Were you a naughty😜😝 child in school who constantly got punishments like:- write “I will not do this again” 100 times?
Do you want to know how Python can make punishments😩like this super🤩easy for you with its functions?
Keep on reading and you will get to know, how.
Contents
Previous post’s challenge’s solution
Pattern-1
n = int(input("enter a number:- "))
i = 1
while i <= n :
j = 1
while j <= i:
print(i, end = " ")
j += 1
print()
i += 1
Output:-
enter a number:- 5
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
Pattern-2
n = int(input("enter a number:- "))
i = 1
while i <= n :
j = 1
while j <= i:
print(j, end = " ")
j += 1
print()
i += 1
Output:-
enter a number:- 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Pattern-3
n = int(input("enter a number:- "))
i = 1
while i <= n :
j = 1
while j <= i:
print("*", end = " ")
j += 1
print()
i += 1
Output:-
enter a number:- 5
*
* *
* * *
* * * *
* * * * *
What is a function in python?
You have used a number of functions in python by now like print(), type(), input(), split(), append(), etc.
What was the common thing you noticed in all of them?
They all are chunks of code that tell python to do something. Like the print() function tells python to display the given text/number as the output.
You must be thinking how are these functions chunks of code, we can only see a word with parenthesis. Well to understand this go to the visual studio code/pycharm then type print(10)
and then press the ctrl
key and click on the print
.
You’ll see something like this:-
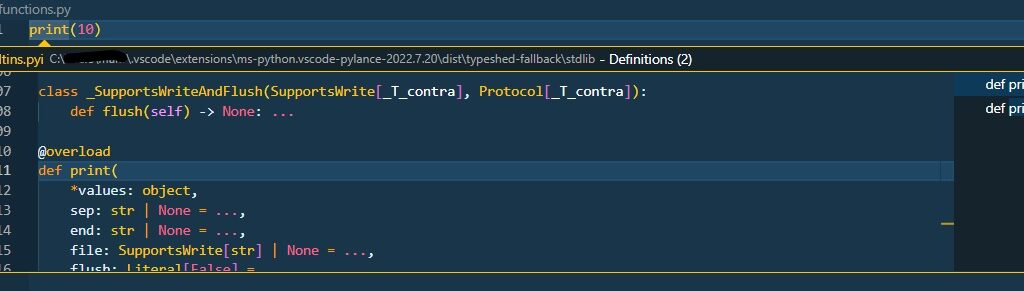
This is the code that runs behind the scene when you call print()
.
Now you know, that you have been using python’s built-in functions already in fact, from your very first program.
Are you thinking about how will they be helping you out in your punishments?
Well, they will directly not help you out with this, you’ll need to create a function of your own.
How to define your own function in python?
NOTE: A parameter is the variable listed inside the parentheses in the function definition. An argument is the value that is sent to the function when it is called.
Say for example you are given a punishment to type I won’t do this again 100 times. This is because you played a prank on your class teacher and she lost all her beautiful hair due to that.
Here’s how you can make a function of your own to get this done in just a few lines of code:
def punishment(text_to_be_typed):
"""This function simply inputs a string and prints it a 100 times"""
for i in range(1, 101):
print(i, text_to_be_typed)
punishment("I won't do this again")
Output:-
1 I won't do this again
2 I won't do this again
3 I won't do this again
4 I won't do this again
5 I won't do this again
...
96 I won't do this again
97 I won't do this again
98 I won't do this again
99 I won't do this again
100 I won't do this again
Here are the steps we followed to do this:
- First, we created a function punishment using the keyword
def
. It means to define. So, we have defined a function named punishment taking a parametertext_to_be_typed
. Heretext_to_be_typed
is a variable that will have a value that we give to our function when we call it. - Next, we gave our function a docstring. A docstring is given just after creating a function inside a triple quote string. It explains the working, features, input, and output of a function.
