Hello, Pythonistas🙋♀️ Welcome back. Do you like to do the same thing over and over again😵? Most probably not. There’s a reason why some people count sheep🐑 when they are unable to fall asleep😴. It’s because endlessly repeating something is boring🥱, and your mind can drop off to sleep more easily.
But there are certain repetitive tasks no matter how much we hate them. Thankfully, most programming languages have what is called a loop, which repeats things like statements or blocks. All to save you😓 from tons of hard work.
Today we are going to explore the for loops in python in depth.
Contents
For loops official documentation.
Before we get started here’s the solution to the previous post’s challenge.
Previous post’s challenge’s solution
calc = """
_____________________
| _________________ |
| | Pythonista 0. | | .----------------. .----------------. .----------------. .----------------.
| |_________________| | | .--------------. || .--------------. || .--------------. || .--------------. |
| ___ ___ ___ ___ | | | ______ | || | __ | || | _____ | || | ______ | |
| | 7 | 8 | 9 | | + | | | | .' ___ | | || | / \ | || | |_ _| | || | .' ___ | | |
| |___|___|___| |___| | | | / .' \_| | || | / /\ \ | || | | | | || | / .' \_| | |
| | 4 | 5 | 6 | | - | | | | | | | || | / ____ \ | || | | | _ | || | | | | |
| |___|___|___| |___| | | | \ `.___.'\ | || | _/ / \ \_ | || | _| |__/ | | || | \ `.___.'\ | |
| | 1 | 2 | 3 | | x | | | | `._____.' | || ||____| |____|| || | |________| | || | `._____.' | |
| |___|___|___| |___| | | | | || | | || | | || | | |
| | . | 0 | = | | / | | | '--------------' || '--------------' || '--------------' || '--------------' |
| |___|___|___| |___| | '----------------' '----------------' '----------------' '----------------'
|_____________________|
Welcome to pythonista Calculator
We support the following operations on two numbers:
- subraction
+ addition
* multiplication
/ division
** exponention
% modular division
"""
print(calc)
first_number = int(input("Enter 1st number:- "))
second_number = int(input("Enter 2nd number:- "))
operator_ = input("Enter the operator here:- ")
if operator_ == "-":
result = first_number - second_number
print(f"{first_number} {operator_} {second_number} = {result}")
elif operator_ == "+":
result = first_number + second_number
print(f"{first_number} {operator_} {second_number} = {result}")
elif operator_ == "*":
result = first_number * second_number
print(f"{first_number} {operator_} {second_number} = {result}")
elif operator_ == "/":
result = first_number / second_number
print(f"{first_number} {operator_} {second_number} = {result}")
elif operator_ == "**":
result = first_number ** second_number
print(f"{first_number} {operator_} {second_number} = {result}")
elif operator_ == "%":
result = first_number % second_number
print(f"{first_number} {operator_} {second_number} = {result}")
else:
print("You entered an invalid operator!!")
Output:-
_____________________
| _________________ |
| | Pythonista 0. | | .----------------. .----------------. .----------------. .----------------.
| |_________________| | | .--------------. || .--------------. || .--------------. || .--------------. |
| ___ ___ ___ ___ | | | ______ | || | __ | || | _____ | || | ______ | |
| | 7 | 8 | 9 | | + | | | | .' ___ | | || | / \ | || | |_ _| | || | .' ___ | | |
| |___|___|___| |___| | | | / .' \_| | || | / /\ \ | || | | | | || | / .' \_| | |
| | 4 | 5 | 6 | | - | | | | | | | || | / ____ \ | || | | | _ | || | | | | |
| |___|___|___| |___| | | | \ `.___.'\ | || | _/ / \ \_ | || | _| |__/ | | || | \ `.___.'\ | |
| | 1 | 2 | 3 | | x | | | | `._____.' | || ||____| |____|| || | |________| | || | `._____.' | |
| |___|___|___| |___| | | | | || | | || | | || | | |
| | . | 0 | = | | / | | | '--------------' || '--------------' || '--------------' || '--------------' |
| |___|___|___| |___| | '----------------' '----------------' '----------------' '----------------'
|_____________________|
Welcome to pythonista Calculator
We support the following operations on two numbers:
- subraction
+ addition
* multiplication
/ division
** exponention
% modular division
Enter 1st number:- 13
Enter 2nd number:- 2
Enter the operator here:- *
13 * 2 = 26
- Here, we first created three variables to store our inputs: two numbers and one operator.
- The default data type of the two numbers is taken as integers and for operators, it is taken as a string.
- Then, a number of if-else-elif statements are used to give the output according to the operator.
- Say, if the operator is – then, subtract, if it
+
then, add, and so on. - Next, we stored the result of the operation in a variable.
- And finally, printed the output using an f-string.
Inside the else statement we gave a statement printing an invalid operator because there is a chance that the user might enter an operator that we haven’t considered.
What is a for loop?
We will help out an old wizard🧙♂️ using for loop throughout this post.
You have already gone through sequences like lists, tuples, strings, etc. A for loop iterates(goes through them one by one) over these sequences.
