Till now from the first post I have been saying🔉 that you’ll be clear with this or that when you’ll know OOP(object-oriented programming).
Aren’t you freaking😤 out on me?
No need to freak out anymore😓.
Today we will start with the concept of object-oriented programming (OOP) in python.
(You will be making one big project at the end of OOP and not this post.)
Contents
NOTE: I don’t add docstrings to functions when I am explaining them, ’cause you already know what’s happening. But it’s a great practice to add them to your functions.
Before we get ahead here’s the solution to the previous post’s challenge:
Previous post’s challenge’s solution
import time
def string_to_list_words(string):
"""Converts a string to a list of words
used: split & extend functions for this purpose.
input: string
output: list of words"""
string_lines = string.split('\n') #list of sentences.
string_words = [] #list of words.
for sentence in string_lines:
word = sentence.split(" ") # sentence to words.
string_words.extend(word) #adds a list at the end of already there list.
return string_words
def error_counter(original, typed):
"""Returns the total number of errors made.
By comaparing original list to typed list.
input: original list, typed list
output: total number of errors"""
errors = 0
for i in range(len(typed)): #comparing all the elements of the typed words list.
if original[i] == typed[i]:
continue
else:
errors += 1
return errors
def speed_calculator(start_time, end_time, words_typed):
time_taken = end_time - start_time
speed = round(words_typed / time_taken) #rounded the speed to avoid incomprehensible numbers.
return speed
def typing_test():
"""Welcomes the user. Prints out the string to be typed.
Starts timer when you press enter. Takes input till you want to.
Stops time immediately when you finish typing.
The counts errors, speed and returns them."""
print("Welcom to the typing test")
print("You need to type the text below...\n")
text ="""The server backend is written in Python, and provides data storage and processing facilities.
The Graphical User Interface front-end is written in Adobe Flex, and provides aid to the functionality exposed
by the server. It requires a live connection to the server in order to function. A JavaScript 'tracking code'
is installed on client web pages and provides the server with active web analytics. Some of the other technologies
we're using at the moment for WordStream are Adobe Flex, Linux, Apache, and C/C++. As for development process
tools, we're using PyDev Extensions, Trac, Buildbot, Review Board, and Git."""
print(text)
text_words = string_to_list_words(text) #The original text string to list of words.
input("Press ENTER to start typing...")
start = time.time()
typed_text = "" #typed text string.
print()
while True:
typed = input()
if not typed:
break
else:
typed_text += f" {typed}"
end = time.time()
typed_words = string_to_list_words(typed_text) #typed words string to list of words.
typed_words.pop(0) #Removing the first element of the list above as it will be a space.
#due to line 65.
errors = error_counter(text_words, typed_words)
speed = speed_calculator(start, end, len(typed_words))
return f"Your speed is {speed} word(s) per second.\nYou made {errors} mistakes."
print(typing_test())
Output:
Welcome to the typing test
You need to type the text below...
The server backend is written in Python, and provides data storage and processing facilities.
The Graphical User Interface front-end is written in Adobe Flex, and provides aid to the functionality exposed
by the server. It requires a live connection to the server in order to function. A JavaScript 'tracking code'
is installed on client web pages and provides the server with active web analytics. Some of the other technologies
we're using at the moment for WordStream are Adobe Flex, Linux, Apache, and C/C++. As for development process
tools, we're using PyDev Extensions, Trac, Buildbot, Review Board, and Git.
Press ENTER to start typing...
The server backend is written in Python, and provides data storage and processing facilities.
Your speed is 1 word(s) per second.
You made 0 mistakes.
So to make our typing speed tester we divided our program into four functions:
- first, to convert string to list: for easy comparison;
- second, for counting errors: so that the user can know🕵️♀️ the words he/she typed wrong;❌
- third, speed calculator;💨
- fourth, taking the test.👩🏫
We divided our program into functions to enhance the readability🧐 and manageability of the code.
I suggest you read the code clearly line-by-line. This will make clear💡 what is going on in these functions. The solution is well documented.
