Welcome to Day Fifteen of my 21-day project series!
Today I made A GUI Password Manager With Database Connectivity in Python.
I have a very very very useless memory. Cause I literally forget almost everything. And most of all passwords. So, I change passwords all the time.
To solve this I made this basic implementation of password manager.
Contents
Project Contents
This mini-project is A GUI Password Manager With Database Connectivity in Python.
This project involves creating a graphical user interface (GUI) for a password manager application using Python.
The application utilizes a MySQL database to store and retrieve passwords securely.
The GUI allows users to enter account information, including the account name, user ID, and password, which are then saved in the database.
Additionally, users can view the saved passwords, which are displayed in the GUI.
I have used customtkinter for GUI.
The project looks like this:
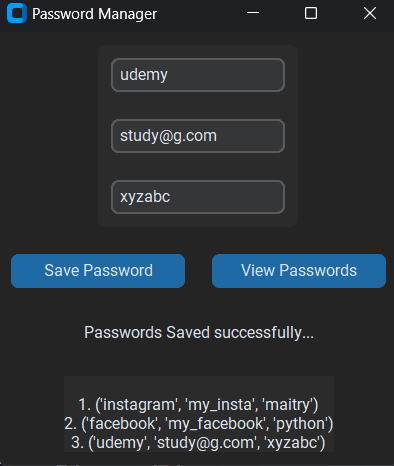
Full Source Code
Here’s the full source code of the GUI Password Manager With Database Connectivity in Python.
from customtkinter import *
import mysql.connector
class DatabaseMediator:
"""Handles the connection and interaction with the MySQL database."""
def __init__(self, host, user, password, database, table_name,*columns):
"""
Initializes the DatabaseMediator object.
Args:
host (str): The MySQL server host.
user (str): The username for the database connection.
password (str): The password for the database connection.
database (str): The name of the database.
table_name (str): The name of the table storing the passwords.
*columns (str): Variable-length argument list of column names in the table.
"""
try:
self.host = host
self.user = user
self.password = password
self.database = database
self.table_name = table_name
self.columns = columns
self.mydb = mysql.connector.connect(
host=self.host,
user=self.user,
password=self.password,
database=self.database
)
self.mycursor = self.mydb.cursor()
except Exception:
print("Cannot connect to the database!")
def insert_data(self, *args):
"""
Inserts data into the MySQL table.
Args:
*args: Variable-length argument list of values to be inserted.
"""
try:
self.mycursor.execute(f"INSERT INTO {self.table_name} ({', '.join(list(self.columns))}) VALUES {args}")
self.mydb.commit()
except Exception:
print("These values can't be inserted!!")
def retrieve_data(self):
"""
Retrieves all data from the MySQL table.
Returns:
list: A list of tuples representing the retrieved data.
"""
try:
self.mycursor.execute(f"SELECT * FROM {self.table_name}")
myresult = self.mycursor.fetchall()
return myresult
except Exception:
print("Couldn't retrieve data!")
class PasswordManager(CTk):
"""The main GUI class for the Password Manager application."""
def __init__(self):
"""Initializes the PasswordManager GUI."""
super().__init__()
self.title("Password Manager")
self.database_connection = DatabaseMediator("localhost", "root", "maitry", "password_manager", "passwords", "account_name", "account_id", "account_password")
self.frame = CTkFrame(self)
self.frame.grid(row=0, columnspan=2, padx=10, pady=10)
self.account_name = CTkEntry(self.frame, placeholder_text="Account name")
self.account_name.pack(padx=10, pady=10)
self.account_id = CTkEntry(self.frame, placeholder_text="Enter your user ID here")
self.account_id.pack(padx=10, pady=10)
self.account_password = CTkEntry(self.frame, placeholder_text="Enter your Password")
self.account_password.pack(padx=10, pady=10)
self.save_password = CTkButton(self, text="Save Password", command=self.save)
self.save_password.grid(row=1, column=0, padx=10, pady=10)
self.view_password = CTkButton(self, text="View Passwords", command=self.view)
self.view_password.grid(row=1, column=1, padx=10, pady=10)
def save(self):
"""Saves the password to the database."""
acc_nm = self.account_name.get()
acc_id = self.account_id.get()
acc_password = self.account_password.get()
self.database_connection.insert_data(acc_nm, acc_id, acc_password)
label = CTkLabel(self, text="Passwords Saved successfully...")
label.grid(row=2, columnspan=2, padx=10, pady=10)
def view(self):
"""Retrieves and displays all passwords from the database."""
passwords = self.database_connection.retrieve_data()
result = ""
for i in range(len(passwords)):
result += f"\n{i+1}. {passwords[i]}"
result_frame = CTkFrame(self)
result_frame.grid(row=3, columnspan=2, padx=10, pady=10)
res_label = CTkLabel(result_frame, text=result)
res_label.pack()
app = PasswordManager()
app.mainloop()
This GUI Password Manager With Database Connectivity in Python is well documented read the code to understand it.
Possible Improvements (That I’ll add after 21 days)
Here’s the list of improvements that I’ll add after 21 days:
- Security
- Error handling
- Clear Buttons
- Password hiding/showing
- Updating information
- Search Password Functionality
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today, was an interesting day. At least I loved it. Making this project I had a great time. And the first thing I would do after these 21 days end, is to make this a very good and secure web app.
It might take quite a long time, but it would be good for all of us. Right?
I am super excited to get started with it.
Do let me know whether you liked this GUI Password Manager With Database Connectivity in Python. Also, let me know if there are any suggestions.
And most importantly how was your DAY 15?
Exciting and interesting?
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…