tkinter place
Hey again!
So you’ve tackled grid()
in Tkinter.
Now let’s talk about its quirky cousin: place()
.
This one’s all about precision. If you’ve ever wanted to say,
“Put that button exactly here!”
then place()
is for you.
Let’s dive in. It’s gonna be fun! 🎉
Contents
What is place() in Tkinter?
Think of place()
like moving stickers on a board.
You pick the exact X and Y position—and drop your widget right there.
Perfect for:
- Artistic apps
- Custom layouts
- Games
Setting Up Your Tkinter Window
We always start with the basics:
import tkinter as tk
root = tk.Tk()
root.title("Using place() in Tkinter")
root.geometry("300x200")
root.mainloop()
Using place() to Position Widgets
Let’s add a label and a button—exactly where we want them:
label = tk.Label(root, text="Hi there!")
label.place(x=50, y=30)
button = tk.Button(root, text="Click Me")
button.place(x=100, y=80)
What’s Happening?
- The label is 50 pixels from the left and 30 from the top.
- The button is 100 pixels from the left and 80 from the top.
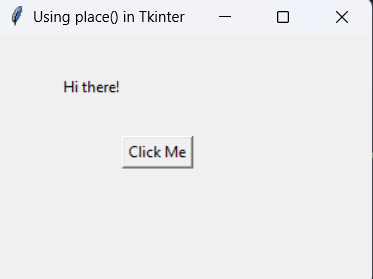
tkinter place
Widgets Don’t Move Around Like in grid()
Yup! With place()
, nothing adjusts itself.
So if the window resizes, your widgets don’t move.
That’s good and bad.
Adding Multiple Widgets with place()
Let’s create a mini-form:
import tkinter as tk
root = tk.Tk()
root.title("Using place() in Tkinter")
root.geometry("300x200")
tk.Label(root, text="Username").place(x=20, y=30)
tk.Entry(root).place(x=100, y=30)
tk.Label(root, text="Password").place(x=20, y=70)
tk.Entry(root, show="*").place(x=100, y=70)
tk.Button(root, text="Login").place(x=120, y=110)
root.mainloop()
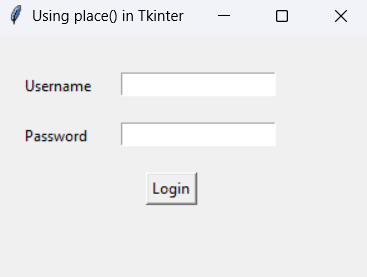
Bonus: Use Relative Positioning with relx and rely
Instead of using pixels, you can use a % of the window.
import tkinter as tk
root = tk.Tk()
root.title("Using place() in Tkinter")
root.geometry("300x200")
button = tk.Button(root, text="Centered")
button.place(relx=0.5, rely=0.5, anchor="center")
root.mainloop()
relx=0.5
puts it halfway acrossrely=0.5
puts it halfway downanchor="center"
centers it right there
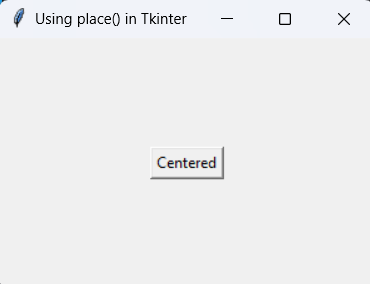
When Should You Use place()?
Here’s a quick breakdown:
Use place() when… | Avoid it when… |
---|---|
You want pixel-perfect layout | You need responsive design |
You’re making a game board | You want widgets to auto-adjust |
You need complete control | You’re building large, complex UIs |
Mixing place() with pack/grid? Nope!
Just like grid()
, don’t mix place()
with pack()
or grid()
in the same container. It messes things up.
tkinter place tkinter place tkinter place