Hello, Pythonistas Welcome Back. Today we will see how to add an image using CustomTkinter.
We will use the CTkImage and CTkLabel Widgets.
Contents
How Does It Look?
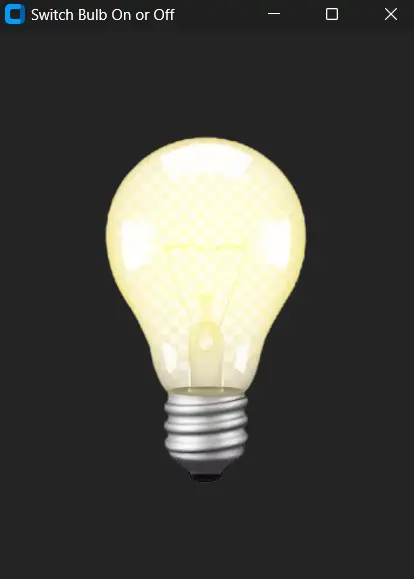
Basic Code To Add An Image Using Customtkinter
Now to add an image using Customtkinter we need 3 things:
- CTkImage
- CTkLabel
- Image module of Python.
First, we need to open an image using the Image module.
Next, we need to pass this image to the CTkImage class.
Lastly, we need to pass this image to the CTkLabel class.
# Importing necessary module
from customtkinter import CTk, CTkImage, CTkLabel
from PIL import Image
# Creating an App class that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
super().__init__() # Calling the constructor of the superclass (CTk)
self.title("Switch Bulb On or Off")
self.on_img = CTkImage(light_image=Image.open("images\\bulb_on.png"),size=(300,400))
self.il = CTkLabel(self, image=self.on_img, text="")
self.il.grid(row=0, column=0, pady=20, padx=20)
app = App()
app.mainloop()
Image resources:
A Sample Modern CTkImage
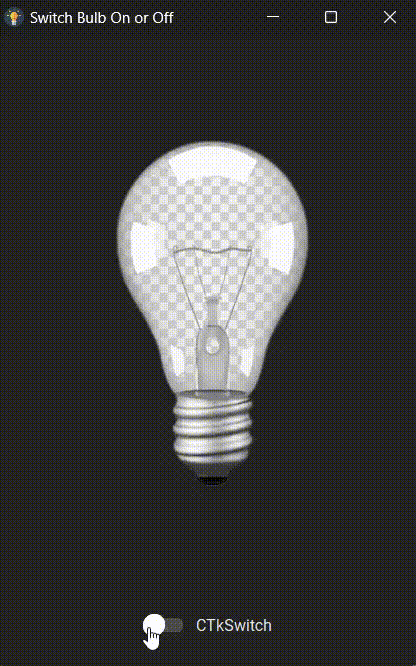
For an explanation click here.
For Code Click Here
# Importing necessary module
from customtkinter import CTk, CTkSwitch, StringVar, CTkImage, CTkLabel
from PIL import Image
# Creating an App class that inherits from CTk (Custom Tkinter)
class App(CTk):
def __init__(self):
super().__init__() # Calling the constructor of the superclass (CTk)
self.title("Switch Bulb On or Off")
# Setting window icon
self.iconbitmap("images\\light.ico")
# Bulb on and off images
self.on_img = CTkImage(light_image=Image.open("images\\bulb_on.png"),size=(300,400))
self.off_img = CTkImage(light_image=Image.open("images\\bulb_off.png"),size=(300,400))
# Creating a CTkSwitch instance within the window
# Along with a variable to keep track of its value
self.switch_var = StringVar(value="on")
self.se = CTkSwitch(self, command=self.switch_event,
variable=self.switch_var,
onvalue="on", offvalue="off",
progress_color="yellow")
self.se.grid(row=1, column=0, padx=20, pady=20)
# method to change the image based on the current value of the switch
def switch_event(self):
get = self.switch_var.get()
if get=="on":
self.il = CTkLabel(self, image=self.on_img, text="")
self.il.grid(row=0, column=0, pady=20, padx=20)
elif get == "off":
self.il = CTkLabel(self, image=self.off_img, text="")
self.il.grid(row=0, column=0, pady=20, padx=20)
app = App()
app.mainloop()