Hey there, and welcome back to python-hub.com!
Building Connect 5 Steps At A Time.
Contents
Welcome to a New Chapter on Python-Hub.com
Today, we’re kicking off something exciting, practical, and, most importantly, structured.
If you’ve been following my earlier work, like Stick To It or Heartline, you’ll know I love experimenting.
But this time, we’re diving into a well-thought-out plan, aimed at delivering consistent, impactful learning.
Here’s the idea:
- 5 steps a day (excluding weekends), taking you through building something meaningful.
- Small, manageable chunks that won’t feel overwhelming.
- Challenges along the way to level up your problem-solving.
- Projects that don’t stop at just code—we’ll aim to take them all the way to deployment with proper documentation.
This isn’t about building pre-designed projects.
Instead, you’ll see the entire journey, including the errors and debugging that come with real-world coding.
We’ll start with simple projects and gradually evolve into advanced, realistic builds.
Building Connect 5 Steps At A Time
Sound exciting?
Let’s jump into the first app in this series!
Introducing: Connect – A Photo Sharing App
Building Connect 5 Steps At A Time
We’re starting with a straightforward app called Connect, a primitive version of Instagram.
While the feature set is basic, this app will help us set the foundation for bigger ideas.
Let’s get started with Step 0!
Step 0: Setting Up Flutter
Before we dive into building, let’s ensure your development environment is ready.
- Install Flutter and all the necessary tools.
- Need help? Check out this video in English or this one in Hindi for step-by-step instructions.
Take your time with this. A solid setup saves a lot of headaches later on.
Building Connect 5 Steps At A Time
Step 1: Creating Your Flutter Project
- Create a folder on your system.
- Open VS Code, hit Command Palette, and search for “Create Flutter Project.”
- Name the project and save it in the folder you just created.
- Attach your device or enable your virtual emulator, and run the app.
You should see something like this on your screen:
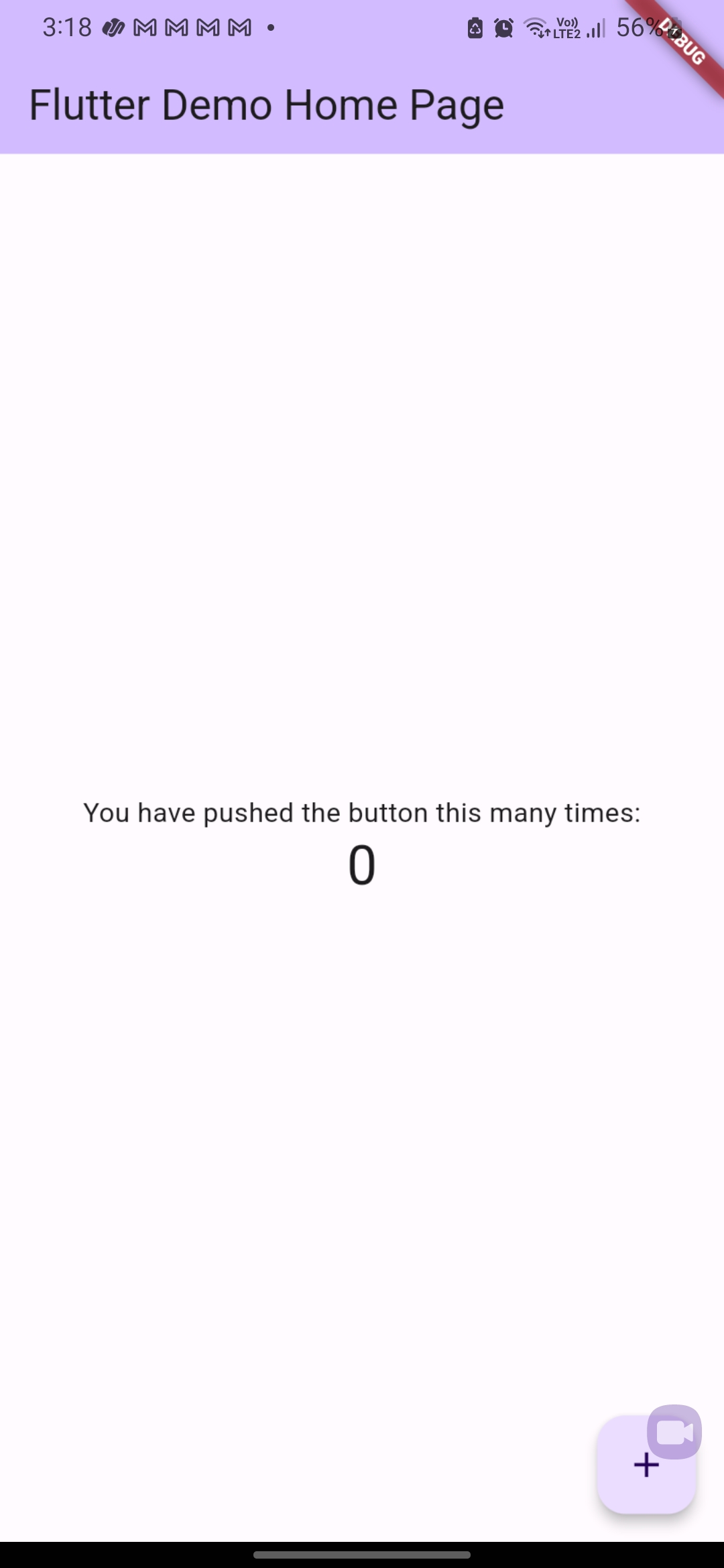
If you’ve completed Steps 0 and 1, take a break!
