What comes to your mind🧠 when I say inheritance?
- Darwin’s Pangenesis,
- Your parent’s property,🤑😅
- Or some majestic fortune🤩 that may belong to you. (Treasure)
In real life, we have a parent-child relationship🤝. Whatever belongs to your parents belongs to you their genes, property, fortune, or even misfortune.
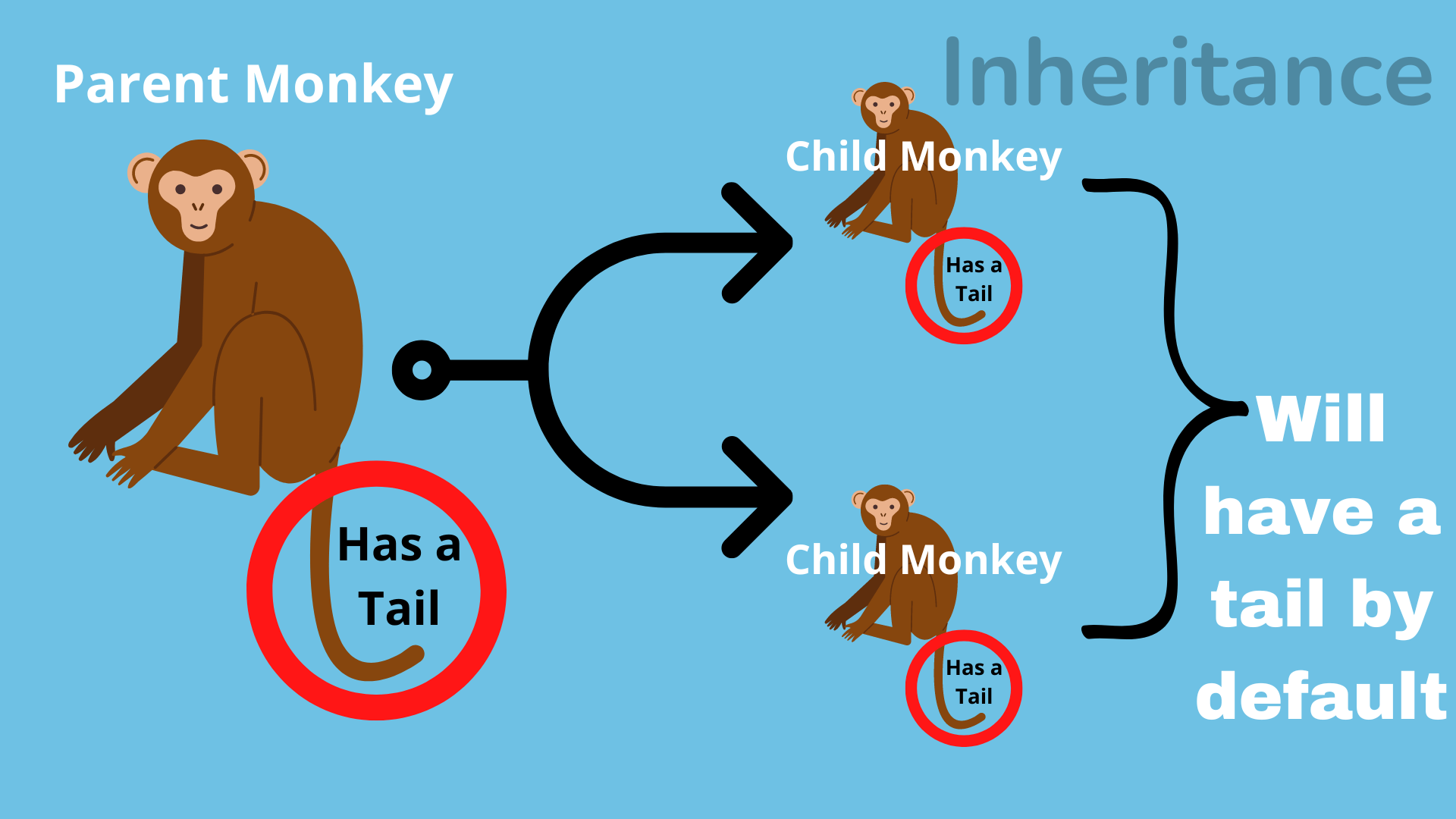
By this example, I am in no way calling you a monkey🐒.🙅♀️
In programming, we implement this concept using classes.
