Hello Pythonistas, welcome back. Today we will see how to use the CTkEntry widget in customtkinter.
CTkEntry is used to take user input in Customtkinter.
Contents
How Does CTkEntry Look?
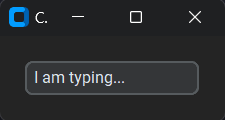
Basic Code
This is how you can make a simple entry widget in CustomTkinter (or CTk).
from customtkinter import CTk, CTkEntry, CTkButton
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("CTkEntry Example")
# Create a CTkEntry
self.entry = CTkEntry(self)
self.entry.pack(padx=20, pady=20)
app = App()
app.mainloop()
Like any other widget in CTk, first, it is created, and then it is pushed to the window.
But, this one is different as it allows the program to take input from the user. The user can type in anything.
This user input can be accessed using the get()
method. It returns the data in the form of a string.
It takes a compulsory argument master. This will specify where it will stay.
A Sample Modern Input Widget
Now we will make a simple facility that takes the user’s name and greets him/her.
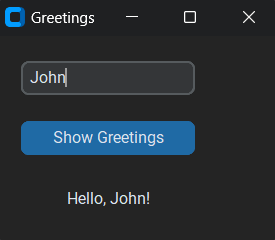
We would need to:
- Create an Entry widget and a button.
- Pack them on the window using the grid method.
- Now create a function. This function:
- Would take up the text in the entry widget.
- Then, will add hello before it.
- Finally, it will put this data in a label.
- Lastly, connect the button to this function.
Simple that’s all!
from customtkinter import CTk, CTkEntry, CTkButton, CTkLabel
# Create a custom application class "App" that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
# Call the constructor of the parent class (CTk) using super()
super().__init__()
self.title("Greetings")
# Create a CTkEntry
self.name = CTkEntry(self, placeholder_text="Enter your name: ")
self.name.grid(row=0, column=0, padx=20, pady=20)
# Create a CTkButton
self.getnm = CTkButton(self, text="Show Greetings", command=self.get_name)
self.getnm.grid(row=1, column=0, pady=20)
def get_name(self):
# getting the name
name = self.name.get()
# adding greeting before the name
greeting = f"Hello, {name}!"
# displaying the greeting
self.greet_label = CTkLabel(self, text=greeting)
self.greet_label.grid(row=2, column=0, padx=20, pady=20)
app = App()
app.mainloop()
The placeholder_text
argument is to display the hint. It’s like the prompt in the input function.
If you want to know how to make a learning diary check this out!
All Configurations
argument | value |
---|---|
master | root, tkinter.Frame or CTkFrame |
placeholder_text | hint on the entry input (disappears when selected), default None |
placeholder_text_color | tuple: (light_color, dark_color) or single color |
width | entry widget’s width in px |
height | entry widget’s height in px |
corner_radius | corner widget’s radius in px |
fg_color | foreground color, tuple: (light_color, dark_color) or single color or “transparent” |
text_color | type in text’s color, tuple: (light_color, dark_color) or single color |
I am not going to give any challenges at the end of such articles as they are just for your quick reference.
Note I haven’t included all the methods and attributes. You can always get that on the documentation.