Do you like adventures🏕 🏜 🏔 🛤? Well, I do.
The for loops that we saw in the last post were like a bit less scary, known adventure. Whereas, the while loops that we are gonna discuss in this post are like an unknown and possibly an endless adventure.
Seems exciting🤩? Keep on reading and we will together see how this example can clearly make you understand while loops.
While loop official documentation.
Contents
Before we get started, here’s the solution to the previous post’s challenge.
Previous Post’s challenge’s Solution
Pattern-1
n = int(input("enter a number:- "))
for i in range(1, n+1):
for x in range(i):
print(i, end=" ")
print()
Output:-
enter a number:- 5
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
- We first created a variable to input an integer from the user.
- Then, we started a for loop which will work in the range of
1
ton+1
numbers. - After that, we started a for loop inside the for loop(nested for loop in fancy terms). This loop works for the range
0
toi
. - Next, we printed
i
and gaveend=" "
(space) so that the value is printed on the same line. - And lastly, we gave an empty print statement to print the next line of the pattern in the new line.
Read this flow chart to understand how will this loop function to print out the pattern.
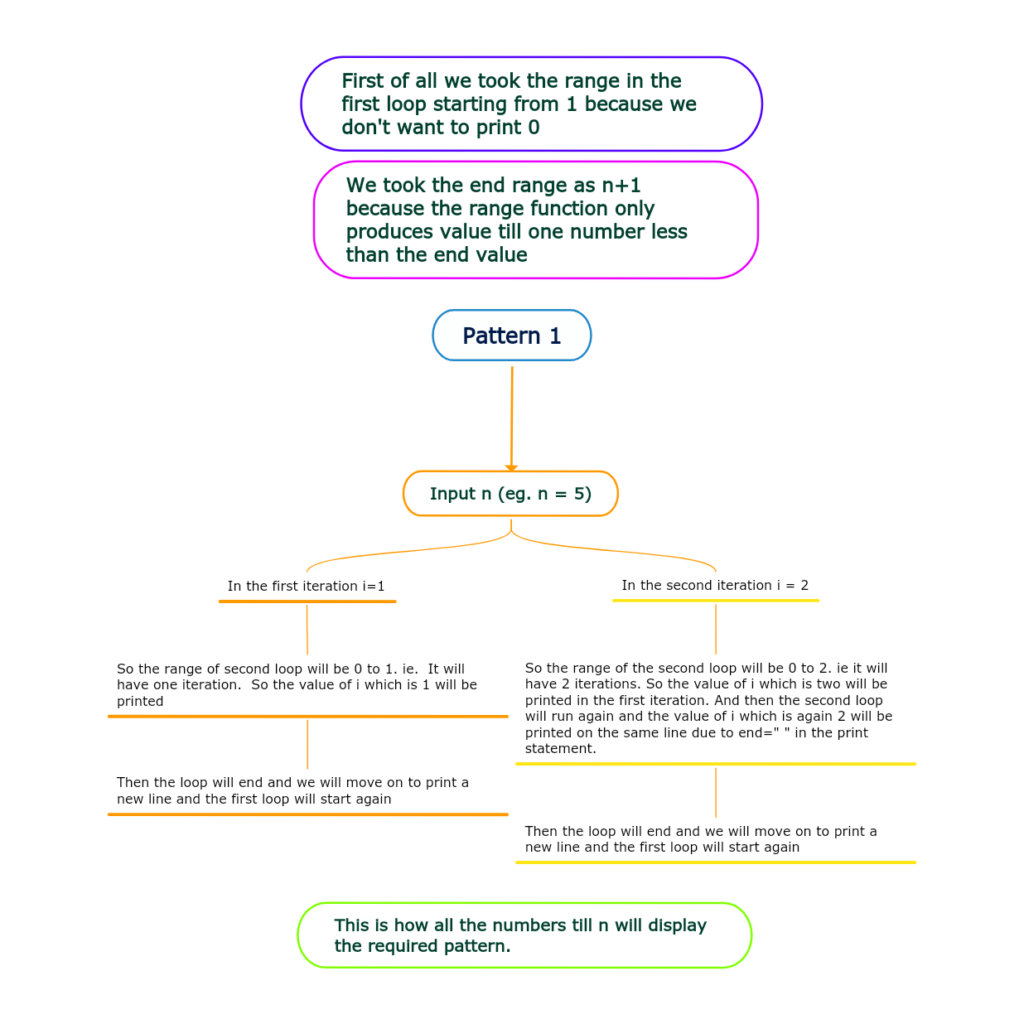
Pattern-2
n = int(input("enter a number:- "))
for i in range(1, n+1):
for x in range(1, i+1):
print(x, end=" ")
print()
Output:-
enter a number:- 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
- We first created a variable to input an integer from the user.
