Hello Pythonistas welcome back.
Today we will take a quick glimpse at the Python dictionary.
Contents
What is a Python dictionary?
What comes to your mind when I say dictionary? A book with all the words and their meaning.
Well, a dictionary which is a data type in python is quite similar to this one.
It is a bunch of “key-value” pairs separated by commas ","
and enclosed in curly brackets {}
.
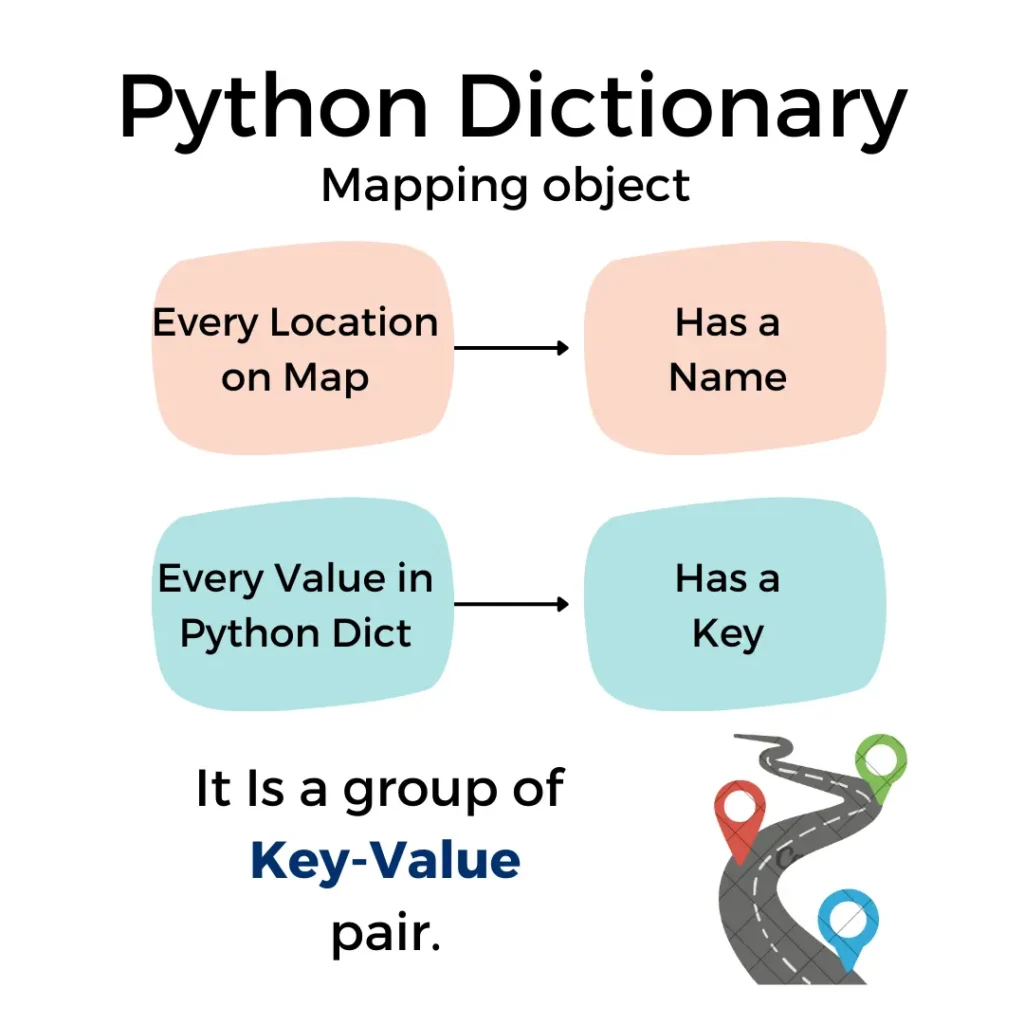
Here’s an example of one:-
where_is_what = {"apple": "top row of shelf",
"banana": "bottom row of the shelf",
"spinach": "middle row of the shelf"}
print(where_is_what)
print(type(where_is_what))
Output:-
{'apple': 'top row of shelf', 'banana': 'bottom row of the shelf', 'spinach': 'middle row of the shelf'}
<class 'dict'>
NOTE:- the key-value pair is separated by a ":"
Here apple🍎, banana🍌,
and spinach
🥦 are keys using which you can find their values. In our case their location.
We will see how you can use keys to get their values further in the post.
Some restrictions on keys
The keys in dictionaries must be immutable.
This means you cannot use a list (or any other mutable objects) as a dict’s key. Let’s try to use it and see what happens.
dict1 = {[1, 2, 3]: 1,
[4, 5, 6]: 2,
[7, 8, 9]: 3}
print(dict1)
Output:-
Traceback (most recent call last):
File "c:UsersDesktoppython-hub.compython_dictionary.py", line 1, in
dict1 = {[1, 2, 3]: 1, [4, 5, 6]: 2, [7, 8, 9]: 3}
TypeError: unhashable type: 'list'
Now you must be wondering🤔 why is it saying list unhashable it should say immutable.
What is the difference between immutable and unhashable?
Take a deep breath and don’t worry😇.
For now, you just have to take them both as the same.
Later on, when you’ll get into advanced python you’ll know✔ the difference.
Let’s move on to see ways to create a dictionary.
Two ways to create a dictionary
Using curly brackets
This is the way we just used.
You can create both an empty dictionary and a dictionary with data. See the examples below:-
empty = {}
print(empty)
where_is_what = {"apple": "top row of shelf",
"banana": "bottom row of the shelf",
"spinach": "middle row of the shelf"}
print(where_is_what)
Output:-
{}
{'apple': 'top row of shelf', 'banana': 'bottom row of the shelf', 'spinach': 'middle row of the shelf'}
Wondering why to create an empty dictionary?
Well, simple enough, say you just don’t know for now what you want to store where you want to store in your store. (Tounge 😜 twister isn’t it?)
Using the dict() function
Like we had functions for all the other data types we saw by now strings, integers, floats, lists, etc. Python dictionary too has a function.
Using the dict() function you can easily create a python dictionary.
Example:-
empty1 = dict()
print(empty1)
where_is_what = dict(apple="top row of shelf", banana= "bottom row of the shelf", spinach= "middle row of the shelf")
print(where_is_what)
Output:-
{}
{'apple': 'top row of shelf', 'banana': 'bottom row of the shelf', 'spinach': 'middle row of the shelf'}
There is a third-way dictionary comprehension to create a python dictionary. However, it includes a bit of nitty-gritty so I’ll discuss them in the next article.