Welcome to Day Twelve of my 21-day project series!
Today I made a Login Form GUI With CSV In Python.
This can help in securing all useful and functional apps…
Do read the experience…
Contents
Project Contents
This mini-project is a Login Form GUI With CSV In Python
This is a GUI login form that is connected to a CSV file to get usernames and passwords.
The structure of the CSV is like:
username, password
username1,password1
username2,password2
username3,password3
....
I have used the pandas library’s data frame to work with CSV. And I have used customtkinter for GUI.
The project looks like this:
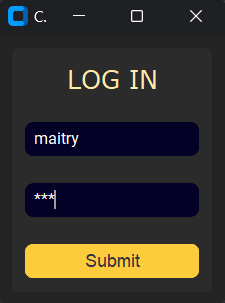
Full Source Code
Here’s the full source code of The Login Form GUI With CSV In Python.
from customtkinter import *
import pandas as pd
class LoginFrame(CTkFrame):
"""
A frame for login functionality.
"""
def __init__(self, master, **kwargs):
super().__init__(master, **kwargs)
"""
Initialize the LoginFrame.
Args:
master: The parent widget.
kwargs: Additional keyword arguments for the frame.
"""
# Create and grid the login label
self.log = CTkLabel(self, text="LOG IN", text_color="#ffe9a6", font=("Verdana",20))
self.log.grid(row=0, column=0,padx=10, pady=10)
# Create and grid the username entry field
self.user = CTkEntry(self, placeholder_text="Username", text_color="white", fg_color="#030126", border_color="#030126")
self.user.grid(row=1, column=0,padx=10, pady=10)
# Create and grid the password entry field
self.password = CTkEntry(self, placeholder_text="Password", show="*", text_color="white", fg_color="#030126", border_color="#030126")
self.password.grid(row=2, column=0,padx=10, pady=10)
# Create and grid the submit button
self.submit = CTkButton(self, text="Submit", fg_color="#fccc3d", text_color="#2e3140", hover=False, command=self.check, font=("arial",14))
self.submit.grid(row=3, column=0,padx=10, pady=10)
def check(self):
"""
Check if the entered username and password are valid.
"""
user = self.user.get()
if self.find_user(user):
passw = self.password.get()
if self.match_password(user, passw):
# Clear the app window and show success message
for widget in app.winfo_children():
widget.destroy()
label = CTkLabel(app, text="Successfully Logged In!!")
label.grid(row=1, column=1, padx=10, pady=10)
else:
# Show invalid password message
label = CTkLabel(app, text="Invalid Password")
label.grid(row=1, column=1, padx=10, pady=10)
else:
# Show invalid username message
label = CTkLabel(app, text="Invalid Username")
label.grid(row=1, column=1, padx=10, pady=10)
def find_user(self, username:str) -> bool:
"""
Find if a user with the given username exists.
Args:
username: The username to search for.
Returns:
bool: True if the username exists, False otherwise.
"""
df = pd.read_csv("users.csv")
for i in range(len(df)):
cuser = list(df.loc[i])[0]
if username ==cuser:
return True
else:
return False
def match_password(self, username:str, password:str) -> bool:
"""
Check if the given password matches the username.
Args:
username: The username to check.
password: The password to match.
Returns:
bool: True if the password matches, False otherwise.
"""
df = pd.read_csv("users.csv")
for i in range(len(df)):
cuser = list(df.loc[i])[0]
passw = list(df.loc[i])[1]
if username ==cuser:
if password == passw:
return True
return False
app = CTk()
frame = LoginFrame(master=app)
frame.grid(row=0, column=1,padx=10, pady=10)
app.mainloop()
This Login Form GUI With CSV In Python is well documented read the code to understand it.
Here are the links to topics within the mini-project. You can check them if you have any doubts or confusion:
Possible Improvements (That I’ll add after 21 days)
So, here are the possible improvements in the Login Form GUI With CSV In Python:
- Sign In option
- Forget password
- Logout
- Database Integration
- Better UI/UX
- Allow good enough passwords only
- Password Reset
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today I made this project in a hurry.
As I was not sure if I would be able to make it. At first, I wanted to make it with the flask module.
But as I have never tried flask before it seemed difficult and complicated.
Due to time constraints, I had to do it with GUI.
All in all, I had a tight schedule today because of a lot of assignments pending. I don’t know why but I always do stuff a day before submissions.
And somehow all the submissions are on the same day.
Do let me know whether you liked this Login Form GUI With CSV In Python. Also, let me know if there are any suggestions.
And most importantly how was your DAY 12?
Having a busy schedule??
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…