- After giving the name, parameter, and docstring to our function we then made it perform some action. The action was to print I won’t do this again 100 times. We used a for loop and the range function for this.
Finally, we need to call a function to use it. We did that by writing the function name followed by a parenthesis and inside them the required argument.
SYNTAX FOR MAKING YOUR OWN FUNCTION IN PYTHON:-
def function_name(parameter):
"""docstring"""
tasks to be performed
by the function
NOTE:-
- giving a parameter inside a function is not compulsory.
- also, you can give more than one parameter.
- a docstring is also not compulsory. But it’s good to have one for the clarity of your code.
- if you have not decided what task the function should perform, you can use the
pass statement
inside function.
Docstrings though not compulsory are extremely useful. They enable you to know about a function’s inputs, processes, and outputs without the need to read its code.
Here’s how you can access any function’s docstring in python:
print(input.__doc__)
Output:-
Read a string from standard input. The trailing newline is stripped.
The prompt string, if given, is printed to standard output without a
trailing newline before reading input.
If the user hits EOF (*nix: Ctrl-D, Windows: Ctrl-Z+Return), raise EOFError.
On *nix systems, readline is used if available.
Arguments in a function
Since you are a very naughty child your teacher this time punished you for not behaving well with others. She told you to greet everyone you meet.
So, you being a smart Pythonista decided to make a function to do that for you.
def greet_each(name, message):
"""this function greets the person with the given message"""
print(f"Hey {name}, {message}")
greet_each("Me", "Good Morning")
Output:
Hey Me, Good Morning
Required arguments
If you go lazy and do not write the message inside the function as an argument then you will get an error, see:-
def greet_each(name, message):
"""this function greets the person with the given message"""
print(f"Hey {name}, {message}")
greet_each("Me")
Output:
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\functions.py", line 5, in <module>
greet_each("Me")
TypeError: greet_each() missing 1 required positional argument: 'message'
This happens because the message is a required argument if you don’t pass it the function won’t function(nice rhyme😏).
The same will happen if you don’t give the name
argument inside the function. And the error will give missing 2 required… if you don’t write any of them.
The moral of the story is that all the parameters you give this way while defining the function become required arguments which means your function cannot function without them.
Default Arguments
If you want a way to sort this problem of required arguments you will need to use default arguments. You can simply set the message to by default have “Good Morning” as a default value.
def greet_each(name, message = "Good Morning"):
"""This function greets to
the person with the
provided message.
If the message is not provided,
it defaults to "Good
morning!"""
print(f"Hey {name}, {message}")
greet_each("Me")
Output:
Hey Me, Good Morning
This way you can type a different message only when you want to greet someone differently. (Like the teacher who gave you a punishment to stay an extra hour at school daily)
Arbitrary Positional Arguments
These types of arguments will be useful to you in case you meet around 10 or more than that number of people a day. I mean even after easing your work using the greet_each()
function it will still be difficult for you to deal with them.
Here’s a solution to this problem.
def greet_each(message, *names):
"""this function greets all the person with the given message"""
for name in names:
print(f"Hey {name}, {message}")
greet_each("Good Morning", "Me", "My best friend", "My teacher")
Output:
Hey Me, Good Morning
Hey My best friend, Good Morning
Hey teacher, Good Morning
Notice how you can pass any number of names as an argument to the function. And the variable names
as a whole is accepted as a tuple.
You cannot, however, give names
before the message
if you try to do so you’ll face an error.
These types of arguments are called Arbitrary positional arguments. In the function, we use an asterisk (*) before the parameter name to denote this kind of argument.
This is the *args you’ll see in the future in a lot of python functions.
Arbitrary keyword Arguments
Say you want to greet everyone with different messages. There you can use **kwargs
, i.e, arbitrary keyword arguments. They allow to input data in the form of a dictionary.
def greet_each(**names_greetings):
"""this function greets all the person with the given specific message"""
for name, greeting in names_greetings.items():
print(f"Hey {name}, {greeting}")
greet_each(Me = "Have a great day", My_best_friend = "What's up buddy",\
teacher = "Good morning ma'am you're the best teacher")
Output:
Hey Me, Have a great day
Hey My_best_friend, What's up buddy
Hey teacher, Good morning ma'am you're the best teacher
We used the items function of the dictionary to extract name
and greeting
separately from the names_greetings
dictionary.