- Then, we started a for loop which will work in the range of
1
ton+1
numbers. - After that, we started a for loop inside the for loop(nested for loop in fancy terms). This loop works for the range
1
toi+1
. - Next, we printed
x
and gaveend=" "
(space) so that the value is printed on the same line. - And lastly, we gave an empty print statement to print the next line of the pattern in the new line.
See how the only difference between both the patterns is that in the first one we printed i
and in the second one, we printed x
.
And in the first pattern, we took the range of the loop from 0
to i
and in the second pattern, we took the range 1
to i+1
.
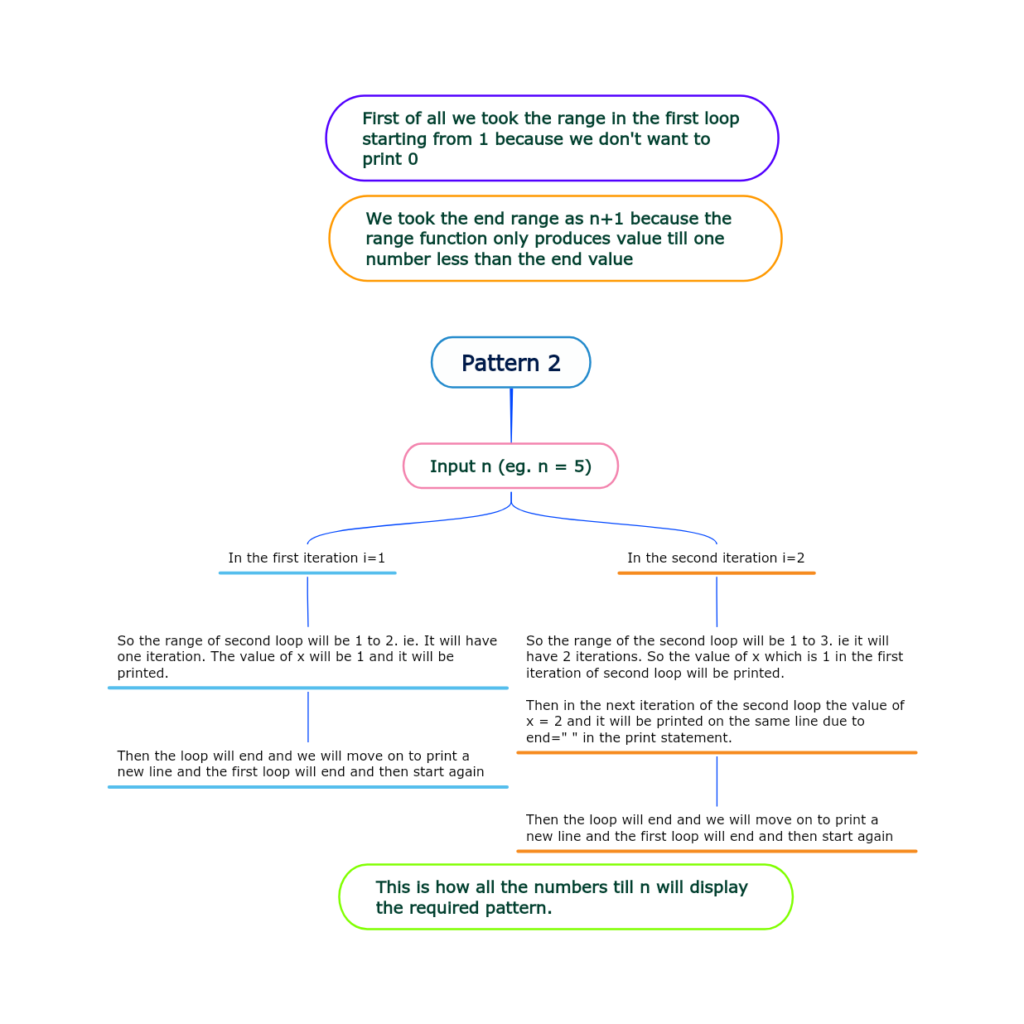
Pattern-3
n = int(input("enter a number:- "))
for i in range(1, n+1):
for x in range(i):
print("*", end=" ")
print()
Output:-
enter a number:- 5
*
* *
* * *
* * * *
* * * * *
- Pattern 3 is the easiest of all especially if you figured out the first one.
