Simply put no, integers in Python are not mutable.
age = 42
print(age) #42
age = 43
print(age) #43
Are Integers Mutable In Python?
This shows we can change the value of integers. So, why is it called unchangeable or immutable?
Look at this image:
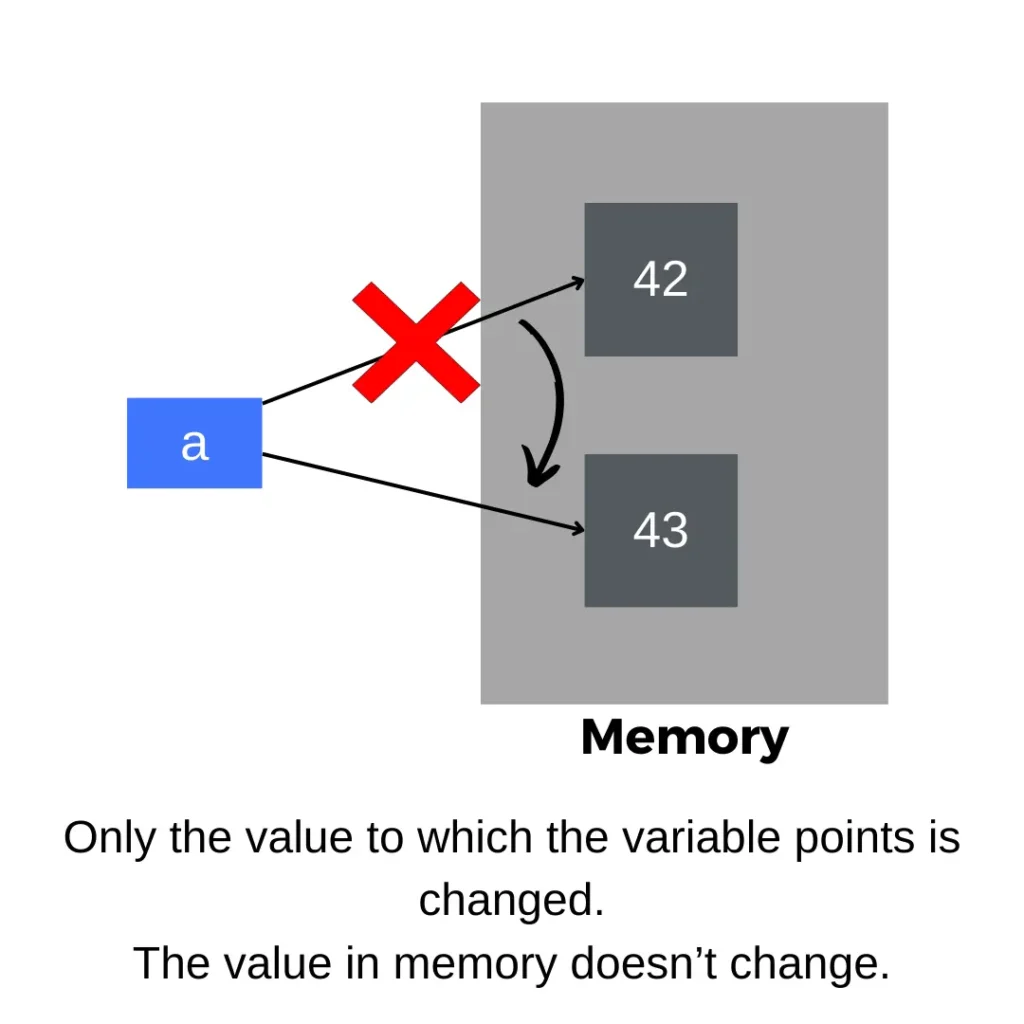
are integers mutable in python
As you can see when you change the value of a or say age from 42 to 43 it doesn’t override 42 in memory and write 43.
Or it doesn’t change the 2 with 3 and keep the 4 as it is.
It creates a new memory with value 43 and points the variable to this new address.
Contents
Why is Python number immutable?
You might ask why this modification of integer/number values is not possible.
The first reason is for efficiency. If Python already knows that the value of integers won’t be modified it can pre-allocate memory to smaller integers.
The second, is integers are used as dictionary keys. In a dictionary, you look up values using keys. Right?
Now if the key changes how would you look for its value?
It’s like you look for the word Python in your dictionary and it is changed to Pythonese for some reason.🤷♀️
There are many other reasons. But for now, I guess these are enough.
Why is int immutable and list mutable?
Someone on Sololearn asked this interesting question.
age = 42
age = 43
Are Integers Mutable In Python?
In the above code, we are not changing the value of age, we are only changing the place where the name “age” points to.
Reason given: “42” is of immutable type int. In the second line, we are creating a new object “43” of type int and assigning this to “age”. It means that when we change the ‘value’ of int, it only changes the assignment.
But in the following code:
a = [1, 2, 3, 4]
a[0] = 5
Aren’t we just changing the assignment of a[0]?
Then why do we say that lists are mutable?
Is there any other reason for calling them mutable or do they work differently (that is, lists don’t point to int objects but do something different to refer to them)?
ANSWER:
When you run this:
age = 42
print(id(age))
age = 43
print(id(age))
# OUTPUT:
# 1772080072208
# 1772080072240
The id that is the memory to which age is pointing changes.
But, when you run this code:
a = [1, 2, 3, 4]
print(id(a))
a[0] = 5
print(id(a))
# OUTPUT:
# 1772081460992
# 1772081460992
Are Integers Mutable In Python?
The id of a doesn’t change.
This means that when you modify a list the value at memory changes. But when you modify an integer value only the variable points to a new memory location.
That is why lists are mutable and integers aren’t.
are integers mutable in python