Hello Pythonistas, welcome back. Today we will explore how to remove an image background using python.
We would use the removebg
module for this.
If you do not know remove.bg is a very popular and free background removal service.
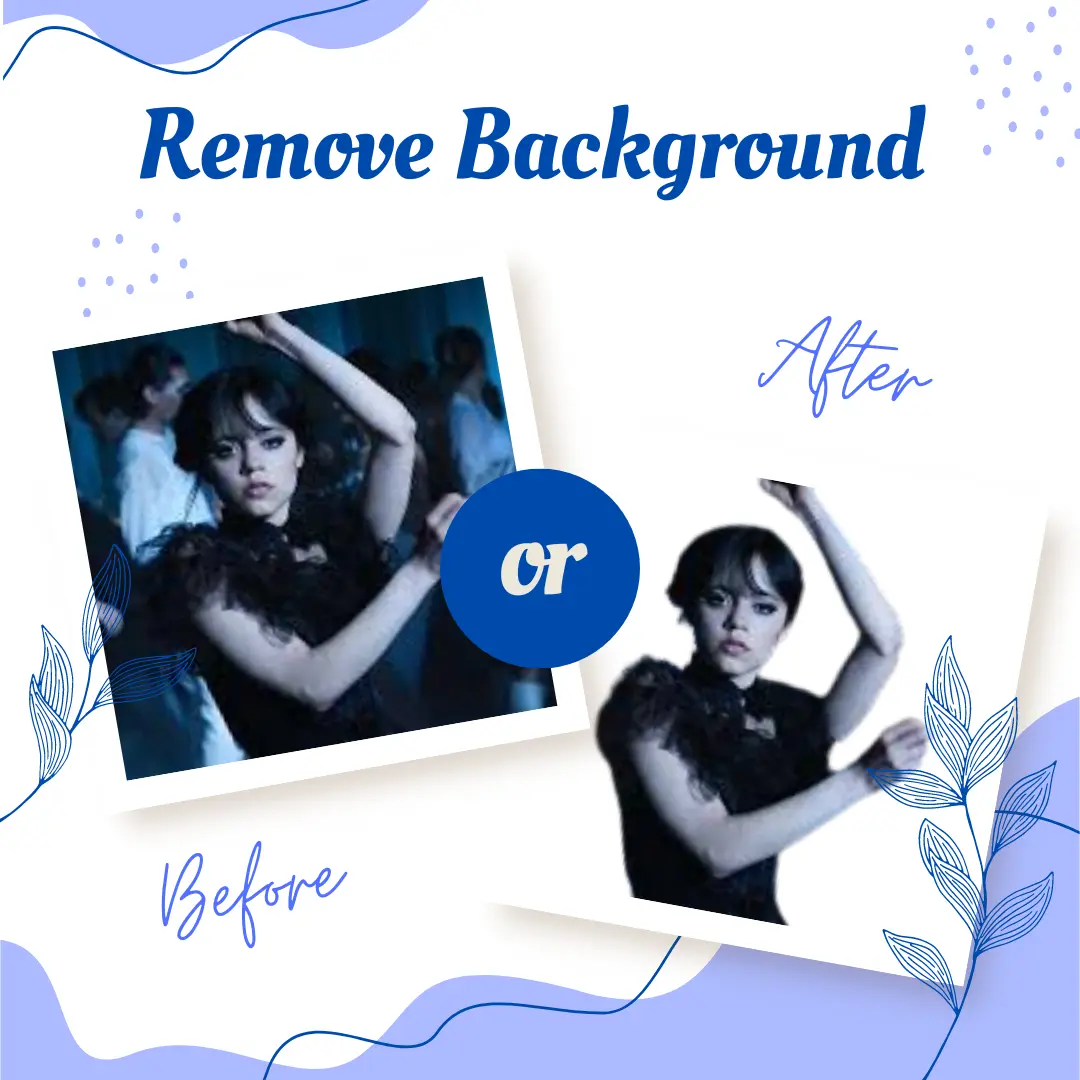
Contents
Get Your API Key
Sign Up for an Account:
- Visit the Remove.bg website.
- Click on the “Sign Up” button and create an account. If you already have an account, simply log in.
Get Your API Key:
- After logging in, go to the API section of the website.
- You will find your API key there. Copy the key.
Steps To Remove An Image Background using Python
First install the module:-
pip install removebg
Now, import the module and store the input path of the image in one variable and the output path.
from removebg import RemoveBg
input_path = 'projects//images//wednesday.jpeg'
output_path = 'projects//images//wednesday.png'
Connect with the service and remove the image’s background:-
API_KEY = 'your_api_key' # Replace with your actual API key
rmbg = RemoveBg(API_KEY, "error.log")
print("Start")
rmbg.remove_background_from_img_file(input_path)
print("Background removed")
This will create a file that has the image with the background removed.
To make that file have your desired name:-
# Rename the output file
import os
os.rename("projects/images/wednesday.jpeg_no_bg.png", output_path)
print("Final image saved")
Full Source Code
from removebg import RemoveBg
input_path = 'projects//images//wednesday.jpeg'
output_path = 'projects//images//wednesday.png'
API_KEY = 'your_api_key' # Replace with your actual API key
rmbg = RemoveBg(API_KEY, "error.log")
print("Start")
rmbg.remove_background_from_img_file(input_path)
print("Background removed")
# Rename the output file
import os
os.rename("projects/images/wednesday.jpeg_no_bg.png", output_path)
print("Final image saved")