- The only change we made in pattern 3’s solution from the 1st one is that instead of printing
i
we printed"*"
this string.
If you are facing any doubts about any of these three solutions feel free to comment your doubts below I will definitely help you with them.
Now let’s dive🏊♀️ into the infinite adventure pool💦of while loops.
What Are While Loops In Python
Unlike the for loops which are usually used to iterate over sequences(when the number of iterations is known) while loops are used when we don’t know how many iterations we would have to make.
For example, if you go to an adventure🏕 park there are a limited number of rides or adventures to be enjoyed, your journey there lasts as long as there are adventures to explore:-
adventure_park = ["roller coaster", "hot air balloon ride", "scuba diving", "bungee jumping", "trekking"]
for a in adventure_park:
print(a)
Output:-
roller coaster
hot air balloon ride
scuba diving
bungee jumping
trekking
But instead of going to an adventure park, if you decide to go to a place like Amazonia🏞🌄.
Where the denseness and enormity of the jungle, its massive amount of rainfall, and its variety of dangerous animals — piranhas🐡, boa constrictors, jaguars🐆, and poisonous arrow frogs🐸 can make your survival alone hard to believe😱.
You’ll meet an unknown number of adventures to be explored. And your adventure will last there until/unless you decide to give up:
Want_to_give_up = False
while Want_to_give_up is False:
print("A new daring adventure!\n")
Want_to_give_up = input("Enter y if you want to leave...\n>")
if Want_to_give_up.lower() == "y":
Want_to_give_up = True
print("Have a good day...")
else:
Want_to_give_up = False
Output:-
A new daring adventure!
Enter y if you want to leave…
>n
A new daring adventure!
Enter y if you want to leave…
>n
A new daring adventure!
Enter y if you want to leave…
>n
A new daring adventure!
Enter y if you want to leave…
>y
Have a good day…
In case you find this code hard to read here are 4 concepts you need to revise:- variables, boolean, if-else statements, and escape sequences.
I hope now you know why I called while loop an unknown adventure. This is because we use a while loop when we don’t know when to stop looping through the statements.
While loop syntax:
while condition_to_be_fulfilled:
statements
code blocks
While loop flow chart
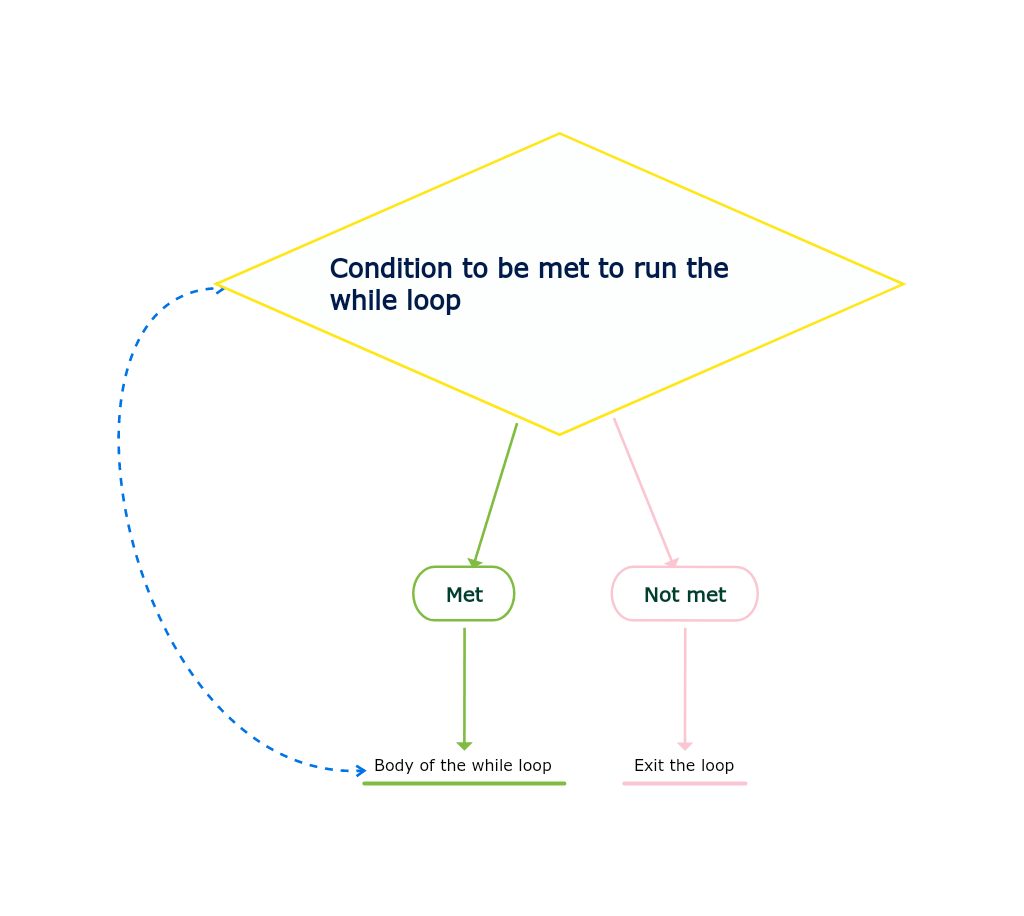
When to use for loops and while loops
