Welcome to Day Fourteen of my 21-day project series!
Today I made a Simple Text Editor In Python CTk.
This is just a step towards making a very useful editor like Notepad or VsCode.
Contents
Project Contents
This mini-project is a Simple Text Editor In Python CTk.
This mini-project allows users to create, open, and save text files.
It provides a user interface similar to Notepad, where users can perform common file operations such as creating a new file, opening an existing file, and saving the file with a chosen name.
And I have used customtkinter for GUI.
The project looks like this:
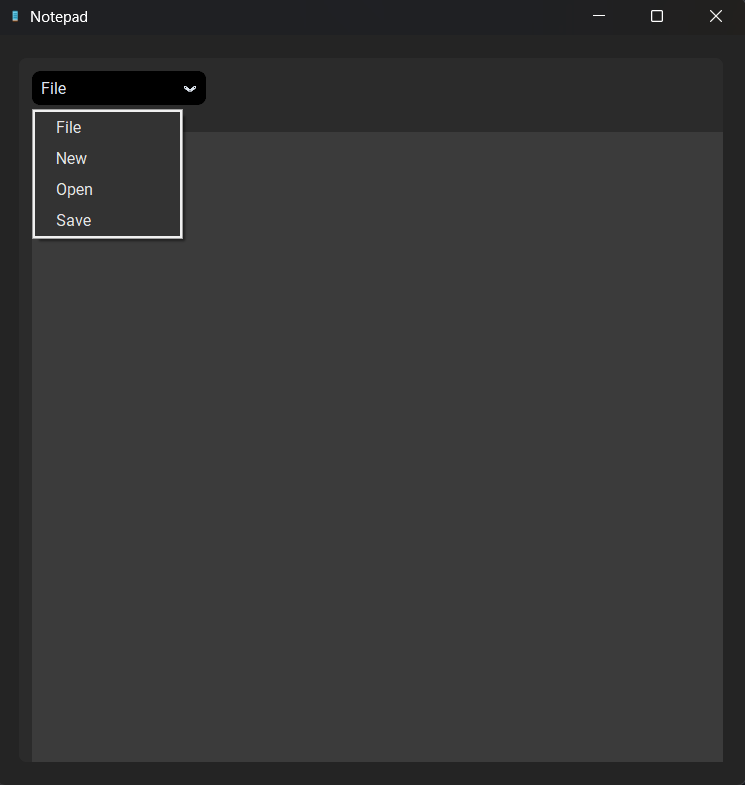
Full Source Code
Here’s the full source code of the Simple Text Editor In Python CTk.
from customtkinter import *
from tkinter.filedialog import askopenfilename, asksaveasfilename
class Notepad(CTk):
def __init__(self):
"""Initialize the Notepad application."""
super().__init__()
self.title("Notepad")
self.iconbitmap("images/notepad.ico")
self.geometry("600x600")
self.configure(padx=10, pady=10)
# file path
self.file = None
# Create a Frame for the menu and textarea
self.frame = CTkFrame(self)
self.frame.pack(fill="both", expand=True, padx=10, pady=10)
# file menu
self.File_var = StringVar(value="File")
self.filemenu = CTkOptionMenu(self.frame,values=["File", "New", "Open", "Save"],
command=self.file_menu_callback,
variable=self.File_var,button_color="black", fg_color="black")
self.filemenu.grid(row=0, column=0, padx=10, pady=10, sticky="w")
# Textbox to get text
self.textarea = CTkTextbox(self.frame, font=("Consolas", 11))
self.textarea.grid(row=1,column=0,columnspan=2, padx=10, pady=10, sticky="nsew")
# create CTk scrollbar
# It only appears in full screen mode
ctk_textbox_scrollbar = CTkScrollbar(self.frame, command=self.textarea.yview)
ctk_textbox_scrollbar.grid(row=1,column=1, sticky="ns")
# connect textbox scroll event to CTk scrollbar
self.textarea.configure(yscrollcommand=ctk_textbox_scrollbar.set)
# Bind the <Configure> event to the resize_textarea function
self.bind("<Configure>", self.resize_textarea)
# event is needed to bind it to resize event
def resize_textarea(self, event):
"""Adjust the size of the textarea based on the available space."""
# Calculate the new width and height for the textarea based on the available space
available_width = self.winfo_width() - 40 # Subtract the padding
available_height = self.winfo_height() - 40 # Subtract the padding
# Update the width and height of the textarea
self.textarea.configure(width=available_width, height=available_height)
def file_menu_callback(self,choice):
"""Handle the file menu commands."""
if choice=='New':
self.new()
elif choice=='Open':
self.open()
elif choice=='Save':
self.save()
def new(self):
"""Create a new file."""
self.file = None
self.title("Notepad")
self.textarea.focus_set()
self.textarea.delete("0.0", 'end')
def open(self):
"""Open an existing file."""
self.file = askopenfilename(defaultextension=".txt",
filetypes=[("All Files", "*.*"),
("Text Documents", "*.txt")])
if self.file == "":
self.file = None
else:
self.title(os.path.basename(self.file))
self.textarea.delete("0.0", 'end')
with open(self.file, 'r') as f:
self.textarea.insert("0.0", f.read())
def save(self):
"""Save the current file."""
if self.file == None:
self.file = asksaveasfilename(initialfile = 'Untitled.txt', defaultextension=".txt",
filetypes=[("All Files", "*.*"),
("Text Documents", "*.txt")])
if self.file =="":
self.file = None
else:
with open(self.file, 'w') as f:
f.write(self.textarea.get("0.0", "end"))
if __name__ == '__main__':
app = Notepad()
app.mainloop()
This Simple Text Editor In Python CTk is well documented read the code to understand it.
Here are the links to topics within the mini-project. You can check them if you have any doubts or confusion:
Possible Improvements (That I’ll add after 21 days)
Here’s the list of improvements that I’ll add after 21 days:
- Copy, Cut, and Paste
- Undo and Redo
- Find and Replace
- Font Formatting
- Word Wrap
- Multiple Tabs
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today, was very cool and calm. Not much stress. Also, there’s nothing much special about the day. But I would be happy to know about your experience for the day for sure…
Do let me know whether you liked this Simple Text Editor In Python CTk. Also, let me know if there are any suggestions.
And most importantly how was your DAY 14?
Cool and calm?
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…
Hurrah, that’s what I was looking for, what a material! existing here at this blog, thanks admin of this web page.
thank you!