Welcome to Day Four of my 21-day project series!
Today I’ll make Your Motivator With Python And Unsplash with a GUI interface in python.
Programming is not a straight easy path we all feel depressed at some or the other time. (And some like me would be depressed most of the time (just to say, It’s always))
I hope it helps us in our low time.
Contents
Project Contents
I’ll make a simple GUI interface that will use Unsplash’s free random-image-providing service to display motivational quotes in customtkinter GUI. Which looks like this:
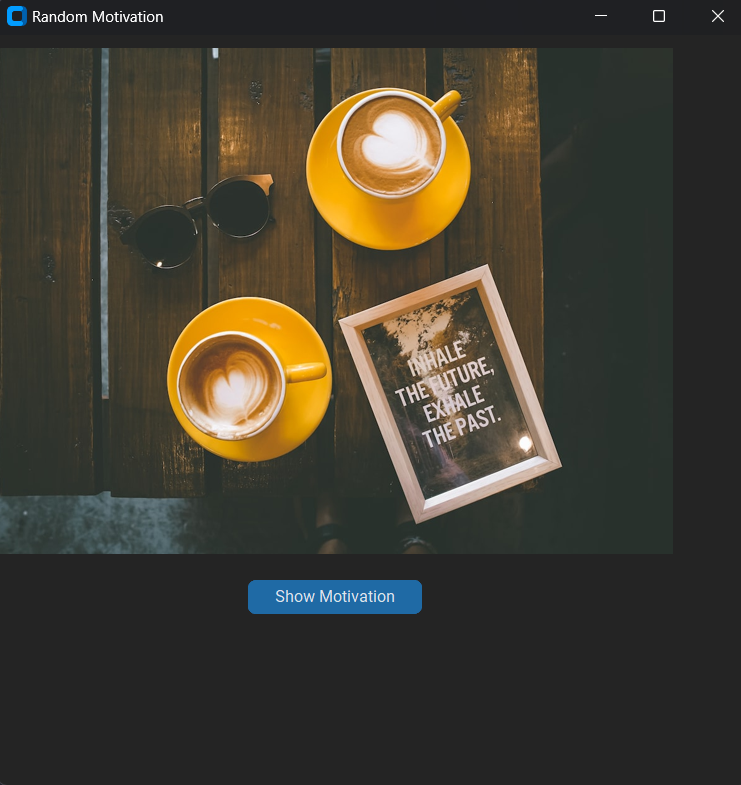
I wouldn’t get into the step-by-step explanation of the code as the article becomes super long. (And I guess boring too.)
But I would post an explanatory article for it soon.
Full Source Code
Here’s the full source code of Your Motivator With Python And Unsplash.
# https://awik.io/generate-random-images-unsplash-without-using-api/
# --------------------------------------------------------------
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from bs4 import BeautifulSoup
import requests
from customtkinter import *
from PIL import Image
from io import BytesIO
class Motivator(CTk):
"""A GUI application for displaying random motivational images. When you are sad."""
def __init__(self) -> None:
"""
Initialize the Motivator GUI.
Sets up the main window, question label, image label, and "Show Motivation" button.
"""
super().__init__()
self.title("Random Motivation")
self.geometry("600x600")
# getting image
self.question = CTkLabel(self, text="Are you sad? Click the button below.")
self.question.grid(row=0, pady=10)
self.sad_image = CTkImage(light_image=Image.open("images/sad.jpg"), size=(300,300))
# making image label
self.ilsad = CTkLabel(self, image=self.sad_image, text="")
# displaying image label
self.ilsad.grid(row=1, pady=10)
# Button to get a random motivational image
self.show_motivation = CTkButton(self, text="Show Motivation", command=self.show_image).grid(row=2, pady=10)
def get_img_url(self) -> str:
"""
Retrieve a random motivational image URL from Unsplash.
Returns:
str: The URL of the random motivational image.
"""
print("Getting image...")
print("Please wait...")
print("It might take a while...")
# configuring chrome options so that the browser doesn't open up
chrome_options = Options()
chrome_options.add_argument("--headless") # Run Chrome in headless mode (no GUI)
chrome_options.add_argument("--disable-gpu") # Required on some systems to avoid issues
# setting up selenium' chrome driver to get the web page
chrome_driver_path = "C:\Development\chromedriver.exe"
service = Service(executable_path=chrome_driver_path)
driver = webdriver.Chrome(service=service, options=chrome_options)
# getting the webpage
driver.get("https://source.unsplash.com/random/1080x810?motivational+quote")
# getting its source code
source = driver.page_source
# making a soup of the html code to parse through it well
soup = BeautifulSoup(source, 'html.parser')
# finding the source url of the image
img_url = soup.find('img')['src']
# quitting the driver (a good practice)
driver.quit()
print("Got the image showing it...")
return img_url
def show_image(self) -> None:
"""
Fetch and display the image got from self.get_img_url.
Uses the get_img_url method to fetch the image URL and displays it on the GUI.
"""
# getting url from the above function
img_url = self.get_img_url()
# getting the source code of the image's web page
response = requests.get(img_url)
# converting code of image to an image readable by python
image = Image.open(BytesIO(response.content))
# getting the image in customtkinter
img = CTkImage(image, size=(540,405))
print("showing the image...")
# showing the image with the use of label
label = CTkLabel(self, image=img, text='')
label.grid(row=1, pady=10)
if __name__ == "__main__":
app = Motivator()
app.mainloop()
The code is well documented and well written it would be easy to understand.
Here are the links to topics within the random motivator mini-project. You can check them if you have any doubts or confusion:
You would have to read on:
- Selenium module
- BytesIO
On your own as of now. I’ll try to publish their explanation as soon as possible.
Possible Improvements (That I’ll add after 21 days)
- Random Motivational Text (As Overlay The alt attribute can be used)
- Error handling (It is a must But I missed it due to time constraints)
- Copy Image URL
- Save the image
- About Page
- Light dark theme options
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
The first thing I improved on is designing the look and feel today. Just look at how good is Your Motivator With Python And Unsplash.
It looks like something I would want to use.
Moreover, this project wasn’t straightforward for me. As I had Pixabay in mind when I thought of the project, but later after around 30 mins research it clicked to me that it was Unsplash, not Pixabay.
This article helped me a lot when I knew what wanted. And obviously, who drinks even water these days without asking GPT or Google what’s the right way to do it?
Next, I tried for around 15 mins to get this done with the requests
module, and then the webbrowser
module.
Until I finally realized that was working with a dynamic webpage.
Then I went on to how to deal with the selenium
module cause I usually avoided it in the past as it seemed overwhelming.
Do let me know whether you liked Your Motivator With Python And Unsplash. Also, let me know if there are any suggestions.
And most importantly how was your DAY 4?
I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Try taking help from Your Motivator With Python And Unsplash.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too.