Hello🙋♀️, Pythonistas welcome back.
Do you like desserts🍩?
Are you also concerned😞 about the calories they possess?
Today we will be diving🤿 into frames🖽 and radio buttons🔘 in python’s customtkinter library.
Using these we will be making a calorie-showing app📈.
For a quick tutorial on CTkFrame click here.
Contents
Previous post’s challenge’s solution
Here’s the solution to the previous post’s challenge:
# Import the customtkinter module
from customtkinter import *
# Define the Greetings_app class that inherits from the CTk class
class Greetings_app(CTk):
# Initialize the GUI window
def __init__(self):
# Call the __init__ method of the parent class (CTk)
super().__init__()
# Set the title of the window
self.title("Greeting App")
# Set the dimensions of the window
self.geometry("400x300")
# Create the label "What's your name?"
self.askn = CTkLabel(self, text="What's your name?")
# Display the label
self.askn.pack()
# Create the entry field for the name
self.ename = CTkEntry(self)
# Display the entry field with a padding of 10 pixels
self.ename.pack(pady=10)
# Create the "Show Greeting" button
self.g = CTkButton(self, text="Show Greeting", command=self.show_greeting)
# Display the button with a padding of 10 pixels
self.g.pack(pady=10)
# Create an empty label to display the greeting later
self.wish = CTkLabel(self, text="")
# Display the label
self.wish.pack()
# Define the function to show the greeting
def show_greeting(self):
# Remove the previous display of the greeting (if any)
self.wish.pack_forget()
# Create a label to display the greeting
self.wish = CTkLabel(self, text=f"Hello {self.ename.get().title()}!")
# Display the label with a padding of 10 pixels
self.wish.pack(pady=10)
# Main function to run the application
if __name__ == '__main__':
# Create an instance of the Greetings_app class
app = Greetings_app()
# Start the main event loop to display the GUI window
app.mainloop()
Here we first set up our basic structure.
Next, we created 4 widgets:-
