Hello Pythonistas, in this article I’ll provide a solution to the challenge I provided in this article.
At the end of that article, I presented a challenge to deepen your understanding of OOP by creating a library management system similar to the ‘Groot’ class.
Now, let’s explore this challenge and its solution step by step.
Contents
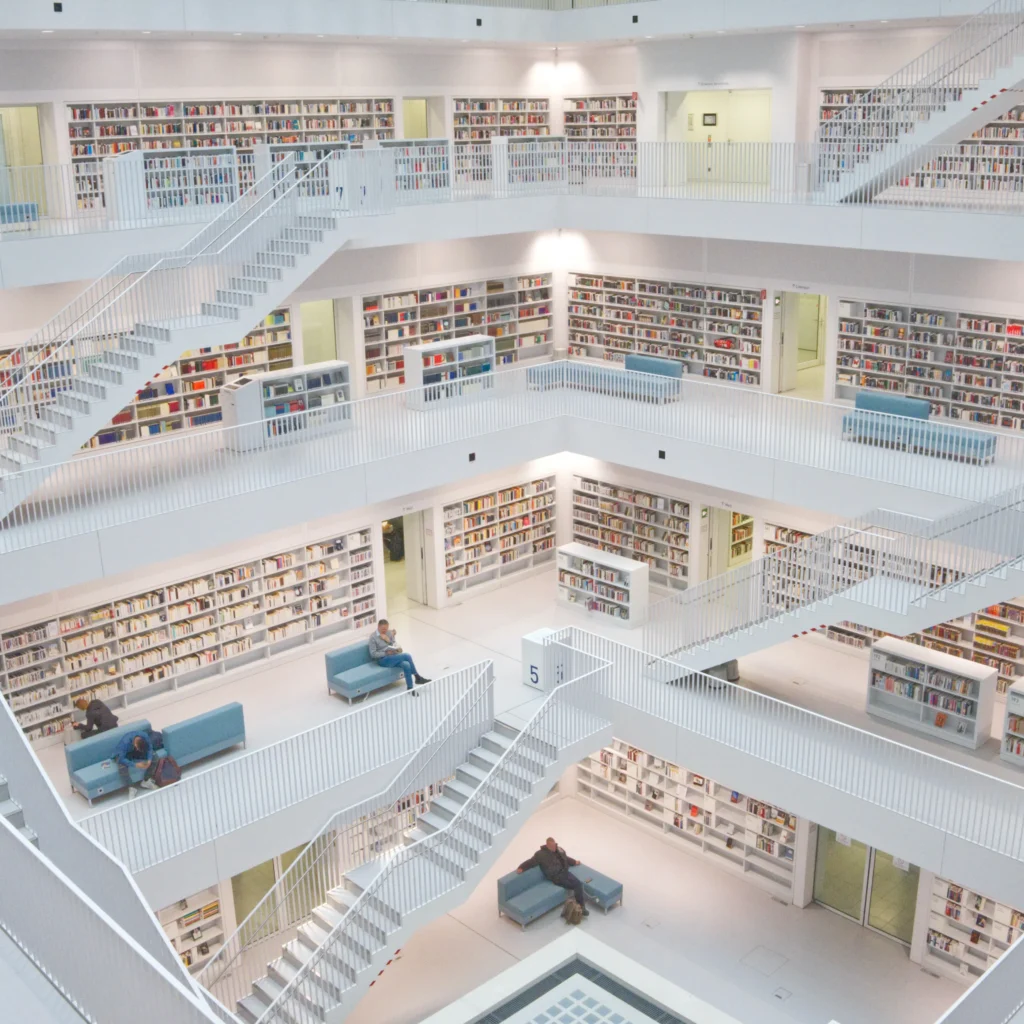
The Challenge
Your task is to design a class that manages a library, resembling the ‘Groot’ class discussed earlier.
The final version of this class will handle various library operations efficiently, i.e., should work as a Library Management System.
Feel free to rename the class but ensure it encapsulates the necessary functionalities.
The Solution: Library Management System
Below is the solution for the ‘LibBot’ class, designed to manage the library:
class LibBot:
def __init__(self, list, name):
self.booksList = list #list of books available in the library
self.name = name #name of the library
self.issueDict = {} #to store the books issued along with the issuer
def list_books(self):
print(f"\nThe following books are available in {self.name} library.")
for book in self.booksList:
print(book)
print()
def issue_books(self, issuer, book):
"""inputs the issuer and book.
checks whether taken by someone else(using the issueDict in the init).
If it is not then updates the issueDict.
If it is then says its already taken."""
if book not in self.issueDict.keys():
self.issueDict.update({book:issuer})
print("Issue-Book database has been updated. You can take the book now")
else:
print(f"Book is already being used by {self.issueDict[book]}")
def donate_books(self, book_name):
"""inputs book and adds it to the booksList.
And thanks the doner"""
self.booksList.append(book_name)
print("Book has been added to the book list.\nThanks for donating.")
def return_books(self, book):
"""pops the book from the issueDict dictionary."""
if book in self.issueDict.keys():
self.issueDict.pop(book)
print(f"{book} is returned successfully.")
else:
print("Check again. No such books were issued.")
Additionally, here’s the ‘Library’ class that utilizes the ‘LibBot’ class:
class Library:
def __init__(self,books,name):
self.memb_dict = {}
self.membNo = 0
self.bookList = books
self.name = name
self.library = LibBot(self.bookList, self.name)
def listBooks(self):
self.library.list_books()
def issueBook(self):
name = input("Enter your name: ")
book = input("Enter the Book's name: ")
if book in self.bookList:
self.library.issue_books(name, book)
else:
print("Sorry we don't have the book")
def donateBooks(self):
book = input("Enter the book name")
self.library.donate_books(book)
def returnBook(self):
book = input("Enter the book name")
self.library.return_books(book)
Lastly, the main code to interact with the library management system:
from booksAndLogo import python_books #I stored books in another file
olib = Library(python_books, "python-hub.com")
while True:
print("Welcome to the Python Library")
print("1. List of Books")
print("2. Donate Books")
print("3. Issue Books")
print("4. Return Books")
choice = int(input("What would you like to do?\n"))
if choice == 1:
olib.listBooks()
elif choice == 2:
olib.donateBooks()
elif choice == 3:
olib.issueBook()
elif choice == 4:
olib.returnBook()
elif choice == 5:
print("\n\nThanks for visiting!!")
break
else:
print("Invalid choice!!")
Feel free to explore this solution and dive deeper into each function’s workings. If you have any questions or need clarification, drop your queries in the comments below.