Which means accessing their elements one by one.
Along with these sequences, a for loop also iterates over iterables.
For now iterable simply means objects whose elements can be accessed one by one. However, later when we will study OOP in python we will look at it in detail.
Ok, so Mr. Wizard🧙♂️ needs your help with his first task. He has stored all the items he wants to shop🛍 for in a python list. Now he wants you to print them all line by line so that he can review🧐 them correctly and can give them to the shopkeeper.
One way he knows is this:-
wizard_list = ["Wand", "Cauldron", "crystal phials", \
"telescope", "brass scales", "Owl", \
"Cat", "Toad", \
"Feather pen"]
print(wizard_list[0])
print(wizard_list[1])
print(wizard_list[2])
print(wizard_list[3])
print(wizard_list[4])
print(wizard_list[5])
print(wizard_list[6])
print(wizard_list[7])
print(wizard_list[8])
Output:-
Wand
Cauldron
crystal phials
telescope
brass scales
Owl
Cat
Toad
Feather pen
But, this much is too tiring😥 for him to write every time. To solve that we will use a for loop:-
wizard_list = ["Wand", "Cauldron", "crystal phials", \
"telescope", "brass scales", "Owl", \
"Cat", "Toad", \
"Feather"]
for items in wizard_list:
print(items)
Output:-
Wand
Cauldron
crystal phials
telescope
brass scales
Owl
Cat
Toad
Feather pen
First, we created a list that had the items Mr. Wizard🧙♂️ wanted to shop for. And then we used for loop to iterate(go through one by one) over the list and display all the items in it.
For loop syntax:-
for any_variable_name in Sequence: # any_variable_name would carry individual elements of sequence in every iteration
statements to be executed for all the elements of the sequence.
Flow chart:
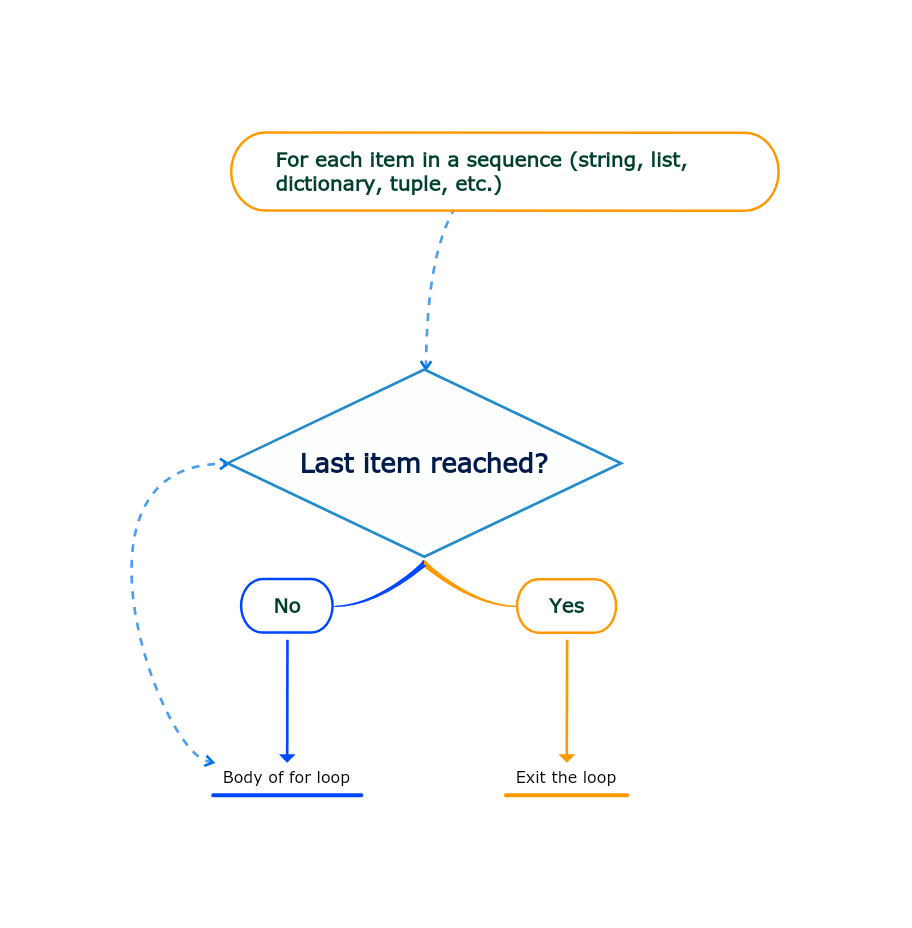
Break Statement In For Loop
**The break statement terminates the loop and moves on to the next lines of code.**
Mr. Wizard🧙♂️ has a maths class today and his teacher👩🏫 has given him a task to identify the number from a list that is divisible by 5.
Now Mr. Wizard🧙♂️ a poor old man doesn’t remember any math🔢 at this old age. But he has to go and study because of a curse he got when he was a child👶.