Ok, so the above explanation might have already✔ answered this question for you. But, still, I would like to leave no scope for confusion😵.
The for loops are used when you know how many iterations you are gonna have like in the Bagles game or a number guessing game.
And a while loop is used when you have no idea for the number of iterations required like in a game where you need to keep on running the game until/unless the player loses.
Infinite while loop in python
You remember in the start I said: “while loops are like an unknown and possibly an endless adventure.”
You got to know why it is like an unknown adventure. But, aren’t you wondering🤔 why it can be an endless adventure?
It is because a while loop can be an infinite loop. This means a while loop that runs🏃♀️ indefinitely(in theory).
This can happen in a while loop because it does not update the value of the variable itself, we need to do that explicitly.
Such loops usually are the results of some bugs(errors/mistakes). Let’s see what one looks like and how can you stop one.
The code below is the same as the one we used in the what are while loops section. But here we did an error, we forgot to update the value of Want_to_give_up
inside the loop. This would result in an endless loop because the value of Want_to_give_up
always remains False.
❗⚠Warning: Don’t run this program without knowing how to stop it.
Want_to_give_up = False
while Want_to_give_up is False:
print("A new daring adventure!\n")
if Want_to_give_up == "y":
Want_to_give_up = True
print("Have a good day...")
else:
Want_to_give_up = False
Output:-
A new daring adventure!
A new daring adventure!
A new daring adventure!
.
.
.
A new daring adventure!
Traceback (most recent call last):
File "c:\Desktop\python-hub.com\while.py", line 4, in
print("A new daring adventure!\n")
KeyboardInterrupt
I pressed ctrl c
to stop this loop. That is why it shows an KeyboardInterrupt
error. Great😀. Now you know how to come out of infinite loops caused by a bug🐞.
Now there is another type of infinite loop the while True
loop.
In this, we intentionally start a loop to run indefinitely till a break
statement
is found, let’s see how to use it.
Loop control statements
These are the statements used to control the flow of a loop. They are useful in both for loops and while loops. We saw them for the for loops in the last post. Let’s see their use in the while loops.
Break statement
The break statement terminates the loop and moves on to the next lines of code.