Let’s explore how.
We will be taking two ✌️ imaginary classes:
- Person 🧑 and
- Superhero 🦸♀️
Contents
Before we dive any deeper🏊♀️ into inheritance here’s the solution to the previous post’s challenge.
Previous post’s challenge’s solution
from abc import ABC, abstractmethod
class Shape(ABC):
def __init__(self):
self.area = 0
self.perimeter = 0
@abstractmethod
def calc_area(self):
pass
@abstractmethod
def calc_perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, width, height, units):
self.width = width
self.height = height
self.units = units
def calc_perimeter(self):
self.perimeter = 2*(self.width + self.height)
print(f"Perimeter of this rectangle is {self.perimeter} {self.units}.")
return self.perimeter
def calc_area(self):
self.area = self.width * self.height
print(f"Area of this rectangle is {self.area} {self.units} sq.")
return self.area
rect = Rectangle(10, 20, "cm")
a = rect.calc_area()
print(a)
p = rect.calc_perimeter()
print(p)
Output:
Area of this rectangle is 200 cm sq.
200
Perimeter of this rectangle is 60 cm.
60
As said we have made a Shape class. It is an abstract class with two abstract methods:
- calc_area()
- calc_perimeter()
The Rectangle class takes methods and variables from the Shape class. (Inherits)
The Rectangle class takes height, width, and units of measurement when an object is created.
These two methods in the Rectangle class simply take calculate area/perimeter print it and return it.
We then created an instance rect, with a width = 10, a height = 20, and cm as its units.
This was a good practice of abstraction along with that was a closer introduction to inheritance.
Now time for inheritance…
What is inheritance?
In simple words, inheritance defines a new class using an existing one.
The class which is defined is called derived/child/subclass.
And the class from which the class is defined is called the base/parent/super class.(The existing one)
Syntax of Inheritance:
class SuperClass:
Body of super class
class SubClass(SuperClass):
Body of sub class
The SubClass will have all the variables and methods of the SuperClass even the __init__ of it along with its own features.
Example of Inheritance:
Let’s assume there are two ✌️ types of people in this world:
- Normal People 🧑 and
- Super Heroes 🦸
What can normal people do? Walk🚶♀️, talk, etc.
What can they have? Name, age, gender, etc.
Let’s create a person class with these methods and attributes:
class Person:
def __init__(self, name, age, gender):
self.name = name
self.age = age
self.gender = gender
def speak(self):
print("Hello, my name is", self.name)
def walk(self):
print(self.name, "is walking")
Now, all the superheroes can do it.
Like even if they have Mjölnir or Cosmic Iron Patriot armor. They can walk.
So, our Person class can be used to create an Iron man class. As practically he is a human too.
Let’s create an iron_man class which will be derived from Person class:
class iron_man(Person):
def __init__(self):
print("\"Cosmic Iron Patriot armor\" activated...")
def genius(self):
print("I am a skilled engineer, inventor, and problem solver.")
def humor(self):
print("I am known for my witty and sarcastic sense of humor. ;-) ")
i1 = iron_man()
(Apologies if I mentioned anything wrong above I am not an avenger fan🤥😅)
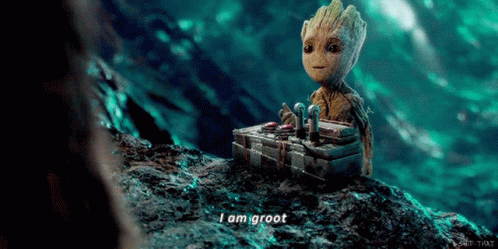
Here, I have just shown how to make a new class that inherits from an existing class.
We will be discussing details but, before that let’s see some real examples, of where inheritance is used.
Where is Inheritance Used
We have used this concept more than thrice already in this tutorial itself.