- 2 labels,
- 1 entry widget and
- 1 button.
The first label simply asks☝️ for the user’s name. The second one is to display a greeting🙏 when the button is clicked.
The button first forgets the existing packing of the label and then redisplays it with the greeting as its text.
And the entry widget is to get the user’s name. That’s it. Easy, Right?
Frames
Have you ever worked with some real and big applications🏙️? You might have observed that they have some partitions🖽 in them.
I mean the editing🛠️ menu on one side and the buttons🔲 on the other.
Say for example the paint🖌️ app or blender(a 3d editing software).
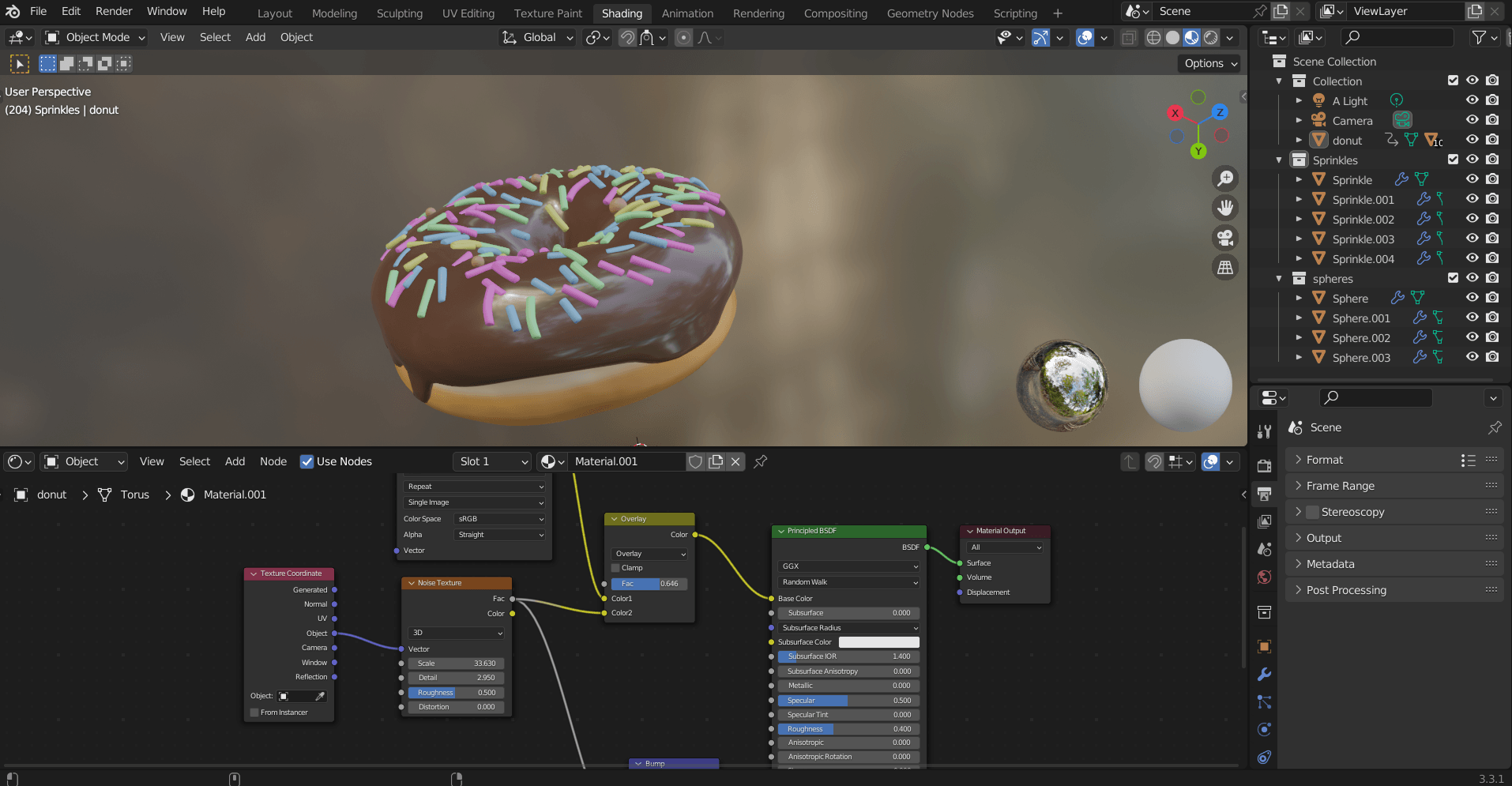
To add such partitions in customtkinter we need to use the frames widget.
Here’s how we can use it:-
from customtkinter import *
class App(CTk):
def __init__(self):
super().__init__()
self.frame = CTkFrame(self, width=400, height=200)
self.frame.grid(row=0, column=0, padx=10, pady=10)
if __name__ == "__main__":
app = App()
app.mainloop()
So, we can create a frame widget just like we do create any other widget.
Now, you can give widgets inside this frame.
Let’s give it a button that on being clicked prints "I am clicked"
:-
from customtkinter import *
class App(CTk):
def __init__(self):
super().__init__()
self.frame = CTkFrame(self, width=400, height=200)
self.frame.grid(row=0, column=0, padx=10, pady=10)
self.b = CTkButton(self.frame, text="click me", command=self.clicked)
self.b.pack(padx=50, pady=50)
def clicked(self):
print("I am clicked!")
if __name__ == "__main__":
app = App()
app.mainloop()
There are two things to be noted:
- One is that we are giving the frame as a master to the button, and
- We can use the pack function inside the frame even if we have used the grid on the frame and vice versa.
Without this frame, we can not use the pack and the grid together.
Frames in customtkinter official documentation
Radio Buttons
We all love desserts🍩 right? But, we cannot eat🍴 a lot of them at the same time.