Still, if you have any doubts🤔 comment in the comment section below.👇
I am not explaining this as, when you become a developer you would need to read and understand others’ code there won’t be any line-by-line explanation. Also, this will help you in learning new technology from its documentation.
Let’s get started with OOP…
what is object-oriented programming?
The way of programming we used to solve the previous post’s challenge is called procedural programming where you divide your code into functions.
Another way of writing your code is: thinking🤔 of everything as an object.📦
But why😕 object?
This is because you write software to solve real-world🌍 problems. And in the real-world🌍 everything is an object. Say for example, if I want to write this blog📝 I need a website📟 object. To operate the website I need a laptop💻 object. And some websites might operate even on a mobile📱 object.
So, object-oriented means ➡directed towards objects. This way of programming makes you think🤔 of everything in form of objects,
OOP has two ✌ main concepts in it:
- Objects, and
- Classes.
Let’s first take at objects in detail.
objects
Every object we know has two major properties:-
- Attributes
- Behavior
Say I as a human object have height and weight, i.e., have some attributes. And I can talk, walk, write, and code, i.e., I have some behaviors.
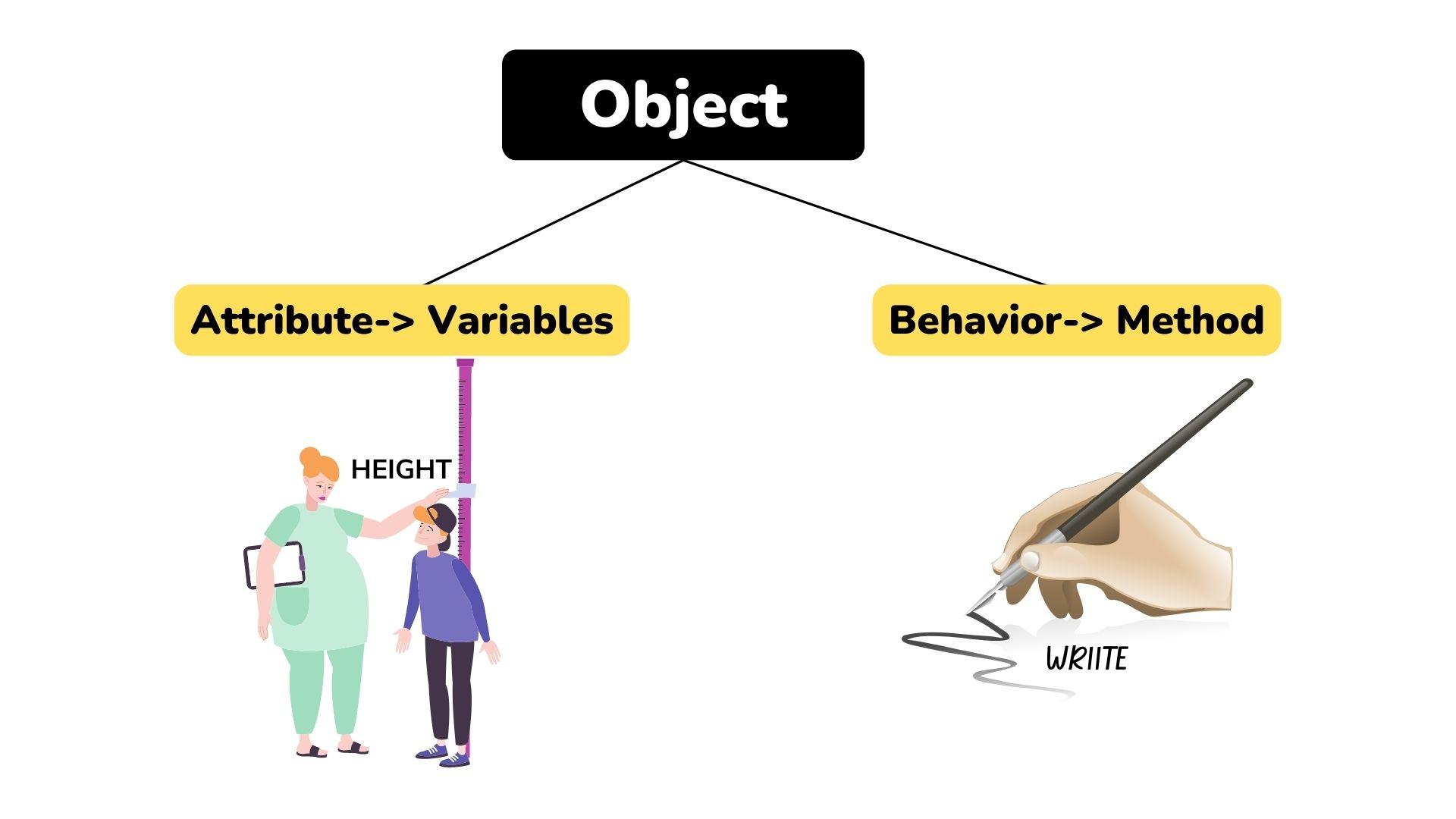
In terms of programming:
- attributes are variables, and
- behaviors are methods. Functions in OOP are called methods.