The latest was when we were discussing abstract methods here.👈
Before that when we started off with GUI using the customtkinter library.👈
And then, when we made the calculator app and the reminisce app.👈
If these real working examples are not enough then type the basic structure of making a GUI application:
from customtkinter import *
class App(CTk):
pass
Now if you are on pycharm or VScode then, press the ctrl key and click on CTk. You’ll see this code in a new file:
class CTk(tkinter.Tk, CTkAppearanceModeBaseClass, CTkScalingBaseClass):
"""
Main app window with dark titlebar on Windows and macOS.
For detailed information check out the documentation.
"""
You’ll see these lines after a few import statements.
What this shows is that while the code for this external library was being written the author used the concept of inheritance.
I hope this makes the use of inheritance clear to you.
Now, let’s see its types.
Types of inheritance
There are mainly 3 types of inheritance in python.
- Single level
- Multi-level
- Multiple
Single level Inheritance
The type of inheritance we just discussed above is single-level inheritance.
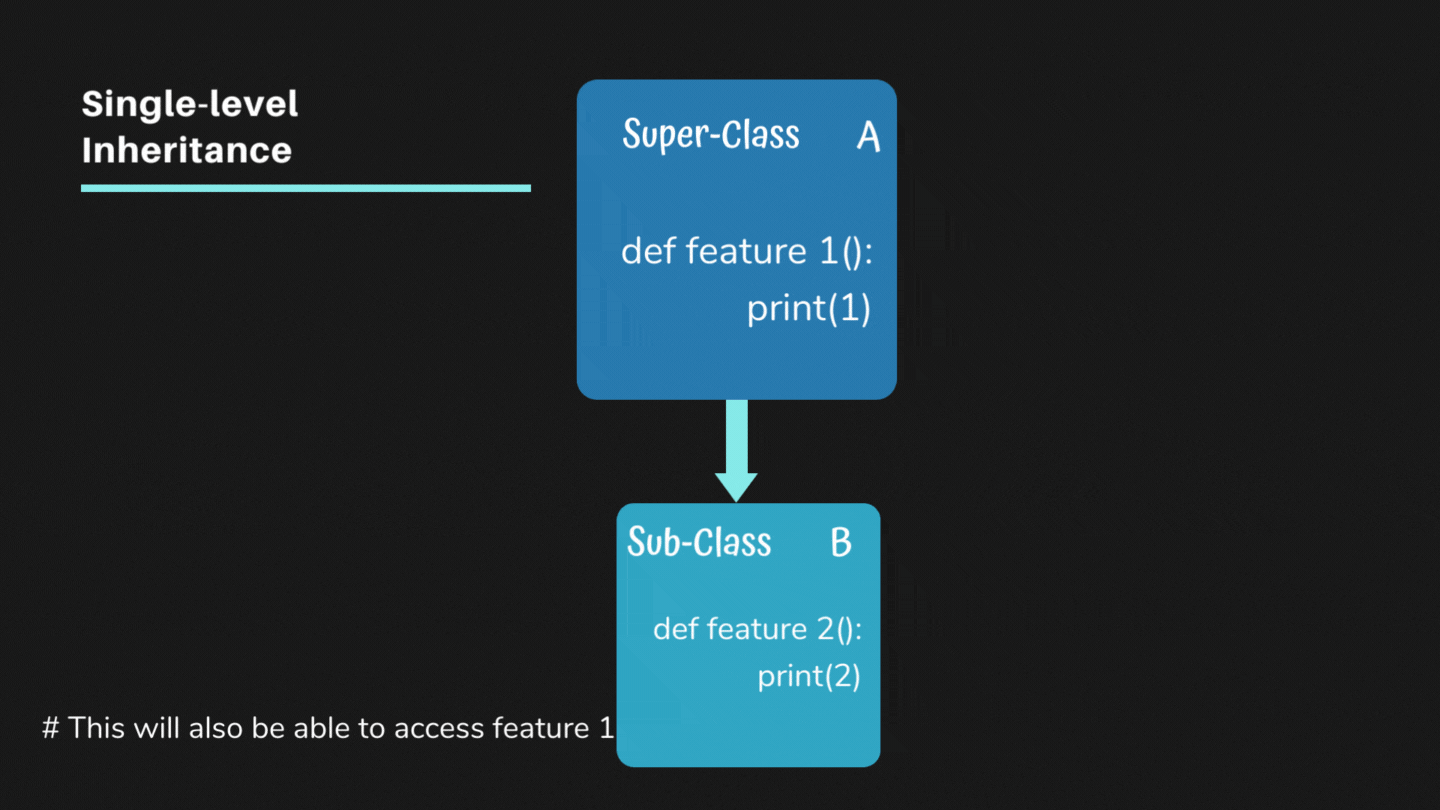
This is just a fancy ✨ way of saying that one class is inheriting another class but just one. Like B is inheriting just A.