He resorts to you for help. You can quickly help him using the for loop, the if statement, and the break statement.
numbers = [51, 32, 63, 74, 45, 36, 47]
for num in numbers:
if num % 5 == 0:
print(num)
break
Output:-
19
Here we first iterated over the given list using the for loop.
Then, we used a conditional statement if
to check whether the number is divisible by 5 or not.
(if a number is divisible by 5 then it will have a remainder 0 when divided by 5 that is why we used num %
5 == 0
as our condition.)
Next, if the number is divisible by 5 the number will be printed.
After that, the loop will be terminated because of the break statement.
We did this because there was only one element in the list that was divisible by 5 and there is no point in checking the other numbers when we have found that one number.
Continue Statement In For Loop
**The continue statement skips an iteration, it forces to execute the next iteration of the loop while skipping the rest of the code inside the loop for the current iteration only **
Now the teacher👩🏫 has told him to tell which are the odd numbers in the list. As you might have guessed💡 already he has asked you for help.
numbers = [51, 32, 63, 74, 45, 36, 47]
for num in numbers:
if num % 2 == 0:
continue
else:
print(num)
Output:-
51
63
45
47
We first iterated through the list.
Then set a condition to skip the current iteration if the number was an even number.
(if a number divided by 2 gives the remainder 0 then it is an even number. that is why we gave the condition num % 2 == 0
)
After that, we simply printed a number if it wasn’t even.
Pass Statement In For Loop
**The pass statement is used as a placeholder for future code.**
It is useful when you don’t know exactly what statements or code blocks you want to execute when the loop runs. Example:-
numbers = [51, 32, 63, 74, 45, 36, 47]
for i in numbers:
pass
The Range() Function
The range function is useful when we need to generate a sequence of numbers. For example, you want to print numbers from 0 to 10. Then instead of creating a list and then looping through it, we can use a range function to make our work easier:-
for i in range(11):
print(i)
Output:-
0
1
2
3
4
5
6
7
8
9
10
Syntax of range function:-
range(start_value, stop_value, step_value)
- Start_value = 0 is default
- Stop_value = it should be given 1 more than the value you want to stop at. eg if you want numbers till 10 write 11. Just like in the example above.
- Step_value = 1 is default. This is the number of increments, i.e if you give it as 2 and the range starts from 1 the second number would be 3. As 1 would be incremented by 2.
Most of the time when using a for loop you will use the range function. It is a super important part of the for loop.
Else In For Loop
You can easily use the else statement with for loops just like you used it with the if statements.
Mr. Wizard🧙♂️ wants you to create a program for him to help him find out which of the items are present in his messy room and which ones are to be bought.
find_item = input("Enter the item to be searched here:- ").lower()
items = {'wand': 5, 'telescope': 2, 'feather pen': 7}
for thing in items:
if thing == find_item:
print(f"{items[thing]} units of {thing} are present.")
break
else:
print('No stock left! You need to go and buy!!')
Output:-
Enter the item to be searched here:- wand
5 units of wand are present.
First, we took user input and converted it into lowercase for easier search.
Then we created a dictionary that held items along with the number of items left.
Next, we iterated through that dictionary(Yes you can iterate through dictionaries too) and set a condition to check whether the item asked for is present or not.
If it is present, then the item would be printed along with the number of items left; if not, no stock left would be printed.
For Loop List Comprehension
Sometimes making a list is tiresome😥 right if you want to create a list that stores all the alphabet you would need to type like this:
alphabet = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']
It would take a hell lot of time⏱and there are numerous chances of errors.❌❗ That’s where list comprehension can help you out. You can simply type this much:
alphabet = [i for i in "abcdefghijklmnopqrstuvwxyz"]
Here we simply told python to insert i
in our list every element of the given string. Look at it in the form of a normal for loop to get a better understanding of this:-
for i in "abcde":
print(i)
Output:-
a
b
c
d
e
Conclusion
In this post we understood the following concepts by helping Mr. Wizard and a few other examples:
- What is a for loop?
- Break statement in for loop
- Continue statement in for loop
- Pass statement in for loop
- The range() function
- Else in for loop
- For loop list comprehension
Challenge🧗♀️
Your challenge for this post is to print the following patterns using for loop and the concepts you have learned by now.
pattern-1
Take an integer input n from the user. And for n print this pattern. Eg. n=5
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
pattern-2
Take an integer input n from the user. And for n print this pattern. Eg. n=5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
pattern-3
Take an integer input n from the user. And for n print this pattern. Eg. n=5
*
* *
* * *
* * * *
* * * * *
You will need to use:-
- nested for loops;
- end parameter of the print statement;
- a bit of logic;
- and lots of errors especially if you are new to programming.
**range() function will help you a lot here.**
When I just started off and was given this problem to be solved it took me nearly a week to just be able to print the first pattern, and a whole another to understand how it did happen.
So all I want to tell you is that by this time you may have started becoming unable to solve problems and look for solutions and are even unable to understand the solutions even after reading them multiple times.
But, trust me it’s all a learning curve you will be able to solve this and even bigger problems later on.
For more such pattern programs and their solutions, you can consider visiting this site. I found it really helpful. Click here to visit.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