Hello Pythonistas🙋♀️, welcome back. In the previous post, we built our first👆, small and cute😇 “Hello World!” app.
Today, we are going to see how to stylize💫 your app’s window and widgets the way you want.
Creativity wings🧚♀️ on… Let’s fly…
Contents
Before you get lost🤩 in the world of styles💫 and colors let’s see the previous post’s challenge’s🧗♀️ solution
Previous post’s challenge’s solution
# Import the CustomTkinter module
from customtkinter import *
# Create a class called App that inherits from CTk
class App(CTk):
# Define the constructor method for the App class
def __init__(self):
# Call the constructor method of the parent class
super().__init__()
# Create a CTkLabel widget and assign it to the instance variable 't'
self.t = CTkLabel(master=self, text="Hello World!")
# Pack the label widget to display it
self.t.pack()
# Check if this file is being run directly (as opposed to being imported as a module)
if __name__ == "__main__":
# Create an instance of the App class
app = App()
# Run the mainloop() method to start the application
app.mainloop()
This code uses the CustomTkinter module to create a graphical user interface (GUI) application.
It defines a class called “App” that inherits from the CTk class, which provides the basic framework for creating windows and widgets.
In the constructor method of the App class, a CTkLabel widget is created and assigned to the variable “self.t”.
The widget’s text is set to “Hello World!” and it is packed to display on the screen.
Finally, the code checks ✅️ if the file is being run directly and creates an instance of the App class.
This instance runs🏃 the mainloop() method to start the application and display the “Hello World!” message.
Colors
It is possible to customize the colors of all the CTk widgets.
Any widget majorly has 4 4️⃣ types of colors in it:
- bg_color
- fg_color and hover_color
- text_color
- border_color
bg_color
bg_color is the color behind the widget:
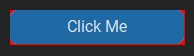
The red color you see behind the button is bg_color.
To set bg_color:
#either give it as an argument inside when you create the widget:
t = CTkLabel(master=app, bg_color="red", text="Hello World!")
t.pack()
#or
#use the configure method like this:
t.configure(bg_color="red")
fg_color and hover_color
fg_color is the blue color on the button in this image.
It is the foreground color for any of the widgets.
To set fg_color:
#either give it as an argument inside when you create the widget:
t = CTkLabel(master=app, fg_color="red", text="Hello World!")
t.pack()
#or
#use the configure method like this:
t.configure(fg_color="red")
hover_color is the color in the foreground when you take your mouse on it.
It will show in place of the blue color in this image.
To set hover_color:
#either give it as an argument inside when you create the widget:
b = CTkButton(master=app, hover_color="red", text="Click Me")
b.pack()
#or
#use the configure method like this:
b.configure(hover_color="red")
Note: hover_color can not be used in labels
text_color
text_color obviously is the color of the text in the widget.
It is the white color of the “Click Me” text in this image.
To set text_color:
#either give it as an argument inside when you create the widget:
t = CTkLabel(master=app, text_color="red", text="Hello World!")
t.pack()
#or
#use the configure method like this:
t.configure(text_color="red")
border_color
border_color is clearly the color of the border of any of the widgets.
It doesn’t show up in the image.
To set border_color we need to give a border_width too (I mean we can’t give color to something that doesn’t exist):
#either give it as an argument inside when you create the widget:
b = CTkButton(master=self, border_width=2, border_color="black", text="Click Me")
b.pack()
#or
#use the configure method like this:
b.configure(border_width=2, border_color="black")
Output:
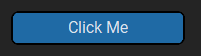
Note: border_color can not be used in labels
Themes
Imagine🤩 that you’re throwing a grand party🥳 at your house.
You’ve invited all your friends and family👨👩👧👦, but you want to make sure the party has a cohesive and stylish atmosphere.
So, you pick a theme – let’s say it’s a beach🏖 party theme.
You decorate your house🏠 with beachy colors, add beach balls and seashells, and maybe even put up a backdrop with a tropical beach scene.
In CustomTkinter, themes work in a similar way.
You can set a theme for your application, which will apply a consistent color scheme and visual style 🎉to all your widgets.
This means you can give your application a cohesive and professional look without having to style each widget manually.
Plus, you can easily switch themes if you want to change the atmosphere of your app – just like you might switch from a beach party theme to a Hollywood🎞 glamour theme for your next big event.
