Learn how to use the Tkinter Scrollbar in Python.
This beginner-friendly guide walks you through step-by-step with code examples and explanations.
Contents
Tkinter Scrollbar Tkinter Scrollbar
What is a Scrollbar in Tkinter?
Scrollbars in Tkinter are those little bars that help you scroll when content overflows.
Imagine you have a huge list or a long text area.
If it doesn’t fit in the window, you need a way to see the rest.
That’s where scrollbars come in.
They don’t belong inside widgets like Text
or Listbox
.
Instead, they are separate widgets that you connect to scrollable ones.
Basics of Tkinter Scrollbar Widget
To make a scrollbar in Tkinter, you use ttk.Scrollbar
.
It’s simple and modern-looking.
The basic syntax looks like this:
ttk.Scrollbar(master=None, **kw)
Here’s what it means:
master
: Where the scrollbar goes (like a Frame or root window).**kw
: Extra options, like direction (orient
), action (command
), style, and more.
Common values:
orient=tk.VERTICAL
ortk.HORIZONTAL
command=some_widget.yview
orxview
Tkinter Scrollbar
How to Create a Vertical Scrollbar
Let’s create one step by step.
v_scrollbar = ttk.Scrollbar(
scrollable_widget,
orient=tk.VERTICAL,
command=scrollable_widget.yview
)
What’s happening here?
scrollable_widget
: This could be aText
,Listbox
, orCanvas
.orient
: We pickedVERTICAL
for up-down scrolling.command
: This tells the scrollbar what to control.
Now, we also need the widget to tell the scrollbar what’s visible.
We do that like this:
scrollable_widget['yscrollcommand'] = scrollbar.set
Now both the scrollbar and widget are in sync.
Making Widgets Scrollable with Scrollbar
Let’s break it down.
Link Scrollbar → Widget
Use command=widget.yview
Link Widget → Scrollbar
Use yscrollcommand=scrollbar.set
Both sides need to talk to each other for a smooth scrolling.
Adding a Scrollbar to a Text Widget With Example
Here’s a working example:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
root.title("Tkinter Scrollbar")
frame = ttk.Frame(root)
frame.pack(padx=10, pady=10, fill=tk.BOTH, expand=True)
v_scrollbar = ttk.Scrollbar(frame)
v_scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
text = tk.Text(frame, height=8)
text.pack(side=tk.LEFT, expand=True, fill=tk.BOTH)
text['yscrollcommand'] = v_scrollbar.set
v_scrollbar.config(command=text.yview)
text.insert(tk.END, "\n" * 20)
root.mainloop()
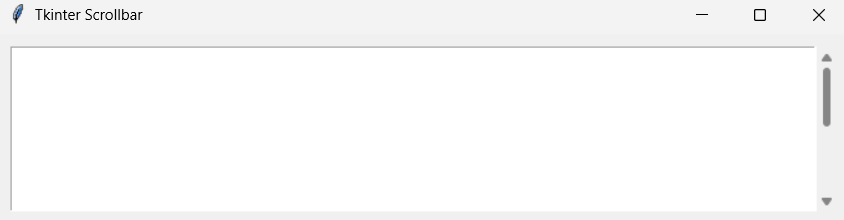
How it works:
- We put both the
Text
andScrollbar
in aFrame
. - The scrollbar is packed to the right, filling vertically.
- The text widget is on the left and expandable.
- We connect both using
yscrollcommand
andcommand
.
Now, when you run it, the scrollbar works perfectly.
Horizontal Scrollbars
Sometimes, your content stretches sideways instead of down. Maybe it’s a wide table, long line of code, or big image. That’s where horizontal scrollbars shine.
How to Add a Horizontal Scrollbar
Let’s tweak our earlier example to include one:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
root.title("Horizontal Scrollbar Example")
frame = ttk.Frame(root)
frame.pack(padx=10, pady=10, fill=tk.BOTH, expand=True)
# Text widget
text = tk.Text(frame, wrap="none", height=8, width=40)
text.pack(side=tk.TOP, fill=tk.BOTH, expand=True)
# Horizontal scrollbar
h_scrollbar = ttk.Scrollbar(frame, orient=tk.HORIZONTAL, command=text.xview)
h_scrollbar.pack(side=tk.BOTTOM, fill=tk.X)
text.config(xscrollcommand=h_scrollbar.set)
# Add sample long line
text.insert(tk.END, "This is a very long line of text that will need horizontal scrolling. " * 5)
root.mainloop()
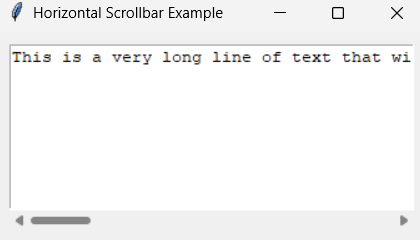
What’s New?
- We added
wrap="none"
to theText
widget. This disables auto line wrapping so the line can scroll horizontally. - We packed the horizontal scrollbar at the bottom (
side=tk.BOTTOM
) and filled it horizontally withfill=tk.X
. - We connected
xview
andxscrollcommand
just like we did with vertical scrolling.
Now you can scroll left and right easily.
Conclusion
Using scrollbars in Tkinter might look a little tricky at first—but once you get the hang of it, it’s super simple.
Let’s recap the key steps:
- Create a
Scrollbar
widget. - Set orientation: vertical or horizontal.
- Link the widget to the scrollbar using
command
andyscrollcommand
orxscrollcommand
. - Pack them properly inside a
Frame
.
With scrollbars, your GUI apps become way more user-friendly. Whether you’re building a text editor, a file browser, or just displaying a lot of data—scrollbars will make your layout clean and accessible.
If you’re feeling adventurous, try combining both horizontal and vertical scrollbars with a Canvas
widget. It gives you full control over scrolling content in any direction.
That’s it! You now have a strong foundation for using the Tkinter Scrollbar widget like a pro.