Here’s another easy example for clarity.
class A:
"""This is our super class A"""
def feature1(self):
"""This is a method inside of our super class."""
print("Feature 1 working...")
class B(A):
"""This is our sub class B"""
def feature2(self):
"""This is a method inside of our sub class."""
print("Feature 2 working...")
b1 = B() #b1 is an object of class B
b1.feature1() #b1 can access methods of class A
b1.feature2() #and can obviously access methods of class B
#This is a very simple example of single-level inheritance
So, we have two ✌ classes A and B.
B is inheriting ⬅ A.
This is an example of single-level ☝ inheritance.
An object of class B will be able to access 👍 all the features and attributes of class A.
B is sub-class and A is super-class.
Now, Let’s see what is multi-level inheritance.
Multi-level Inheritance
We all have parents and grandparents. I know that’s obvious.
Consider you and your parents are single children and your parents or grandparents aren’t planning to donate anything they have.
This means that whatever belongs to your grandparents belongs to your parents. And whatever belongs to your parents belongs to you.
Now how will you represent cases like this using single inheritance?
The simple answer is, it’s not possible that way you need to use something we call Multi-level inheritance.
In Multi-level inheritance:
- One class inherits another
- And that class is inherited by the third class
- And so on…
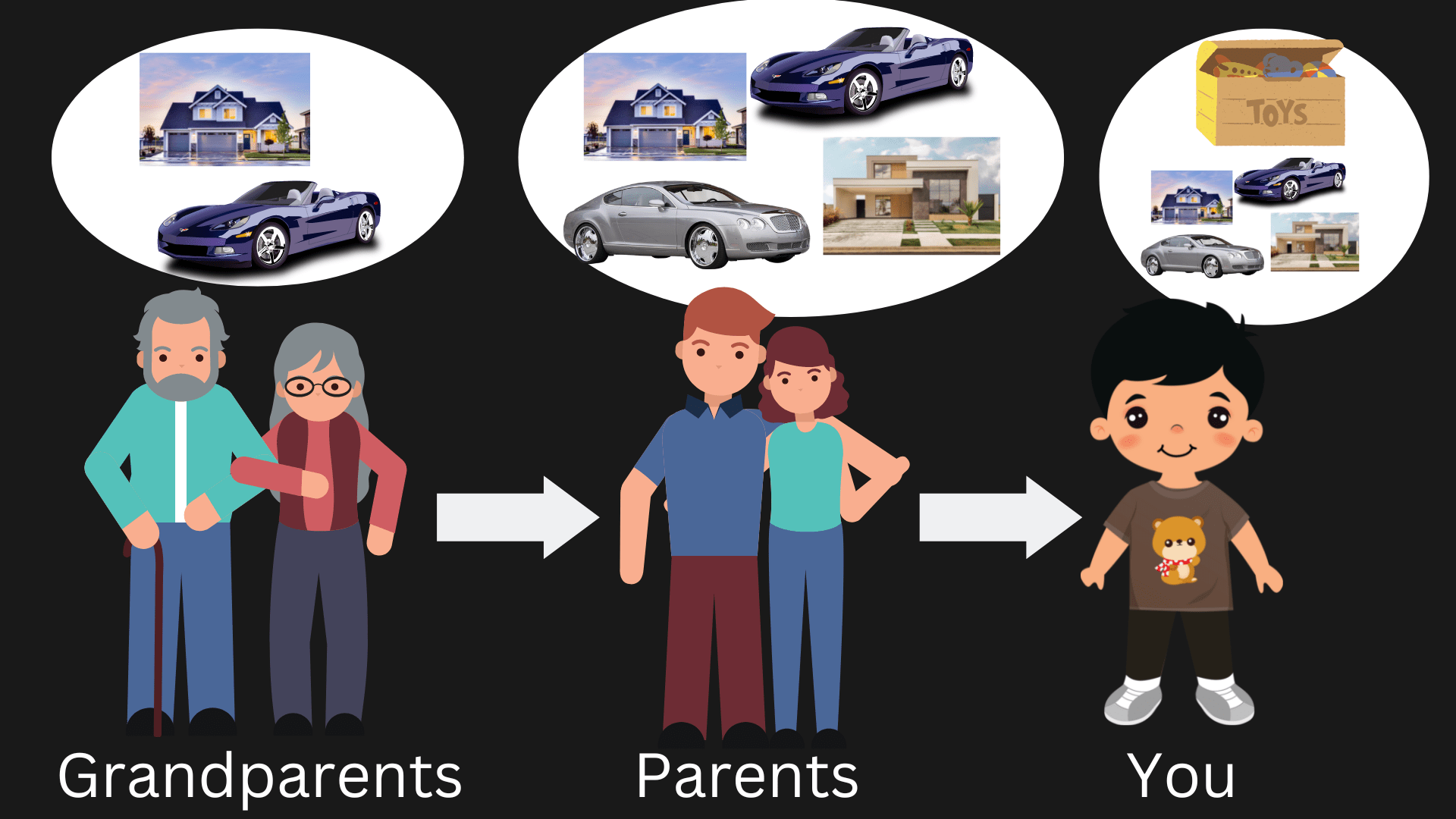
An Example of Multi-level inheritance
Meaning we will have two super-classes and two sub-classes.
Like in your case we have two parents and two children.
The code will make it more clear to you:
class Grandparent:
old_car = 100_000
old_house = 1_000_000
class Parent(Grandparent):
new_car = 100_000
new_house = 1_000_000
class You(Parent):
latest_car = 100_000
latest_house = 1_000_000
you = You()
#You can access all that your parents have
print(you.new_car)
print(you.new_house, "\n")
#You can even access all your grandparents have
print(you.old_car)
print(you.old_house)
#You can obviously access all you have
Output:-
100000
1000000
100000
1000000
Here,
- Your parents are inheriting from your grandparents meaning they can access all your grandparents have.
- You are inheriting from your parents meaning you can access all your parents have. This includes all your grandparents have, too.
This is an easy peasy example of multi-level inheritance.
Now, Let’s see what is multiple inheritance.
Multiple Inheritance
OK so now let’s just talk about parent-child relations.
I mean you and your mom and dad.
Maybe you have got hair like that of your mom and face structure like that of your dad. This means you have inherited two different characteristics from two different people.
However, they haven’t inherited anything from each other they are two different entities. In terms of inheritance, we call it “multiple inheritance”.
