Hello, Pythonistas Welcome Back.
Today we will see how to make Labels in Tkinter Python.
Contents
labels in tkinter
How Does ttk.Label (tkinter label) Look?
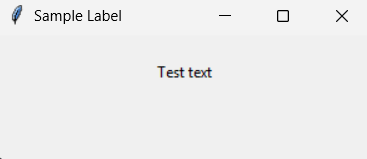
Basic Code
This is how you can make a simple Label in Tkinter (or ttk).
import tkinter as tk
from tkinter import ttk
root = tk.Tk() # Create the root window
root.title("Sample Label")
root.geometry("300x100")
# Creating label and packing it to the window.
label = ttk.Label(root, text="Test text")
label.pack(padx=20, pady=20)
root.mainloop()
How it works.
First, create a new instance of the Label
widget.
Second, place the Label
on the main window by calling the pack()
method.
If you don’t call the pack() function, the program still creates the label but does not show it on the main window.
The pack()
function is one of three geometry managers in Tkinter, including:
- Pack
- Grid
- Place
You’ll learn about this in detail in the upcoming tutorials.
How To Add Image To ttk.Label (tkinter label)?
To display an image label, you follow these steps:
- OGet an image (I’ll be using this image from Unsplash)
You can also download it from there or use any other image.
- Second, create a PhotoImage widget by passing the path of the image to the
PhotoImage
constructor. - Third, assign the
PhotoImage
object to theimage
option of theLabel
widget.
Complete code:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
root.title("Sample Image Label")
root.geometry("300x400")
# Creating label and packing it to the window.
photo = tk.PhotoImage(file='ocean.png')
label = tk.Label(root, image=photo)
label.pack(padx=20, pady=20)
root.mainloop()
Output:
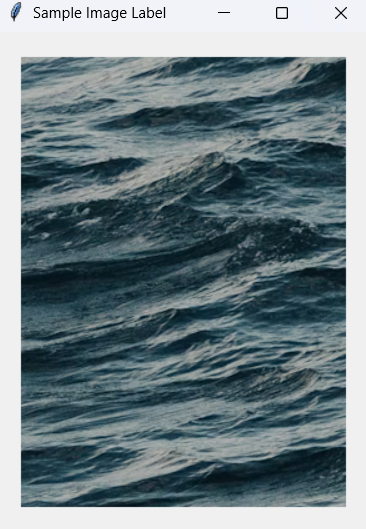
All Major Configurations
Option | Description |
---|---|
text | Specifies a text string to be displayed inside the widget. |
textvariable | Specifies a name whose value will be used in place of the text option resource. |
underline | If set, specifies the index (0-based) of a character to underline in the text string. The underline character is used for mnemonic activation. |
image | Specifies an image to display. |
compound | Specifies how to display the image relative to the text, in the case both text and images options are present. |
width | If zero or unspecified, the natural width of the text label is used. |
For details on the attributes refer ttk label’s documentation click here.
To see the label widget in the customtkinter click here.
labels in tkinter