Here’s how you set the theme:
class App(CTk):
set_default_color_theme("green")
def __init__(self):
super().__init__()
self.b = CTkButton(master=self, text="Click Me")
self.b.pack()
if __name__ == "__main__":
app = App()
app.mainloop()
Currently, there are three3️⃣ themes.
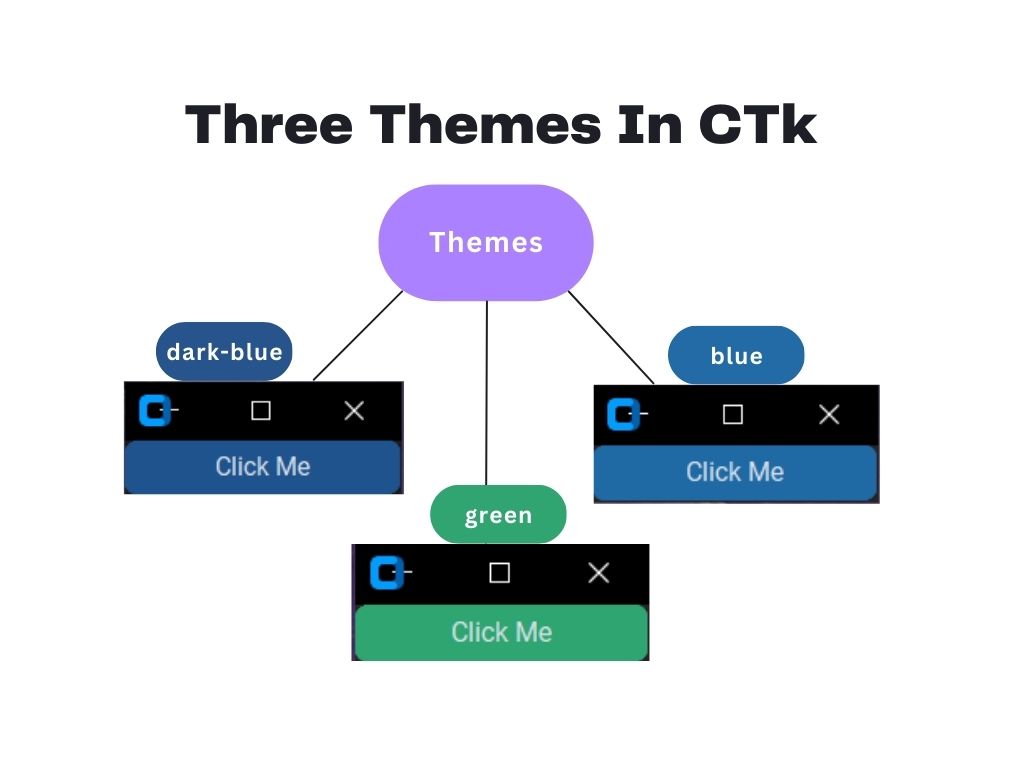
But in case you don’t like these themes, you can make your own.
Create your own theme
To make your own theme:
- First, you need to copy this code from the official doc.
- Now, create a new file with the
.json
extension and paste this code in this file. - You can easily change the colors inside this file.
- To get color codes for your fav color you can go to any color picker.
You can change the color for any widgets in CTk.
And yes you can give two colors for each of the widgets.
One for the light💡 mode and one for the dark mode.
If you give just one color then it will take the same for the light and dark theme.
To link this .json
file in your python code, we need to write its full path in the set_default_color_theme()
function:
set_default_color_theme("C:\Users\pyhon-hub\GUI\theme.json")
There you go your app is styled in your own way…
Appearance
As programmers, we usually like dark🔦 themes everywhere (and some like me are obsessed with it), Then why not in our own apps?
You can set your app’s theme to light💡, dark🔦, or system🖥.
By default, it’s set to system.
To change it:
from customtkinter import *
class App(CTk):
set_appearance_mode("light")
So, that’s how you make your window’s theme light, dark, or set to the system’s default theme.
And with that, we come to the end of this post.
Conclusion
Hope you guys enjoyed😄:
- playing with colors,
- experimenting with themes, and
- personalizing with appearance.
Keep playing, experimenting, and personalizing until you are satisfied.
