Hello Pythonistas welcome back.
For this website, I constantly need to upscale the images I have.
But going to other websites and upscaling my images raises a question about that data’s privacy.
Are you too tired of such blurry images??
Here I’ll show you how to upscale your images using Python quickly.
Contents
Installation
Why installation?
Well, that’s because it can be quite tricky for beginners.
You first need to install the latest versions of opencv and opencv-contrib.
pip install opencv-python
pip install opencv-contrib-python
Check if dnn_superres
is there:-
import cv2
# Check if dnn_superres is available
if hasattr(cv2, 'dnn_superres'):
print("dnn_superres module is available.")
else:
print("dnn_superres module is NOT available.")
Then update if dnn_superres
is not found.
pip install --upgrade opencv-contrib-python
Next, you need to choose a model and download it.
Choose a model from this link and download it.
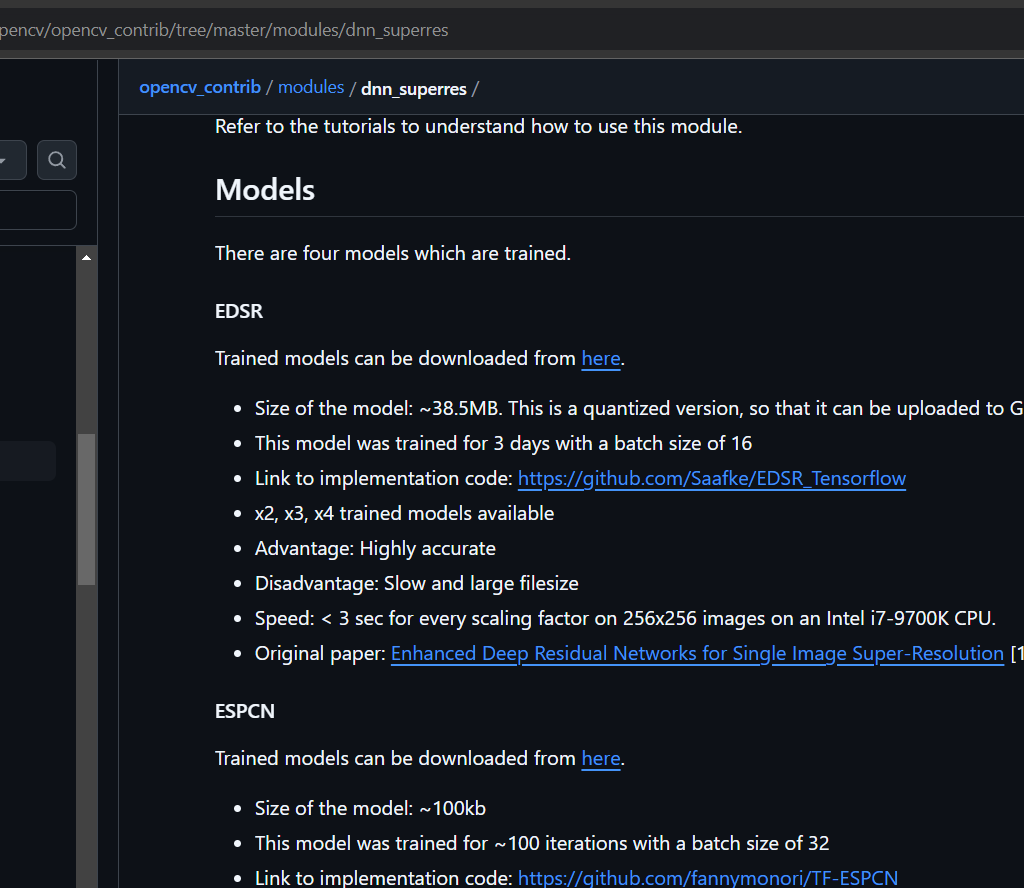
If you tried it directly you might have encountered a few errors like:
sr = cv2.dnn_superres.DnnSuperResImpl_create()
AttributeError: module 'cv2' has no attribute 'dnn_superres'
sr.readModel(model_path)
cv2.error: OpenCV(4.10.0) D:\a\opencv-python\opencv-python\opencv\modules\dnn\src\caffe\caffe_io.cpp:1138: error: (-2:Unspecified error) FAILED: fs.is_open(). Can't open "EDSR_x4.pb" in function 'cv::dnn::ReadProtoFromBinaryFile'
And I know it’s quite frustrating when you need something done quickly. That’s why this section.
Full Source Code: Upscale Your Images Using Python
import cv2
# Load the pre-trained super-resolution model
sr = cv2.dnn_superres.DnnSuperResImpl_create()
# Read the image
print("Reading image")
image = cv2.imread('input_image.jpg')
# Read the model
print("Reading Model")
model_path = "EDSR_x4.pb" # You can choose from various models: EDSR, ESPCN, FSRCNN, etc.
#You would need to install the model.
sr.readModel(model_path)
# Set the model and scale to be used
print("Setting Model scale")
sr.setModel("edsr", 4) # EDSR model with a scaling factor of 4
# Upscale the image
print("upscalling the image")
upscaled_image = sr.upsample(image)
# Save the output
print("scaling upscaled image")
cv2.imwrite('upscaled_image.jpg', upscaled_image)