Step 2: Features Outline
Here’s a quick look at the features we’ll implement in Connect:
- Authentication (login, register, logout, forgot password (for people like myself 😅))
- Uploading and deleting posts
- Liking ❣️ and commenting on posts
- Following/unfollowing users
- Viewing profiles
- Search functionality
- Light/Dark Mode toggle
- Responsive design for web and mobile
Simple yet effective. Let’s move on to the real work in Step 3.
Step 3: Folder Structure
Time to clean up the auto-generated files and create a structure for clean, maintainable code:
➡️Click to view main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(),
);
}
}
Inside the lib
folder, create:
features/auth/data/
features/auth/domain/
features/auth/presentation/
Here’s how it looks:
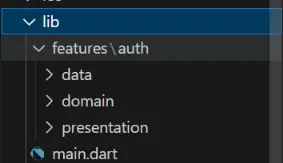
Each layer has a purpose, and we’ll build them step by step. For now, let’s start with the Domain Layer.
Step 4: Defining the User Entity
In the domain
folder, create a subfolder called entities/
and a file named app_user.dart
.
Here’s what we’ll include:
- A class with 3 properties:
userID
,email
, andname
. - Two methods:
- Convert JSON to User Class.
- Convert User Class to JSON.
➡️Click to View the Code of app_user.dart
class AppUser {
final String uid;
final String email;
final String name;
AppUser({
required this.uid,
required this.email,
required this.name
});
//convert app user to => json
Map<String, dynamic> toJson() {
return {
'uid':uid,
'email':email,
'name':name
};
}
//convert json to => app user
factory AppUser.fromJson(Map<String, dynamic> jsonUser) {
return AppUser(
uid: jsonUser['uid'],
email: jsonUser['email'],
name: jsonUser['name']
);
}
}
This sets the groundwork for efficient data handling using lightweight JSON structures.
Step 5: Building the Auth Repository
In the domain
folder, create a repos/
folder and a file called auth_repo.dart
.
This file will define an abstract class outlining our app’s authentication operations:
- Login with email and password
- Register with email and password
- Logout
- Get Current User
➡️Click to View the Code of auth_repo.dart
// Outlines the possible authentication related operations of this Connect.
import 'package:connect/features/auth/domain/entities/app_user.dart';
abstract class CAuthRepo {
Future<CAppUser?> loginWithEmailPassword(String email, String password);
Future<CAppUser?> registerWithEmailPassword(String name, String email, String password);
Future<void> logout();
Future<CAppUser?> getCurrentUser();
}
Next, move to the data
folder and create firebase_auth_repo.dart
, where we’ll implement these methods.
And that’s it for today!
What’s Next?
Tomorrow, we’ll dive into setting up Firebase for the app.
For beginners, this might have been a solid 2-day sprint (installation alone can be tricky).
For pros, this was likely a 10-minute warm-up.
Either way, the goal here is to build daily momentum while breaking down complex problems into achievable steps.
Let me know how you’re finding this format and if there’s anything I can do to make it even better for you.
Stay happy, and keep coding! See you tomorrow. 🚀
Building Connect 5 Steps At A Time Building Connect 5 Steps At A Time Building Connect 5 Steps At A Time Building Connect 5 Steps At A Time