I’ll see you in the next post, we will go on to the next step of making your first application…
Challenge 🧗♀️
Your challenge for this post is to create a small GUI window.
When you run the program it should display a random number between 1 to 10.
You’ll need to use the random module.
Stay happy 😄 and keep coding and do suggest any improvements if there.
Take care and have a great 😊 time I’ll see you soon in the next post…Bye Bye👋
Superb post however , I was wanting to know if you could write a litte more on this subject? I’d be very thankful if you could elaborate a little bit further. Kudos!
Sure, there will be more on this subject very soon…😊 Stay tuned
Hey are using WordPress for your site platform? I’m new to the blog world but I’m trying to get started and set up my own. Do you require any html coding expertise to make your own blog? Any help would be greatly appreciated!
If you are a beginner I suggest to use blogger as its free you should first experiment for some time and then 💵invest. For setting up a basic blog in WordPress you don’t need any coding experience its easy. This article might help. All the best for your blogging journey.😊👍 Hope I helped…
Thank you for another great article. Where else may anybody get that kind of info in such a perfect method of writing? I have a presentation subsequent week, and I am at the search for such info.
I got what you intend,saved to bookmarks, very nice internet site.
Thank you!
I’m really enjoying the theme/design of your weblog. Do you ever run into any internet browser compatibility issues? A couple of my blog audience have complained about my website not operating correctly in Explorer but looks great in Chrome. Do you have any solutions to help fix this problem?
Thank you for enjoying the design of our website! Browser compatibility can indeed be a tricky aspect of web development. Internet Explorer, especially older versions, tends to have difficulties displaying modern web elements accurately due to its lack of support for newer technologies and standards.
I am sorry I don’t have any real solution for this problem of yours.
All I can say is try to customize your website for this browser too.
My brother recommended I might like this web site. He was entirely right. This post truly made my day. You cann’t imagine simply how much time I had spent for this info! Thanks!
Thank you!!
First off I would like to say great blog! I had a quick
question that I’d like to ask if you don’t mind.
I was curious to know how you center yourself and clear
your head prior to writing. I have had a difficult time clearing my
mind in getting my ideas out there. I truly do take pleasure in writing but it just seems like the first 10 to 15 minutes are usually
wasted simply just trying to figure out how to begin. Any suggestions or
tips? Many thanks!
Heya! I just wanted to ask if you ever have any trouble with hackers?
My last blog (wordpress) was hacked and I ended up losing a few months of hard work due to no backup.
Do you have any methods to protect against hackers?
Sorry to hear that. The best I know is install wordfence or jetpack. I would advice to get an expert if these don’t work. All the best with your blog.
It’s pretty normal to be confused. Especially, when you are new. Don’t worry and keep working on it you’ll soon find it easy. Till then try to first see what readers want to read and then create an outline this way you won’t be lost and would exactly know what you want to write. A very Good luck for your writing…
Hey! This is kind of off topic but I need some advice from an established
blog. Is it very difficult to set up your
own blog? I’m not very techincal but I can figure things out pretty
fast. I’m thinking about creating my own but I’m not sure
where to begin. Do you have any tips or suggestions?
Many thanks
Great goods from you, man. I’ve keep in mind your stuff previous to and you are just too
great. I really like what you have bought here, really like what you are stating
and the best way through which you assert it.
You’re making it entertaining and you continue to care for to
stay it wise. I can not wait to read far more from you.
This is really a wonderful site.
Do you mind if I quote a few of your articles as long
as I provide credit and sources back to your weblog?
My blog is in the very same niche as yours and my users would certainly benefit from some of the information you present here.
Please let me know if this alright with you. Thanks a lot!
I was curious if you ever thought of changing the structure of your blog?
Its very well written; I love what youve got to say. But maybe you could a
little more in the way of content so people could connect with it
better. Youve got an awful lot of text for only having 1 or 2 images.
Maybe you could space it out better?
Yes you can quote but cannot copy the entire articles.
I’ll surely take your suggestion in consideration. Thank you for the constructive feedback
Thank you very much. A lot more coming soon…
Not at all starting a blog is not difficult in fact it is fun and enriching. If you don’t know much of technical stuff just head over to WordPress, Wix, or Blogger. Wish you a very good luck for your blogging journey.