Say for example you want to travel in Amazonia till you meet a jaguar.
while True:
print("\nA new daring adventure!")
adventure = input("What was the adventure?\n>>")
if adventure == "jaguar":
print("Bye Bye... Have a good day(if you stay alive)...")
break
Output:-
A new daring adventure!
What was the adventure?
>>had a 40km walk
A new daring adventure!
What was the adventure?
>>had nothing to eat for two days
A new daring adventure!
What was the adventure?
>>jaguar
Bye Bye… Have a good day(if you stay alive)…
This is an intentional infinite while loop made using while True
and break statement
.
Read break statement in for loop if you find this difficult to understand.
continue statement
The continue statement skips an iteration, it forces to execute the next iteration of the loop while skipping the rest of the code inside the loop for the current iteration only.
For example, you don’t want to do the 5th adventure in Amazonia for it might turn out to be life-threatening.
skip_this = 0
run_till = 0
while run_till < 10:
skip_this += 1
run_till += 1
if skip_this == 5:
continue
else:
print(f"adventure #{skip_this}")
- We first created(initialized) two variables: one for knowing which adventure to skip, and another to limit the iterations of the while loop.
- Then we limited the while loop to run 10 times. And then gave increments in the value of both the variables as the loop started.
- After that, we gave an if-else statement to skip the adventure if it is 5th or else print it(go for it).
Output:-
adventure #1
adventure #2
adventure #3
adventure #4
adventure #6
adventure #7
adventure #8
adventure #9
adventure #10
Pass statement
The pass statement is used as a placeholder for future code.
It is useful when you don’t know exactly what statements or code blocks you want to execute when the loop runs.
Example:-
new_ideas_for_adventure = " "
while new_ideas_for_adventure == " ":
pass
else clause
You can easily use the else statement with while loops just like you used it with the if statements and the for loops.
I will just show you the syntax as there are not many use cases of this until you work on big projects.
while condition:
statements
code block
else:# to be executed the condition in the while loop evaluates to False
statements
code block
If the loop is terminated with a break statement the else part would be ignored.
Hence, a while loop’s else clause runs only if :-
- no break statements are executed,
- and the condition is false.
Why python doesn’t have do while loops
The do while loops are present in a number of programming languages but, not in python.
These are the types of loops that run at least once no matter what the condition is.
We don’t have them in python because there’s no need for it.
You can use a do while loop using an infinite loop while True
and break
:
while True:
statements
code block
if condition:
break
It has the same effect as a do while loop in the other programming languages.
which is faster for loop or while loop
In one line the for loop👑 is faster than the while loop.
This is because in a for loop you don’t have to initialize a variable and then change its value inside the loop.
Whereas in a while loop you need to initialize a variable and then have to change its value inside the loop. So as to terminate the loop.
while loop comprehension
There are two major ways to write while loops in one line:-
Method#1:- When you just have one single statement to be executed.
while True: print("I love adventure")
#Don't run this it is an infinite while loop
#And if you accidentally run this code
#press ctrl c
Method#2:- When you have more than one statements to be executed.
while True: print("I love adventure"), print("But my parents worry about my safety")
#Don't run this it is an infinite while loop
#And if you accidentally run this code
#press ctrl c
However, this makes the code less readable. But, you should know them as other programmers you are working with might use this.
Conclusion
In this post, we learned about while loops. We saw they are used when the number of iterations is unknown. We then also saw an infinite while loop which keeps on running until there is any external interruption. We also saw two types of infinite while loops.
Then we compared for loops with while loops. And saw loop control statements with while loops. After that, we saw why python does not have do while loops. Finally, we concluded the post with two methods for one-liner while loops.
challenge🧗♀️
Your challenge for this post is to print the same patterns as were given in the previous post. But this time using a while loop.
pattern-1
Take an integer input n from the user. And for n print this pattern. Eg. n=5
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
pattern-2
Take an integer input n from the user. And for n print this pattern. Eg. n=5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
pattern-3
Take an integer input n from the user. And for n print this pattern. Eg. n=5
*
* *
* * *
* * * *
* * * * *
You will need to use:-
- nested while loops;
- end parameter of the print statement;
- a bit of logic;
- and lots of errors especially if you are new to programming.
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