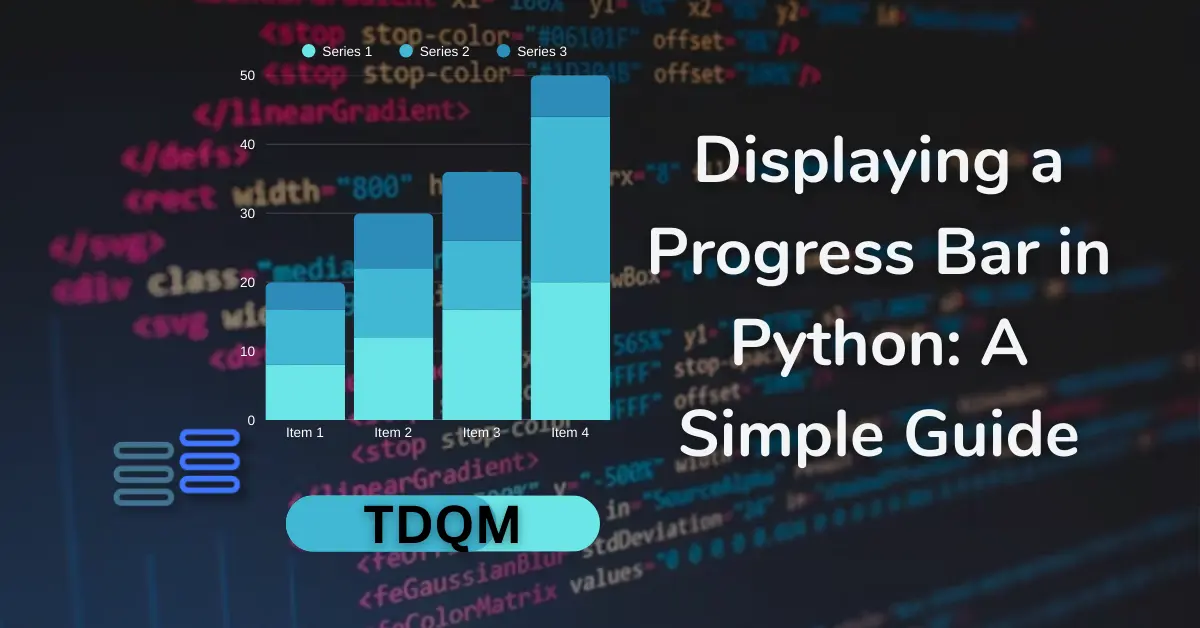
Displaying a Progress Bar in Python
Hello, Pythonistas welcome back! π
Today, weβre diving into a topic that every programmer working with long-running tasks will appreciate: progress bars in Python!
Whether you’re processing large datasets or running computationally expensive loops, adding a progress bar makes your code more user-friendly and insightful.
Displaying a Progress Bar in Python: A Simple Guide
Contents
Why Use a Progress Bar? π€
Imagine waiting for a script to finish running without knowing how long it will take. Frustrating, right? This is where progress bars come in handy! They provide real-time feedback, helping you:
β
Monitor execution progress
β
Estimate completion time
β
Debug performance bottlenecks
And the best part? Python has an awesome library called tqdm that makes adding progress bars super easy! Let’s explore how to use it.
Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide
π Installing tqdm
Before using tqdm, you need to install it. Thankfully, it’s as simple as running:
pip install tqdm
Once installed, youβre all set to add progress bars to your loops and data processing tasks.
π A Basic Example: Loop with tqdm
The simplest way to use tqdm is by wrapping it around a loop. Letβs see an example:
from tqdm import tqdm
for i in tqdm(range(10000)):
pass # Simulating work
When you run this code, youβll see a progress bar updating in real time as the loop executes. Pretty neat, right?
π How It Works:
tqdm(range(10000))
wraps around the iterable, automatically displaying a progress bar.- The bar updates as the loop progresses, showing completion percentage, iteration count, and estimated remaining time.
π Using tqdm with Pandas
If you’re working with large DataFrames, tqdm can integrate seamlessly with pandas, making data processing much more transparent.
Example: Tracking DataFrame Operations
import pandas as pd
import numpy as np
from tqdm import tqdm
# Configure tqdm for pandas
tqdm.pandas(desc="Processing DataFrame")
# Create a large random DataFrame
df = pd.DataFrame(np.random.randint(0, 100, (100000, 1000)))
# Apply a function with a progress bar
df.progress_apply(lambda x: (x + 3) ** 3)
π How It Works:
tqdm.pandas()
enables tqdm support for pandas operations.progress_apply()
replaces the usualapply()
method, displaying a progress bar while applying a function to each row/column.- The
desc
parameter lets you add a custom label to the progress bar.
This feature is a lifesaver when dealing with massive datasets, as it allows you to track processing time and identify slow operations.
Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide
π‘ Advanced Usage: tqdm in Nested Loops
For tasks involving multiple loops, you can nest tqdm progress bars to track inner and outer loop execution separately.
from tqdm import tqdm
for i in tqdm(range(5), desc="Outer Loop"):
for j in tqdm(range(100), desc="Inner Loop", leave=False):
pass
πΉ leave=False ensures the inner progress bar disappears after completion, keeping the output clean.
πΉ Each loop gets its own labeled progress bar for better readability.
π― Customizing the Progress Bar
You can customize tqdmβs appearance to match your needs!
from tqdm import tqdm
import time
for i in tqdm(range(10), desc="Custom Progress", bar_format="{l_bar}{bar} | {n_fmt}/{total_fmt} items | {elapsed}<{remaining}"):
time.sleep(0.5)
π Custom Elements:
{l_bar}{bar}
β Progress bar layout{n_fmt}/{total_fmt}
β Current iteration / Total iterations{elapsed}<{remaining}
β Elapsed time & estimated remaining time
This level of customization ensures tqdm fits your workflow perfectly!
π Why tqdm is a Game-Changer?
Hereβs why tqdm is one of the best Python libraries for tracking progress:
β Minimal setup β Just wrap your iterable!
β Lightweight β Doesn’t slow down execution.
β Highly customizable β Supports different styles, colors, and formats.
β Works with pandas, multiprocessing, and even Jupyter Notebooks!
Next time you’re running a long loop, donβt let your users (or yourself) stare at a blank screen! A simple progress bar makes your script feel interactive and professional.
Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide Displaying a Progress Bar in Python: A Simple Guide
π’ Final Thoughts
We hope this quick guide inspires you to integrate tqdm into your Python projects.
Whether you’re handling data, training models, or processing files, a simple progress bar can greatly improve the user experience.
π¬ FAQ: Frequently Asked Questions
πΉ 1. Can I use tqdm in Jupyter Notebooks?
Yes! Use tqdm.notebook
instead of tqdm
πΉ 2. Does tqdm work with multiprocessing?
Yes, but you need to use tqdmβs multiprocessing support:from multiprocessing import Pool
from tqdm import tqdm
def work(x):
return x ** 2
with Pool(4) as p:
results = list(tqdm(p.imap(work, range(1000)), total=1000))
πΉ 3. How do I remove the progress bar after completion?
Set leave=False
to remove the bar after it finishes
πΉ 4. Can I change the color of tqdm bars?
Yes! Use the colour
parameter
πΉ 5. Does tqdm slow down execution?
Not significantly. The overhead is minimal, making it perfect for tracking long-running tasks.
πΉ 6. Can I use tqdm in GUI applications?
Yes! You can integrate tqdm with Tkinter or PyQt using custom callback functions.
Thatβs a wrap! π Let me know if you have any questions or cool ways youβve used tqdm in your projects! π