Hello, Pythonistas Welcome Back. Today we will see how to make a fully functional modern Scroll bar in CustomTkinter.
We will use the CTkScrollbar Widget.
Contents
How Does CTkScrollbar Look?
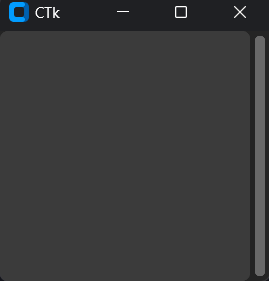
Basic Code
This is how you can make a simple Scroll Bar in CustomTkinter (or CTk).
from customtkinter import CTk, CTkTextbox, CTkScrollbar
class App(CTk):
def __init__(self):
super().__init__()
# Create a TextBox and disable its default scrollbar
self.textbox = CTkTextbox(self, activate_scrollbars=False)
self.textbox.grid(row=0, column=0)
# create CTk scrollbar and connect it to the textbox
self.scrollbar = CTkScrollbar(self, command=self.textbox.yview)
self.scrollbar.grid(row=0, column=1)
# connect textbox scroll event to CTk scrollbar
self.textbox.configure(yscrollcommand=self.scrollbar.set)
app = App()
app.mainloop()
Like any other widget in CTk, it is created and pushed to the window.
It cannot be used alone you need another widget like a text box. As the textbox has a default scrollbar we first need to disable it.
Next, we need to connect this scrollbar to the text box’s scroll event. That’s it!
It takes a compulsory argument master. This will specify where it will stay.
Why Do We Need An External Scroll Bar?
Well, it’s for those who want every nitty-gritty under their control.
It’s used to give a custom look to the scrollbar. That’s it.
All Configurations
argument | value |
---|---|
master | root, tkinter.Frame or CTkFrame |
command | function of scrollable widget to call when scrollbar is moved |
width | button width in px |
height | button height in px |
corner_radius | corner radius in px |
border_spacing | foreground space around the scrollbar in px (border) |
fg_color | forground color, tuple: (light_color, dark_color) or single color or “transparent” |
button_color | scrollbar color, tuple: (light_color, dark_color) or single color |
button_hover_color | scrollbar hover color, tuple: (light_color, dark_color) or single color |
minimum_pixel_length | minimum length of scrollbar in px |
orientation | “vertical” (standard), “horizontal” |
hover | hover effect (True/False) |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.