Welcome to Day Seventeen of my 21-day project series!
Today I have made a fun Adventure Game GUI In Python
It would be interesting to play it a few times… (For later you will know the result)
Do read the experience…
Contents
Project Contents
This mini-project is an Adventure Game GUI In Python
It is a very simple implementation of this game. And the coding style sucks.
Here’s how it will look:
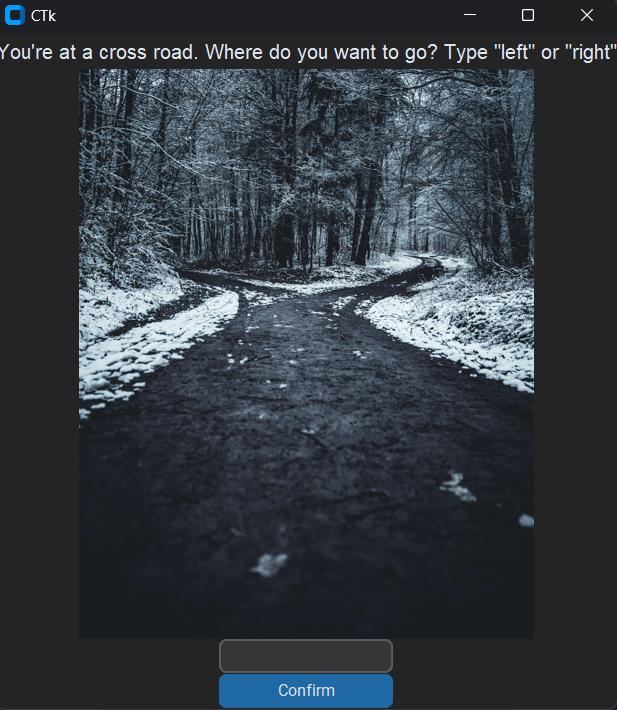
I made it from inspiration from a text-based project that I previously made while doing Angela Yu’s course on Udemy.
Full Source Code
Here’s the full source code of the Adventure Game GUI In Python.
Disclaimer: This is a disastrous coding style. Like, don’t even think of coding this way. Please don’t.🙏🙅♀️❌
from customtkinter import *
from PIL import Image
class adventure_game(CTk):
def __init__(self):
super().__init__()
self.images = [CTkImage(light_image=Image.open("Timages\\two roads.jpg"), size=(364,456)),
CTkImage(light_image=Image.open("Timages\\island.jpg"),size=(402,226)),
CTkImage(light_image=Image.open("Timages\\hole.jpg"),size=(595,396)),
CTkImage(light_image=Image.open("Timages\\Three doors.png"),size=(420,306)),
CTkImage(light_image=Image.open("Timages\\shark.jpg"),size=(400,266)),
CTkImage(light_image=Image.open("Timages\\fire.jpg"),size=(589,396)),
CTkImage(light_image=Image.open("Timages\\wolves.jpg"),size=(600,400)),
]
self.first_label = CTkLabel(self, text="You're at a cross road. Where do you want to go? Type \"left\" or \"right\"", font=("Arial", 16))
self.first_label.pack()
self.first_image = CTkLabel(self, image=self.images[0], text="")
self.first_image.pack()
self.choice1 = CTkEntry(self)
self.choice1.pack()
self.confirm = CTkButton(self, text="Confirm", command=self.choice_one)
self.confirm.pack()
def choice_one(self):
ch1 = self.choice1.get()
# Clear the app window
for widget in self.winfo_children():
widget.destroy()
if ch1 == "left":
self.second_label = CTkLabel(self, text="You've come to a lake. There is an island in the middle of the lake. Type \"wait\" to wait for a boat. Type \"swim\" to swim across.", font=("Arial", 16))
self.second_label.pack()
self.second_image = CTkLabel(self, image=self.images[1], text="")
self.second_image.pack()
self.choice2 = CTkEntry(self)
self.choice2.pack()
self.confirm = CTkButton(self, text="Confirm",command=self.choice_two)
self.confirm.pack()
else:
self.second_label = CTkLabel(self, text="You fell into a hole. Game Over.", font=("Arial", 16))
self.second_label.pack()
self.second_image = CTkLabel(self, image=self.images[2], text="")
self.second_image.pack()
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.pack()
def choice_two(self):
ch2 = self.choice2.get()
# Clear the app window
for widget in self.winfo_children():
widget.destroy()
if ch2 == "wait":
self.third_label = CTkLabel(self, text="You arrive at the island unharmed. There is a house with 3 doors. One red, one yellow and one blue. Which colour do you choose?", font=("Arial", 16))
self.third_label.pack()
self.third_image = CTkLabel(self, image=self.images[3], text="")
self.third_image.pack()
self.choice3 = CTkEntry(self)
self.choice3.pack()
self.confirm = CTkButton(self, text="Confirm",command=self.choice_three)
self.confirm.pack()
else:
self.third_label = CTkLabel(self, text="You get attacked by an angry trout. Game Over.", font=("Arial", 16))
self.third_label.pack()
self.third_image = CTkLabel(self, image=self.images[4], text="")
self.third_image.pack()
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.pack()
def choice_three(self):
ch3 = self.choice3.get()
# Clear the app window
for widget in self.winfo_children():
widget.destroy()
if ch3=="yellow":
self.fourth_label = CTkLabel(self, text="You win\nHere's the treasure:-\nIt's a comic website.\n-----------Happy Reading-----------")
self.fourth_label.pack()
import antigravity
from time import sleep
sleep(1)
self.quit()
elif ch3 == "red":
self.fourth_label = CTkLabel(self, text="It's a room full of fire. Game Over.", font=("Arial", 16))
self.fourth_label.pack()
self.fourth_image = CTkLabel(self, image=self.images[5], text="")
self.fourth_image.pack()
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.pack()
elif ch3 == "blue":
self.fourth_label = CTkLabel(self, text="You enter a room of beasts. Game Over.", font=("Arial", 16))
self.fourth_label.pack()
self.fourth_image = CTkLabel(self, image=self.images[6], text="")
self.fourth_image.pack()
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.pack()
else:
self.fourth_label = CTkLabel(self, text="Game Over.", font=("Arial", 16))
self.fourth_label.pack()
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.pack()
app = adventure_game()
app.mainloop()
This Adventure Game GUI In Python is not documented at all. It would be tough to understand it. But, I hope you guys have a way through it.
Search through the blog and even google if needed.
And of course needless to say do comments if you have any doubts regarding this or python or even programming in general.
Possible Improvements (That I’ll add after 21 days)
So, here are the possible improvements in the Adventure Game GUI In Python:
- A decent coding structure.
- New Environments and Challenges
- Exploration Rewards
- Different zones
- Side Quests
- Multiple Endings
- Mini-Games
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today, I had more time than any other day.
So, I decided to make a database-connected code snippet saver. It was going well and then confusions on confusions.
Cause I didn’t plan what to make, what can be the edge cases, etc. And that’s why I had to drop that idea—and had to start working on this one.
As a result, this has a disastrous coding style that I would have never used otherwise. The prompts should have been in a list like the images. Also, there should have been at max 2-3 functions to deal with the GUI.
It could have had an iterator or a counter.
It’s ok. One doesn’t get success on every try. Today I was meant to have a great project. But, this is what I have.🥺
Do let me know whether you liked this Adventure Game GUI In Python. Also, let me know if there are any suggestions.
And most importantly how was your DAY 17?
Ironic??
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…