Ever wondered what language a sentence is written in?
With just a few lines of Python code, you can create a simple app that detects the language of any sentence you input. A Language Detector Using Python and CustomTkinter
In this article, I’ll walk you through how to build a language detector using the langdetect
library and CustomTkinter
for the graphical user interface (GUI).
Let’s dive in!
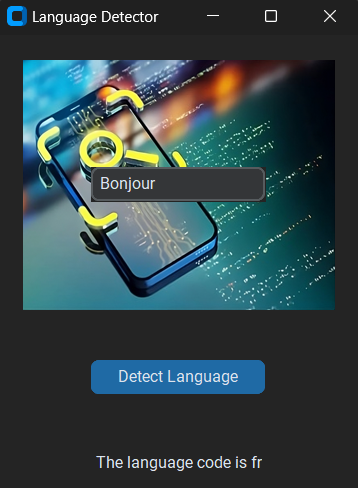
Contents
What Does the Code Do?
This project creates a small desktop application that allows you to enter a sentence and detect its language.
The language code (like ‘en’ for English, ‘fr’ for French) will be displayed as the output.
Importing Necessary Libraries
langdetect
is a Python library that helps detect the language of a given text.
CustomTkinter
is a modern and customizable version of the Tkinter library used for creating GUIs.
PIL (Python Imaging Library) is used to handle images in the application.
from langdetect import detect, DetectorFactory
from customtkinter import *
from PIL import Image
Creating the Main Application Class
We create a class LanguageDetector
that inherits from CTk
, which is the main window of our app.
The __init__
method is the constructor that initializes the window and its elements like the title, text entry, and buttons.
class LanguageDetector(CTk):
def __init__(self):
super().__init__()
self.title("Language Detector")
Adding an Image
An image is loaded and displayed using CTkImage
and CTkLabel
.
This adds a nice visual element to our app.
self.search_img = CTkImage(light_image=Image.open("search.png"), size=(250, 200))
self.il = CTkLabel(self, image=self.search_img, text="")
self.il.grid(row=0, column=0, pady=20, padx=20)
Creating a Text Entry
CTkEntry
is used to create a text box where users can enter the sentence they want to analyze.
self.text = CTkEntry(self, placeholder_text="Enter a sentence: ")
self.text.grid(row=0, column=0, padx=20, pady=20)
Adding a Button
A button labeled “Detect Language” is added. When clicked, it triggers the detect
method, which handles the language detection.
self.gettext = CTkButton(self, text="Detect Language", command=self.detect)
self.gettext.grid(row=1, column=0, pady=20)
Language Detection Logic
The detect
method retrieves the text entered by the user, detects its language using the langdetect
library, and displays the result.
def detect(self):
text = self.text.get()
DetectorFactory.seed = 0
detected_lang_code = detect(text)
label = f"The language code is {detected_lang_code}"
self.code_label = CTkLabel(self, text=label)
self.code_label.grid(row=2, column=0, padx=20, pady=20)
Running the Application
Finally, an instance of the LanguageDetector
class is created and the mainloop
method is called to keep the app running.
app = LanguageDetector()
app.mainloop()
Full Source Code: Language Detector Using Python and CustomTkinter
Click for the full code of Language Detector Using Python and CustomTkinter
from langdetect import detect, DetectorFactory
from customtkinter import *
from PIL import Image
class LanguageDetector(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("Language Detector")
# image
self.search_img = CTkImage(light_image=Image.open("search.png"),size=(250,200))
self.il = CTkLabel(self, image=self.search_img, text="")
self.il.grid(row=0, column=0, pady=20, padx=20)
# Create a CTkEntry
self.text = CTkEntry(self, placeholder_text="Enter a sentence: ")
self.text.grid(row=0, column=0, padx=20, pady=20)
# Create a CTkButton
self.gettext = CTkButton(self, text="Detect Language", command=self.detect)
self.gettext.grid(row=1, column=0, pady=20)
def detect(self):
"""Takes text as input and shows language code."""
# getting the text
text = self.text.get()
DetectorFactory.seed = 0
detected_lang_code = detect(text)
label = f"The language code is {detected_lang_code}"
# displaying the code
self.code_label = CTkLabel(self, text=label)
self.code_label.grid(row=2, column=0, padx=20, pady=20)
app = LanguageDetector()
app.mainloop()
Language Detector Using Python and CustomTkinter Language Detector Using Python and CustomTkinter Language Detector Using Python and CustomTkinter