Welcome to Day Five of my 21-day project series!
Today I’ll make Dice Stimulator Python CTk GUI.
Now, this isn’t what I actually planned to make. But what I wanted to make didn’t happen. That’s why I had to make something in just 30 mins. So, this is all I could come up with.
Say, it was my low day today. Sorry, for this.
Read the experience for sure I guess it will help you a lot on your low day.
Contents
Project Contents
I’ll make a simple GUI interface that will display random no. on dice. It looks like this:
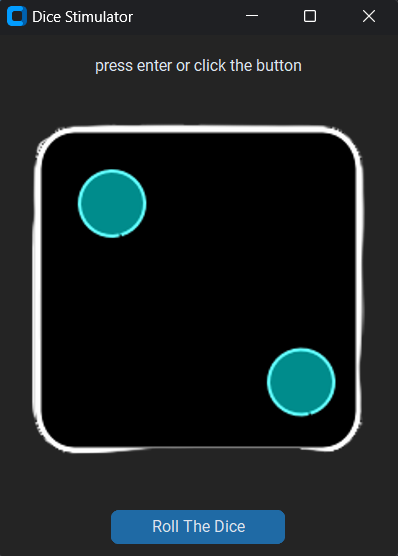
I wouldn’t get into the step-by-step explanation of the code as the article becomes super long. (And I guess boring too.)
But I would post an explanatory article for it soon.
Full Source Code
Here’s the full source code of Dice Stimulator Python CTk GUI.
from customtkinter import *
import random
from PIL import Image
class Dice(CTk):
def __init__(self):
super().__init__()
self.title("Dice Stimulator")
# Getting images into CTk
self.dice1 = CTkImage(light_image=Image.open("images/dice1.png"), size=(300,300))
self.dice2 = CTkImage(light_image=Image.open("images/dice2.png"), size=(300,300))
self.dice3 = CTkImage(light_image=Image.open("images/dice3.png"), size=(300,300))
self.dice4 = CTkImage(light_image=Image.open("images/dice4.png"), size=(300,300))
self.dice5 = CTkImage(light_image=Image.open("images/dice5.png"), size=(300,300))
self.dice6 = CTkImage(light_image=Image.open("images/dice6.png"), size=(300,300))
# putting them in a list for random choice
self.image_list = [self.dice1, self.dice2, self.dice3, self.dice4, self.dice5, self.dice6]
# Information
label = CTkLabel(self, text="press enter or click the button")
label.grid(row=0, padx=10, pady=10)
# Button to roll the dice
btn = CTkButton(self, text="Roll The Dice", command=self.roll)
btn.grid(row=2, padx=10, pady=10)
# Connecting button to the enter key
self.bind("<Return>", lambda event: btn.invoke())
def roll(self):
# choosing a random die face
img = random.choice(self.image_list)
# displaying it on the label
self.label = CTkLabel(self, text="", image=img)
self.label.grid(row=1, padx=10, pady=10)
if __name__ == "__main__":
app = Dice()
app.mainloop()
The code is not well documented today yet it is well written it would still be easy to understand.
Here are the links to topics within the Dice Stimulator Python CTk GUI. You can check them if you have any doubts or confusion:
Possible Improvements (That I’ll add after 21 days)
Personally, it’s a very simple project so I can’t think of many improvements in it.
However, it could be converted to a mobile app as no one would turn on a desktop for this.
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
So, this wasn’t the project I started with I started with a drink water reminder. That reminds the user to drink water every day at a specific time.
However, I noticed that I go on copy-pasting and don’t try to make the project on my own. It seemed that I was just in the race to finish the project as I didn’t want to miss out on the challenge.
I don’t think this is going to add any value to my skill set. I just keep prompting Gpt.
That’s why I shifted to a simpler project. I know it’s not great. But at least I did it on my own. I need to slow down and still be fast.
I promise to not go on making this challenge a race, that needs to be won by hook or crook.
Instead, I want it to be an improvement journey. Where I might not be perfect or awesome every time but better than I was yesterday.
Do let me know whether you liked this Mini silly dice stimulator Python CTk GUI. Also, let me know if there are any suggestions.
And if my thinking on the making is right?
And most importantly how was your DAY 5?
I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue from tomorrow.
And today just write one line of code as simple as making a variable or printing something. I couldn’t give my best today either.
Think about it: Ever felt lost? When you are doing everything but mindlessly. What do you do to change this???
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too.
You have a special talent for making even the most complex topics understandable and interesting.
Thanks a lot!