An instance is a fancy name for an object.
Classes
You must be thinking ok objects are valid✅ they have importance as everything in the real world is an object.
But why❓ classes?
I am sure you use a phone📱, let’s say you use an Apple🍎 iPhone. Now this iPhone is an object for you👩, right? Did you manufacture it at your home🏡? No, it was manufactured in Apple’s factory🏭.
Are you the only one who has this Apple iPhone? Again no, there are hundreds if not thousands of Apple iPhones.
So do you think Apple has a different design for all these iPhones? No, they were made on the same design.
This means that before the phone object was created Apple had a blueprint of how to make it, what features or attributes would it have, etc.
This blueprint based on which an object is created is called a class.
Thus, classes are important as objects are made on the basis of the blueprint created by them.
The flow of a program in OOP:
- So we first create a class and then, give it some:-
- variables,
- methods, and
- instructions on how the object will be created.
- Based on the class we create objects.
All the classes give the objects a blueprint to
- have something, namely variables
- do something, namely methods
- a way to be created, namely constructor. (details in later posts.)
Example:-
my_integer = int("10")
print(type(my_integer))
#Output
#<class 'int'>
Here, my_integer
is a variable that refers to the object 10
. And 10
is an object of a class named int
. You can press ctrl and click on int
if you are in vs code or pycharm and see the code of the class int
.
Here’s a code snippet of the built-in class int
.
class int:
@overload
def __new__(cls: type[Self], __x: str | ReadableBuffer | SupportsInt | SupportsIndex | SupportsTrunc = ...) -> Self: ...
@overload
def __new__(cls: type[Self], __x: str | bytes | bytearray, base: SupportsIndex) -> Self: ...
if sys.version_info >= (3, 8):
def as_integer_ratio(self) -> tuple[int, Literal[1]]: ...
@property
def real(self) -> int: ...
Don’t worry😰 you won’t understand😵 anything more than the fact that int
is a class. That’s it and that’s all you need to understand right now.👍
Why do we need OOP?
Are you wondering🤔 why on the earth🌍 there is a need for object-oriented programming?
That too when we already have procedure programming. It’s quite a simple task to think of everything in functions compared to objects.
But imagine you are writing a code to make a clone of Instagram🌐.
For just getting your user to create an account you’ll need like 10-12 functions. And you’ll need to repeat these 10-12 functions around 1 billion times because that’s the current user count of Instagram.
The functional logic of your code would look like spaghetti🍜.
But if you use object-oriented programming this would look like a clean building🏢 layout.
If you are still in doubt🧐 imagine that you are coding a self-driving car🚗 like Tesla. And remember you would have teams👩🏼🤝👩🏼👩🏼🤝👩🏼 to work on such large projects.
Now the choice is all yours:
- OOP or
- functions.
Creating your own classes and objects
Imagine🤩 that you have started a restaurant🍽. For the restaurant, you have decided to provide an online ordering and booking service.💻📲🧾
For making this up you can use simple procedural programming. It would work fine 👍 as long as you just have 4-5 customers👨👩👧👦 coming into your restaurant.
