Are you looking to make your Tkinter application more interactive and responsive?
Well, you’re in the right place!
In this tutorial, we’ll dive into the world of Tkinter command binding, which allows you to link events—like mouse clicks or key presses—to specific functions.
Ready to make your application come to life?
Let’s go!
Contents
What is Tkinter Command Binding?
Tkinter command binding is all about getting your app to respond when users interact with it.
For instance, when a user clicks a button or presses a key, you want your application to do something—like display a message, open a new window, or maybe even trigger a mini-game.
This is achieved by associating a callback function with a particular event of a widget.
How Tkinter Command Binding Works
To bind an event to a function in Tkinter, you need to:
- Define a function as a callback (this is the action your widget will perform).
- Assign that function to the widget’s
command
option.
When the event happens, the callback function is automatically called.
Simple, right?
Let’s break it down with an example.
Creating a Simple Button Click Event
Here’s a straightforward way to connect a button click to a function:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
# Step 1: Define the callback function
def button_clicked():
label1["text"] = "Button Clicked"
# Step 2: Bind the function to a button using the 'command' option
button = ttk.Button(root, text='Click Me', command=button_clicked)
button.pack()
label1 = ttk.Label(root)
label1.pack()
root.mainloop()
When you run this code and click the button, you’ll see the message “Button clicked” printed in your label.
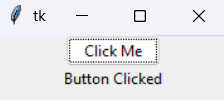
Important:
Make sure you pass the function name without parentheses—like command=button_clicked
—because if you use parentheses, the function will be called immediately when the program starts.
Adding Arguments to Command Binding
But what if you want your function to do something specific based on user input?
Easy!
You can pass arguments to your function using a lambda expression.
Here’s how:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
def button_clicked(option:str):
label1["text"] = option
button = ttk.Button(root, text='Rock', command=lambda: button_clicked("Rock"))
button.pack()
button1 = ttk.Button(root, text='Paper', command=lambda: button_clicked("Paper"))
button1.pack()
button2 = ttk.Button(root, text='Scissors', command=lambda: button_clicked("Scissors"))
button2.pack()
label1 = ttk.Label(root)
label1.pack()
root.mainloop()
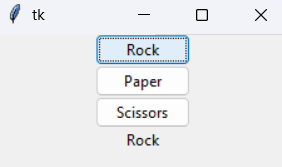
With this setup, clicking each button will display the corresponding option to the terminal—Rock, Paper, or Scissors.
It’s like building a mini-game!
Limitations of Tkinter Command Binding
While Tkinter command binding is super helpful, it’s not perfect. Here are some limitations you should be aware of:
- Not Available Everywhere: The
command
option isn’t supported by all widgets. It mainly works withButton
and a few others. - Limited Event Types: You can only bind the
command
option to certain events—most notably, mouse clicks and the backspace key. It doesn’t work with theReturn/Enter
key. - Inflexibility: Changing the binding of the command function can be tricky.
Overcoming These Limitations
Thankfully, Tkinter provides a more flexible alternative called Event Binding.
This approach lets you bind functions to almost any event—mouse clicks, key presses, hovering, you name it.
But that’s a topic for another day! In fact the next article most probably.
Summary
To wrap it all up, Tkinter command binding is a simple yet powerful way to make your widgets respond to events.
Just remember:
- Define a callback function.
- Assign it to the widget’s
command
option. - Enjoy your interactive application!
But don’t stop here!
Now that you’ve got the basics down, try experimenting with event bindings to enhance your app’s interactivity.
Challenge
Create a simple rock-paper-scissors game.
It should have 3 buttons rock paper and scissors.
When I click either computer will choose either of these 3 randomly from a list. (use random.choice)
Then we need to put a few if conditions to check whether the user won or the computer.
After you have built yours.
➡️Check To View The Solution’s Code To Solution
import tkinter as tk
from tkinter import ttk
import random
root = tk.Tk()
root.title("Rock-Paper-Scissors Game")
# Define the function that handles button clicks
def button_clicked(your_choice: str):
comp_choice = random.choice(["Rock", "Paper", "Scissors"])
choice_label["text"] = f"Computer's Choice: {comp_choice}\nYour Choice: {your_choice}"
if (comp_choice == your_choice):
result_label["text"] = "It's a Tie! 🤝"
elif (comp_choice == "Rock" and your_choice == "Paper") or \
(comp_choice == "Scissors" and your_choice == "Rock") or \
(comp_choice == "Paper" and your_choice == "Scissors"):
result_label["text"] = "You Win! 🎉"
else:
result_label["text"] = "You Lose! 😢"
# Creating the buttons and labels
ttk.Button(root, text='Rock', command=lambda: button_clicked("Rock")).pack()
ttk.Button(root, text='Paper', command=lambda: button_clicked("Paper")).pack()
ttk.Button(root, text='Scissors', command=lambda: button_clicked("Scissors")).pack()
choice_label = ttk.Label(root)
choice_label.pack()
result_label = ttk.Label(root)
result_label.pack()
root.mainloop()