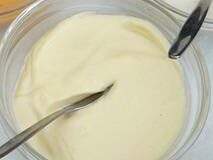
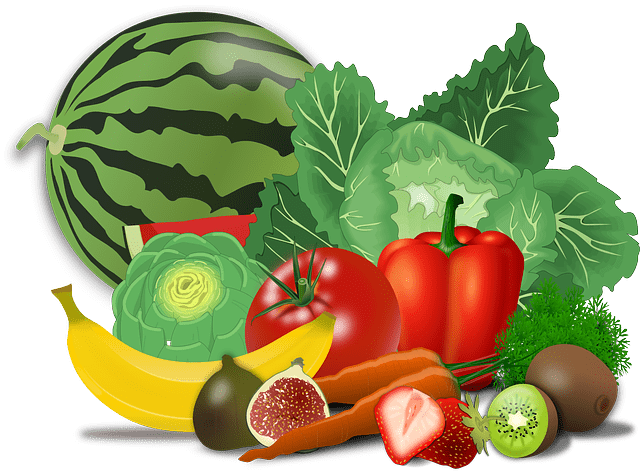
In this post, we covered what is a list, list slicing and accessing, and a few functions on lists. There is a lot of other stuff related to it like nested lists or list comprehension, etc. But, right now you don’t know about loops and if-else statements due to which you won’t be able to understand these concepts. Don’t worry about it, in further posts (very very soon. so stay tuned) we will cover these topics.
Hope you enjoyed reading.🤞 Try solving the challenge below to test the clarity of your concepts.
Challenge🧗♀️
Challenge time.
Your challenge for this post is a continuation of the previous challenge you need to store all the inputs in a list and then are supposed to give them to the changed story variable.
This might sound like a super simple challenge given your skill level but I swear it will slowly develop your logic building.
Also, this challenge makes no sense in reality but that’s alright it’s still gonna help you out with lists.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊Bye bye👋
Hello mate, I just wanted to tell you that this article was actually helpful for me. I was fortunate to take the tips you actually so kindly shared and even put it to use. Your web blog article truly aided me and i also would like to inform your dedicated audience that they really have someone that has her or his thoughts fixed. Thank you once more for the excellent posting. I’ve truly bookmarked this on my favored online bookmarking web site and i also recommend everyone else do the very same.
Glad to know that it helps you. Thanks for your kind appreciation. Stay tuned for more of such content on python programming.