But what when the no. of customers increases👨👩👧👦👨👩👧👦👨👩👧👦? And what when you open a new branch of that restaurant🏢?
Would the system be practical?
No. It’s not practically possible to manage such a lot of users with procedure programming.
So, we will make a restaurant class
for your online services.💻📱
Our Restaurant class will need, at a minimum,
- A menu,🌭🍕🍔🍿🥞
- Customer’s name,
- Customer’s order, and
- The total bill as its data.💵
Our class must also perform some actions:
- Show menu
- Place order😋
- Pay the bill🤑
Now I know that a restaurant has much more than this but we are starting out so this much is enough for now.
Defining a class
Let’s create a class for your restaurant:
class Restaurant:
menu = {"Pizza": 10, "Waffle": 4, "Coffee": 2.5}
def __init__(self,name,):
self.name = name #string
self.order = [] #A list
self.total_bill = 0 #A float value
def show_menu(self):
print("Here's the menu of our restaurant:-")
for item, price in self.menu.items():
print(f"{item} ${price}")
def place_order(self,order):
print(f"We are Placing Order please wait {self.name}...")
self.order = order.title().split(", ")
print(f"Your is Order Placed successfully {self.name}!")
print(f"Your order is: \n{self.order}")
def pay_bill(self):
if len(self.order)!=0:
for i in self.order:
if i in Restaurant.menu:
self.total_bill+=Restaurant.menu[i]
else:
print(f"Sorry {self.name}, {i} is not available in this restaurant.")
else:
print("You need to Place order first!")
print(f"Your total bill amounts to ${self.total_bill}")
This is how you define a class in python.
- Start with the
class
keyword, followed by the class name in pascal case, and colon. - Here we defined a class named Restaurant.
- This class has 4 attributes:
menu
#it’s a class variable more on that later.name
#its a string to store the customer’s nameorder
#its a list to store customer’s ordertotal_bill
#it’s a float value to store the customer’s total bill amount.
- This class has three(I know 4) methods(functions):
show_menu()
:- Prints the menu with items and prices. It uses for loop for this purpose.place_order()
:- Takes an argument order(a string) and then converts it to the title case and then to a list. Next, the function stores it in theself.order
variable.pay_bill()
:- This method checks whether the customer has placed the order. If he has placed an order, the bill amount is updated using the menu and order variable.
Now, after reading this much you’ll have two questions:
- What is the __init__ method?
- Why have I used the menu variable using the class’s name and the others using the self keyword? And what is this self?
The init
is a special✨ method and so is the self
keyword a special keyword we will take a look at both of them in the next post.
For now, you need to understand that we need to define all the variables related to an object inside the __init__ method.
And for the next question menu is a class variable
and all the others used with a self. before them are instance variables
, and the ones used without anything special are local variables
we’ll look at them in detail in next to next post.
Till then just know that they are different.
In short, an instance of the Restaurant class will
- have a menu, the customer’s name, his order, and bill details
- Also, this class can:
- show customer’s the menu
- take orders from them
- and get payments from them.
don’t be confused by the keyword self
we will look at it in depth in the next post. for now, just understand that it is important while working with methods inside of a class.
A Point to keep in mind🧠: Classes are usually named in “Pascal case”. This means the first letter of each word is capitalized.
Creating an instance(object)
To understand this concept well let’s create an instance of Restaurant class.
cust1 = Restaurant("John") #we need to pass customer's name because of the init method.
cust1 is a variable that stores the instance of the Restaurant class.
To create an instance we simply need to write the class name followed by parenthesis.
And to be able to use that instance/object you need to assign it to a variable.
So, we have finally created our class’s instance. Which means we have an object ready from our blueprint.
Accessing variable in class using instance(object)
Now let’s see whether we can print the customer’s name or not.
To access the menu variable:-
print(cust1.name)
#Output:
#John
To access a variable inside the class you need to give the object followed by a dot and the variable name.
Always remember you should never access any variable of class outside the class. You should define methods to get or set values for them.
Just like we did for the menu variable.
What we did above is not a good practice strictly avoid this.