The return Statement
By now our functions do not return any values. They just print the desired output. The return
statement is used to return something and then exit the function. I know you feel confused.
Let’s say for example your teacher👩🏫 is impressed with your python skills👩💻 and wants you to make a calculator that can take two values and an operator and then store the result in a result variable.
You can do it simply using if-else-eilf statements as you did here. But, it would not be the best way and repeated use will require a bunch of code.
Here’s how you can make such a calculator using your own function.
if you are not following along with this blog just go and read this solution and you’ll be able to get along.
def calculator(first_number, second_number, operator_):
if operator_ == "-":
result = first_number - second_number
return f"{first_number} {operator_} {second_number} = {result}"
elif operator_ == "+":
result = first_number + second_number
return f"{first_number} {operator_} {second_number} = {result}"
elif operator_ == "*":
result = first_number * second_number
return f"{first_number} {operator_} {second_number} = {result}"
elif operator_ == "/":
result = first_number / second_number
return f"{first_number} {operator_} {second_number} = {result}"
elif operator_ == "**":
result = first_number ** second_number
return f"{first_number} {operator_} {second_number} = {result}"
elif operator_ == "%":
result = first_number % second_number
return f"{first_number} {operator_} {second_number} = {result}"
else:
return "You entered an invalid operator!!"
addition = calculator(10,20,"+")
subtraction = calculator(10,20,"-")
multipliction = calculator(10,20,"*")
division = calculator(10,20,"/")
exponent = calculator(10,2,"**")
modular_division = calculator(10,20,"%")
print(addition, subtraction, multipliction, division, exponent, modular_division, sep="\n")
Output:
10 + 20 = 30
10 - 20 = -10
10 * 20 = 200
10 / 20 = 0.5
10 ** 2 = 100
10 % 20 = 10
- First, we created a function calculator taking three arguments two numbers, and one operator.
- Then, to decide which operation is to be performed when we gave a bunch of if-else-elif statements to check which operator is given in the input. And perform the operation accordingly.
- Next, we returned the result of the operation using the
return
statement. That means we used thereturn
keyword to return the result. - The
return
statement does not print the result as output instead it allows you to store the result inside a variable as an object.
All this means that whenever you call a function that returns something. The function is replaced by the result of the return statement and it is an object.
You can now easily use this function multiple times or can put it inside of a loop to run it till the user wants it.
Scope of variables
There can be two types of variables when you are using functions:-
- global and
- local.
Their classification is on the basis of the portion of the program where the variables are recognized.
This means where you can access those variables.
Global variables
Global variables can be accessed from anywhere in the program. Be it inside the function or outside it. For example:
y = 100
def any_function():
print(y)
any_function()
print(y)
Output:
100
100
Here y is a global variable because it can be accessed(used) from both inside and outside the function.
local variables
Local variables can only be accessed inside the function and not anywhere else in the program. For example:
def any_function():
x = 100
print(x)
any_function()
print(x)
Output:
100
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\functions.py", line 7, in <module>
print(x)
NameError: name 'x' is not defined
Here x
is a local variable because it can only be accessed(used) inside the function it has no existence outside it.
Conclusion
So, in this post, we saw what are functions, and also learned to create our own functions which simplified our punishment of typing something 100 times. We also saw how we can give arguments function in different ways.
After that, we made a calculator function to understand the return statement.
And lastly saw that the scope of variables can be local and global.
Challenge🧗♀️
Your challenge for this post is to create a suggest_places to travel function. You can use as many countries as you want. A minimum of three countries should be there.