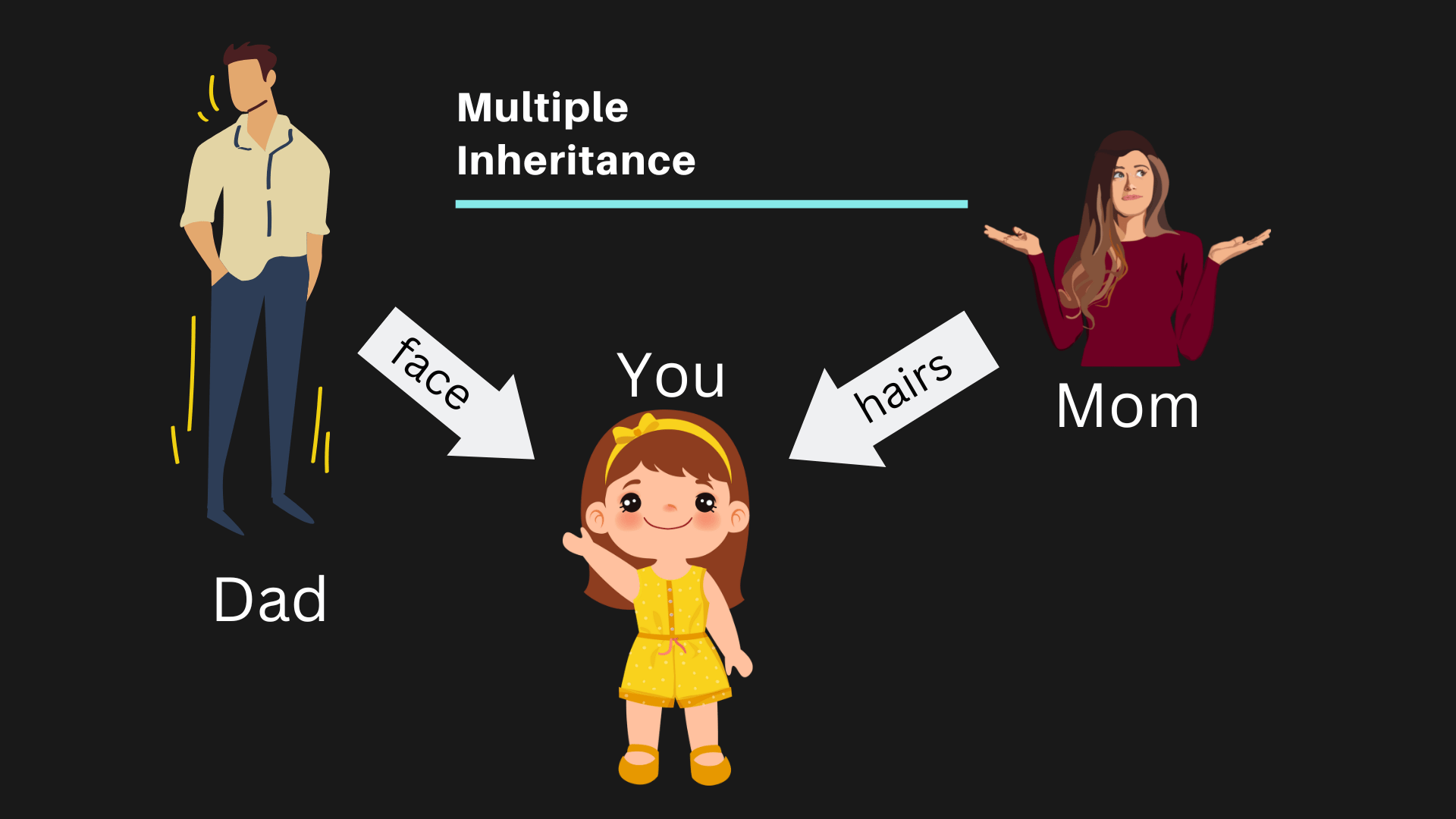
Under this one class inherits from more than one class.
See the example below:
class Mom:
hairs = ["bouncy", "fluffy", "delicate", "fine"]
class Dad:
face_structure = "OVAL"
class You(Mom, Dad):
pass
y = You()
print(y.hairs)
print(y.face_structure)
Output:-
['bouncy', 'fluffy', 'delicate', 'fine']
OVAL
pass written inside the You class indicates that there is nothing inside this class.
This was a handy example of multiple inheritance.
Let’s move on to see how the constructor and other methods and variables work in inheritance.
Constructor in inheritance
Ok so by now you know that whenever we want to use features of an existing class instead of copy-pasting those features we will say that the new class inherits this class.
Let’s now look 👀 at how the constructor (__init__) works in inheritance.
Suppose that in class A we created here, we are defining an __init__ method that simply prints “In A init”.
class A:
"""This is our super class A"""
def __init__(self):
"""Constructor. Prints In A init"""
print("In A init")
def feature1(self):
"""This is a method inside of our super class."""
print("Feature 1 working...")
class B(A):
"""This is our sub class B"""
def feature2(self):
"""This is a method inside of our sub class."""
print("Feature 2 working...")
b1 = B()
Output:-
In A init
“Whenever the sub-class does not have its init, the init of the super-class is called. Like in our example above.”