Accessing Methods in class using instance(object)
Let’s use all our methods one after another.
Starting with show_menu
cause that’s technically the first thing any customer would do.🍕☕🍩🧇
Now let’s see how can you use methods that are inside of a class using instance:
cust1.show_menu()
Output:
Here's the menu of our restaurant:-
Pizza $10
Waffle $4
Coffee $2.5
Here, you are telling the object of cust1
that it needs to access the show_menu()
method.
To use any methods inside of the class using its instance: give the instance followed by "."
, the method name, and parenthesis. And of course, any arguments to the method if required.
Now that the customer has gone through the menu he would like to order something.
Let’s call the place_order()
method for him.
cust1.place_order("coffee, waffle")
Output:
We are Placing Order please wait John...
Your is Order Placed successfully John!
Your order is:
['Coffee', 'Waffle']
Now it’s time for payment.💵
Let’s use the pay_bill()
method:
cust1.pay_bill()
Output:
Your total bill amounts to $6.5
And that’s it your class functions awesome👍😊. It has got variables and methods that function well.
I know that this would seem quite illogical😒 as our class isn’t perfect to cater a large number of customers👨👩👧👦👨👩👧👦👨👩👧👦👨👩👧👦👨👩👧👦.
But this example was to understand the basic concepts of how to make
the class.
We’ll look forward to how to use
them after we finish the basic concepts.
Conclusion
In this post, we saw what is meant by object-oriented programming (OOP).
Next, we saw what is meant by objects and classes in OOP.
After that, we saw what is the need for OOP.
Lastly, we saw how to define your own class by creating the Restaurant class for the online services of your restaurant.
Also, we saw that this just explains how to write the code of a class and not how to use them yet.
OOP is a vast concept we will continue this in the upcoming posts.
Challenge🧗♀️
Your challenge is to create an Account class:
This class should have three variables inside of the __init__ method:
- name
- balance
- password
It should also have three methods:
- withdraw
- deposit
- show balance
Create an instance/object of this class along with that show the use of all the methods with that instance.
Helper template:- (Don’t shy away or feel bad😞 if you need to use it. It’s absolutely normal to be confused😵 with the question.)
class Account:
def __init__(self, name, balance, password):
self.name = name
self.balance = balance
self.password = password
def withdraw(self, amt, password):
"""Check if the password is correct or not.
Check if the amount is positive or not.
Check whether the balance is present in the account or not.
If all checks passed decrease the balance."""
def deposit(self, amt, password):
"""Check if the password is correct or not.
Check if the amount is positive or not.
If all checks passed increase the balance."""
def show_balance(self, password):
"""print name, balance in dollars."""
Solve this challenge and have fun.
There is a strong chance that from now on it would be difficult to understand the logic of the program. To solve this you should make programs in three steps:
- Solve it in simple English,
- Convert this into a flowchart, and
- Then make your code based on the flowchart.
This would increase your speed and boost your logic. Try solving all the previous challenges this way and you would see the difference it has brought in your coding skills.
To make flowcharts you can go to this free website:- click here
For flowchart-making rules:- click here.
In the next post, we will dive deeper into OOP. And till then try to think of every problem in OOP. As OOP is not just a concept it’s a whole new way of thinking.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time. Bye bye😊🖐
Itís difficult to find knowledgeable people about this subject, but you sound like you know what youíre talking about! Thanks
Glad it helped. Stay tuned for more.
Thanks for your blog, nice to read. Do not stop.
Glad you liked it. Sure will continue👍
Itís nearly impossible to find well-informed people for this subject, however, you seem like you know what youíre talking about! Thanks
Thanks a lot for your kind words. Stay tuned for more.
In all your gettings, get wisdom.
Thanks for another informative website. Where else could I get that type of info written in such an ideal way? I have a project that I am just now working on, and I have been on the look out for such info.
Thanks a lot for your kind words. I am happy that it helped you with your project. You can ask for any errors in your project I’ll try my best to help you with it.
Hi there, I enjoy reading all of your article post. I wanted to write a little comment to
support you.
Thanks a lot for your support.