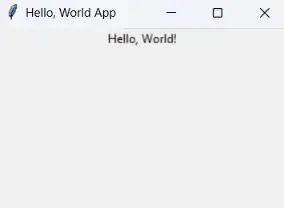
Tkinter Python Tutorial For Beginners
In this beginner-friendly tutorial, we’ll learn how to create your first Tkinter program.
Don’t worry—it’s easier than you think!
By the end of this tutorial, you’ll know how to display a window, add a label to it, and even fix common issues.
Let’s get started!
Tkinter Python Tutorial For Beginners
Contents
Step 1: Creating a Window
In any Tkinter program, the first thing you need is a window.
This window will act as a container for your app.
import tkinter as tk
root = tk.Tk()
root.mainloop()
What’s Happening Here?
Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners
Import Tkinter:
We first import Tkinter using:
import tkinter as tk
This line brings the Tkinter library into your program.
We give it the nickname tk
so we don’t have to type tkinter
every time.
Create the Main Window:
Next, we create the main application window:
root = tk.Tk()
Here, Tk()
creates a window, and we store it in a variable called root
.
You can name it anything you want, but root
is a common choice.
Display the Window:
Finally, we use the mainloop()
method:
root.mainloop()
This keeps the window open on the screen.
Without it, the window would appear and disappear too fast to even notice!
Step 2: Adding a Label (Hello, World!)
Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners
Now, let’s make the window a little more interesting by adding a label.
This label will display the classic message, “Hello, World!”.
import tkinter as tk
root = tk.Tk()
# Adding a label
message = tk.Label(root, text="Hello, World!")
message.pack()
root.mainloop()
What’s Happening Now?
Creating the Label:
This line creates a label:
message = tk.Label(root, text="Hello, World!")
root
tells Tkinter that the label belongs to the main window.
text="Hello, World!"
is the message that the label will show.
Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners
Placing the Label:
This line makes the label visible:
message.pack()
Without pack()
, the label is created, but it won’t show up on the window.
Step 3: Troubleshooting Common Tkinter Issues
Sometimes, things don’t work as expected.
But don’t worry!
Here are some common problems and how to fix them:
1. ImportError on Linux (Ubuntu)
If you see this error:
ImportError: No module named Tkinter
It means Tkinter is not installed. To fix it, run this command in your terminal:
sudo apt-get install python3-tk
2. Fixing Blurry Text on Windows
Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners Tkinter Python Tutorial For Beginners
If the text in your app looks blurry on Windows, you can fix it with a little extra code.
Add this before the mainloop()
method:
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
To make your app work on all platforms (Windows, macOS, and Linux), use this version:
try:
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
finally:
root.mainloop()
This code works on Windows and won’t crash your app on other systems.
Let’s Put It All Together
Here’s the final version of our “Hello, World!” program, with everything in place:
import tkinter as tk
try:
from ctypes import windll
windll.shcore.SetProcessDpiAwareness(1)
finally:
root = tk.Tk()
message = tk.Label(root, text="Hello, World!")
message.pack()
root.mainloop()
Importance of Object-Oriented Programming (OOP) in GUI Programming
Let’s imagine you’re building a big GUI app.
You’ll probably have lots of buttons, labels, and event handlers. If you don’t organize your code, things could get messy pretty quickly!
That’s where Object-Oriented Programming (OOP) helps.
OOP allows you to organize your code into classes and objects.
Instead of writing everything in one place, you can create reusable components and keep your code clean and scalable.
Here’s how you can write the “Hello, World!” program using OOP:
import tkinter as tk
# Create a class for the Hello, World! app
class HelloWorldApp:
def __init__(self, root):
self.root = root
self.root.title("Hello, World App")
# Add a label to the window
self.label = tk.Label(self.root, text="Hello, World!")
self.label.pack()
# Create the main window and run the app
if __name__ == "__main__":
root = tk.Tk()
app = HelloWorldApp(root)
root.mainloop()
What’s Happening Here?
- Class Definition: We create a class called
HelloWorldApp
. This class handles the window and widgets. - The
__init__()
Method: This method runs automatically when we create theHelloWorldApp
object. It sets up the window and adds a label. - Creating and Running the App: At the bottom, we create the main window (
root
), make an instance of our app class, and run the event loop withroot.mainloop()
.
By using a class, you keep your code more organized. As your app grows, you can add more methods and widgets without things getting messy.
Conclusion
Congratulations! You’ve just built your first Tkinter app.
You’ve learned how to create a window, add a label, and troubleshoot common problems.
Tkinter is fun and easy to learn, and this is just the beginning.
Keep practicing, and soon you’ll be building more advanced GUIs.
Happy coding! 💻
Common FAQs
What is the easiest GUI to learn for Python?
The easiest GUI library to learn for Python is Tkinter. It’s built into Python, so you don’t need to install anything extra. Tkinter has a simple syntax, and you can create a basic window in just a few lines of code. Plus, there’s a lot of community support and tutorials available, making it perfect for beginners.
Is there a better GUI than Tkinter?
Whether a GUI is “better” depends on what kind of app you’re building:
Tkinter: Best for beginners and small-to-medium projects. It’s easy to learn, lightweight, and built into Python.
CustomTkinter: If you like Tkinter but want a modern look, this is a great choice. It’s simple and gives your app a more polished design.
PyQt/PySide: Ideal for more advanced projects. These libraries let you create professional-looking apps but can be harder to learn.
Kivy: Great if you want to build apps for both desktop and mobile. It’s useful for touch-friendly interfaces.
PyGTK: Best for building Linux-based apps.1
What is Tkinter used for?
Tkinter is a library in Python that helps you create graphical user interfaces (GUIs). It lets you add windows, buttons, labels, and more to your apps.
What does mainloop()
do?
The mainloop()
method runs an event loop. This loop keeps the window open and responsive until you close it.
Why do we need pack()
in Tkinter?
The pack()
method places widgets (like labels) in the window and makes them visible. Without it, the widget won’t appear.