Hello Pythonistas, welcome back.
Here we will see what are lists in python
Contents
What are lists in python?
You know that numbers in python help you with math and finance stuff, and strings help you with stories, essays, and a lot more.
A list too simply is a data type in python that will help you with your shopping list, to-do list, bucket list, and a lot more.
A list in python is a data type that stores data in an ordered manner.
It is always enclosed inside square brackets [ ]. Here’s an example:-
shopping_list = ["fruits", "veggies", "nuts", "milk"]
print(shopping_list)
Output:-
['fruits', 'veggies', 'nuts', 'milk']
You can also make a list containing numbers(integer, float, complex, boolean) like the one below:-
marks = [98, 95, 97, 96, 89]
print(marks)
Output:-
[98, 95, 97, 96, 89]
You can also create lists having elements of more than one data type like this one👇:-
mixed = [98, "hi", 9.7, "happy", 89+3j]
print(mixed)
Output:-
[98, 'hi', 9.7, 'happy', (89+3j)]
NOTE:- indexes start from 0 in lists just like they did in strings. Python doesn’t view lists the same as we do it views them like this👇
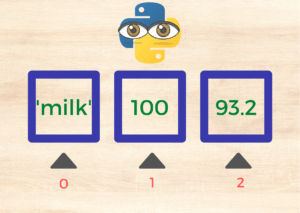
I hope you are clear about what are lists in python.
List Accessing And Slicing
Now that you are clear with what are lists in python let’s see how can you access a portion of it.
Accessing lists
Accessing a list is the same as accessing a string.
It simply means that elements of a list can be individually accessed.
lsit1 = ["milk", 100, 93.2]
print(list1[1])
Output:
100
You can also use negative indexes to access elements.
Always remember negative indexes start from-1
, not 0
!!
lsit1 = ["milk", 100, 93.2]
print(list1[-2])
Output:
100
Slicing lists
Slicing a list is the same as slicing a string.
It simply means that elements of a list can be accessed in small parts.
list2 = ["milk", 100, 93.2,"hello", "python"]
print(list2[1:4:2])
Output:
[100, 'hello']
First comes the start index number then, comes the stop index number, and lastly comes no. of steps, you want to take.
Here too, you can use negative indexes like this:-
list2 = ["milk", 100, 93.2,"hello", "python"]
print(list2[-2:-5:-2])
Output:
['hello', 100]
NOTE:- Anyone or two index values can also be negative it is in no way necessary to use all negative index numbers.
However, it is not suggested to use the negative values in step as there are chances of errors or unexpected output.
If this seems quite fast and confusing I strongly suggest you read string accessing and slicing.
This would help you relate both concepts and would help you understand both of them better. Click here to read them.
Just wanted to let you know how impressive I find your article to be. The clarity of your post is remarkable, leading me to believe that you are an authority on this subject. If it’s okay with you, I’d like to subscribe to your feed in order to be notified of future posts. Your work is truly appreciated. Please continue this gratifying endeavor.
Thank you. You can join the whatsapp channel for further updates.