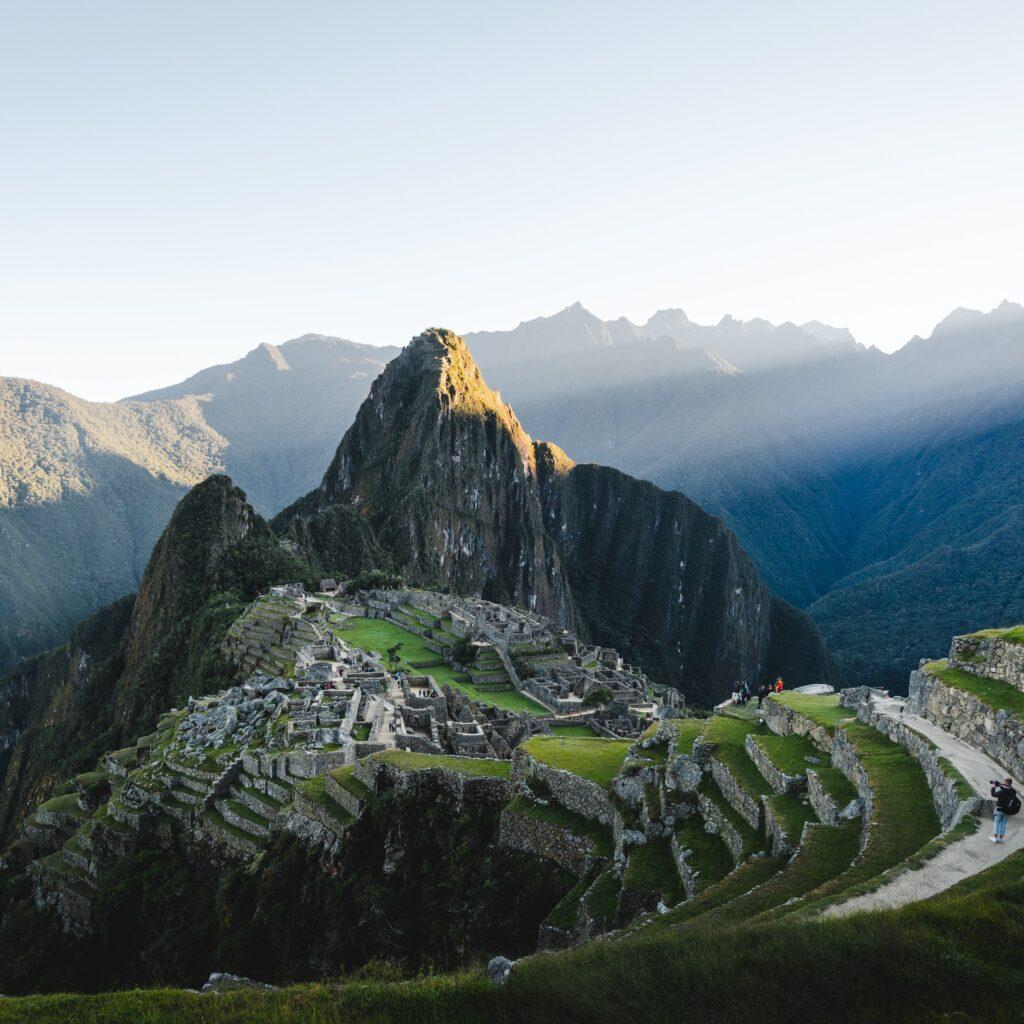
Your function should be like this:-
- Takes the name of a country as input.
- Suggests some places to travel to in that country.
- It should have a dictionary that stores the country name as key and the places as value.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊
Great goods from you, man. I’ve understand your stuff
previous to and you’re just too wonderful. I really
like what you’ve acquired here, certainly like what you are stating and the way in which you
say it. You make it enjoyable and you still take care
of to keep it smart. I can not wait to read much more from you.
This is actually a terrific web site.
Great to know it helps. Stay tuned for more