Health👩🏻⚕️ and money💸 do not allow this.
This means you need to choose between your favorite😋 desserts every time.
What about making a calorie-showing📈 app?
Whenever you click on the dessert name, it will show you the number of calories it has.
I am not a nutritionist so the calorie numbers are google searched.
But before that, we will need to create a few 🔘🔘 radio buttons.
To create a radio button:-
self.calorie = IntVar()
self.rb = CTkRadioButton(self, text="Doughnuts", command=self.show_calories, variable=self.calorie, value=300).pack()
def show_calories(self):
print(f"I have {self.calorie.get()} calories!")
To make a useful radio button we need a customtkinter:- variable and a function.
master, text, and command are the same as a normal button.
Variable
is needed because we need to group all the options under one head. And value
is to give the value of that specific option(or button)
To get the value inside a customtkinter button we need to use the get
method.
And to define a customtkinter integer variable we need to use IntVar
. For a string variable we would use StrVar
.
Now, making all the buttons one after another like this would be long and not pythonic so, we will do it using: lists, tuples, and a for-loop.
#This list stores desserts and their calories in a tuple form
self.D = [
("Doughnuts", 300),
("Pumpkin Pie", 243),
("Fudge", 411),
("Brownies", 466),
("Cheesecake", 321),
]
# create a IntVar to hold the value(calorie) of the radio buttons(desserts)
self.selected = IntVar()
# loop over the items in the list self.D
for dessert, calories in self.D:
self.rb = CTkRadioButton(self, text=dessert, variable=self.selected, value=calories, command=self.show_calories)
self.rb.pack()
The dessert 🍮 variable will have the name of the dessert.
And the calories 🔢 will have the number of calories it has.
We gave dessert as text to be displayed, and calories as the value of our cutomtkinter variable.
We stored it all under a common variable as these belong to the same group🎗️.
Finally, we have given show_calories
method that prints the no. of calories.
Now, we can update our show_calories
method, it will display the calories on a label.
We would need a label too for this purpose:
# Create an empty label to display the calories later
self.show = CTkLabel(self, text="")
# Display the label
self.show.pack()
def show_calories(self):
# Remove the previous display of the calories (if any)
self.show.pack_forget()
# Create a label to display the calories
self.show = CTkLabel(self, text=f"I have {self.selected.get()} calories!")
# Display the label with a padding of 10 pixels
self.show.pack(pady=10)
Here’s the full code if you got lost:
from customtkinter import *
class App(CTk):
def __init__(self):
super().__init__()
self.title("Desserts and Calories")
self.geometry("400x300")
self.D = [
("Doughnuts", 300),
("Pumpkin Pie", 243),
("Fudge", 411),
("Brownies", 466),
("Cheesecake", 321),
]
self.selected = IntVar()
for dessert, calories in self.D:
self.rb = CTkRadioButton(self, text=dessert, variable=self.selected, value=calories, command=self.show_calories)
self.rb.pack(pady=10)
self.show = CTkLabel(self, text="")
self.show.pack()
def show_calories(self):
self.show.pack_forget()
self.show = CTkLabel(self, text=f"I have {self.selected.get()} calories!")
self.show.pack(pady=10)
if __name__ == "__main__":
app = App()
app.mainloop()
Radio Buttons in customtkinter official documentation
Conclusion
In this post, we saw frames and radio buttons in customtkinter.
Along with this, we made a calorie-showing📈 app.
Now, we will be continuing with OOPs concepts in python, in the next post. As I don’t want this python tutorial to be a GUI tutorial for you all.
We will be having GUI tutorials in the GUI tab of python-hub.com.
You can continue with that if you wish to do so.
Challenge 🧗♀️
So the challenge is to show the money required along with the calorie count.
Here’s a hint:
Use StringVar and then use it as a list.
I’ll see you in the next post with one of the base principles of OOP.
Till then solve the challenge, have a great time, and keep smiling😊. Bye Bye👋