But what happens when both of them have an init method?🤔
class A:
"""This is our super class A"""
def __init__(self):
"""Constructor. Prints In A init"""
print("In A init")
def feature1(self):
"""This is a method inside of our super class."""
print("Feature 1 working...")
class B(A):
"""This is our sub class B"""
def __init__(self):
"""Constructor. Prints In B init"""
print("In B init")
def feature2(self):
"""This is a method inside of our sub class."""
print("Feature 2 working...")
b1 = B()
Output:-
In B init
In this case, the constructor of the sub-class is called.
But what if in such a case you want to access both init methods?🤷♀️
The super() method will help you here.💁♀️ Let’s see how.
super()
The super method is always used inside of the sub-class. In our case inside B’s init.
class B(A):
"""This is our sub class B"""
def __init__(self):
"""Constructor. Prints In A init and
Prints In B init"""
super().__init__()
print("In B init")
def feature2(self):
"""This is a method inside of our sub class."""
print("Feature 2 working...")
Output:-
In A init
In B init
Here both the constructors are called because we used the super method to call the constructor of the super-class.
The syntax of super:
super().method/attribute
This means we use super whenever we want to call super-class methods, attributes, and constructors with the same name.
It is commonly used to eliminate confusion between superclasses and subclasses that have methods with the same name.
MRO (method resolution order)
In case B inherits from two classes A and C. The leftmost class will be given priority while using the super method. This is method resolution order(MRO).
If you want to read about this in detail then. Click here. It’s python’s official documentation.
Conclusion
Finally, today we did a lot.
We saw what’s inheritance in python OOP with the help of the Person and the Iron man class.
Then we looked into its types by children-parent examples with images to ease our explanation.
Then we came across the super method and also briefly saw what’s MRO.
Challenge🧗♀️
Have you ever been to a bank?
I mean obviously, you have been and at least would know that we can open an account there to hold our cash in a bank.
Don’t get angry I just couldn’t come up with how to introduce the question. So I tried the TV ad thing. That’s it.🤷♀️
Your task is to create an Account class.
This class should have methods for:-
- creating an account,
- depositing cash,
- withdrawing cash, and
- knowing the balance in the account.
It should have three instance variables:
- account holder’s name,
- account balance, and
- password.
You must also know this already but banks require a password from you to proceed with transactions. You know like ATM pins.
Hope you learned something and enjoyed reading.😊
Ok so solve this challenge well and I’ll see you in the next post.
Till then take care. Bye-bye.👋🙋♀️
To read the official documentation on inheritance click here.
How could i subscribe for a blog website?
As of now, you can follow python-hub.com here on twitter. https://twitter.com/PythonHub_com
I wanted to write you this very small observation to be able to thank you very much again regarding the fantastic techniques you’ve shared in this article. It was surprisingly generous of you to offer extensively just what many individuals might have made available for an e-book to end up making some bucks for themselves, primarily seeing that you could possibly have tried it if you desired. The things also served as the good way to be aware that some people have the identical zeal just like mine to realize significantly more on the topic of this issue. Certainly there are some more pleasurable occasions ahead for folks who look over your blog.
Thanks a lot for taking your precious💎 time ⌚ to acknowledge… Stay tuned for more.😊
bookmarked!!, I love your web site!
Thanks a lot…
I discovered your weblog website on google and examine a number of of your early posts. Continue to maintain up the excellent operate. I just further up your RSS feed to my MSN Information Reader. Seeking forward to studying more from you afterward!…
Thank you very much! Surely stay tuned…
I don’t even know how I finished up right here, however I thought this submit was great. I do not recognise who you are however definitely you’re going to a well-known blogger for those who are not already 😉 Cheers!
Your style is so unique compared to many other people. Thank you for publishing when you have the opportunity,Guess I will just make this bookmarked.2
Thank you!!
I have been surfing on-line more than 3 hours as of late, yet I never discovered any interesting article like yours. It’s pretty price sufficient for me. In my view, if all site owners and bloggers made just right content as you probably did, the net shall be a lot more helpful than ever before.
Asking questions are genuinely good thing if you are not understanding something completely,
except this article presents fastidious understanding even.
Thank you!!