Welcome to Day Six of my 21-day project series!
Now, as a blogger, I at least need to convert 4-5 PNG to WEBP images. Though there are a lot of free tools online none of them convert images in bulk.
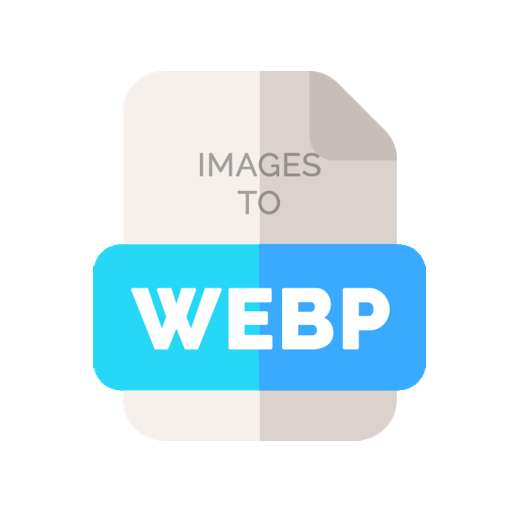
I understand they have security issues. But, it’s too long and frustrating for me. That’s why I decided to make a python script that can take up any amount of images from a folder and convert them all to WEBP.
This way all I need to do is download the images in that folder and I am done.
Contents
Project Contents
Now, I don’t want it to be any GUI. I simply want to press the run button and convert my PNG to WEBP.
Why?
Cause, WEBP’s are much more smaller and modern ways to have visual content. They load faster and take up much lesser space.
I’ll use Pillow
, os
, and pathlib
library for this purpose.
I wouldn’t get into the step-by-step explanation of the code as the article becomes super long. (And I guess boring too.)
But I would post an explanatory article for it soon.
Full Source Code
Here’s the full source code of PNG to WEBP Converter With Python.
from PIL import Image
import os
from pathlib import Path
def compress_image(image_path:str) -> None:
'''Takes up a PNG file, compresses it and converts it to a WEBP.
Uses the pillow, pathlib and os module to do this.
Args:
image_path: The path to the image (str)'''
try:
compression_level = 6
img_nm = Path(os.path.basename(image_path))
compressed_png_path =Path(f"images_webp/{img_nm}")
if not(os.path.exists(compressed_png_path.with_suffix(".webp"))):
# opening image
img = Image.open(Path(image_path))
# compressing image
img.save(compressed_png_path, format="png", optimize=True, compress_level=compression_level)
# opening compressed image
img_compressed = Image.open(compressed_png_path)
# Convert the compressed PNG to WebP
img_compressed.save(compressed_png_path.with_suffix(".webp"), format="webp")
# removing the compressed PNG
os.remove(compressed_png_path)
else:
print("File already exists")
except FileNotFoundError as e:
print(f"Error: {e}")
paths = Path("images").glob("**/*.png")
for path in paths:
compress_image(str(path))
The code is well documented and well written it would be easy to understand.
There is nothing much tough in this. So, I am not linking up any posts.
However, this can help you in understanding the Pillow library a bit.
At least, as much as is required for understanding this.
Possible Improvements (That I’ll add after 21 days)
Personally, it’s a very simple project so I can’t think of many improvements in it.
It works great for my requirements. However, you can customize it for yourself.
This article helped me.
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
So, I was tired of converting my images time and again. That’s why I thought of making this.
At first, I thought I’ll make a complicated GUI project from this which would have all the features to deal with files and folders.
But, on second thought it seemed as long as it solves problems it doesn’t need to be awesome looking.
(Wow what lines! 😅I talk maturely at times)
Do let me know whether you liked this Mini PNG to WEBP Converter With Python or not. Also, let me know if there are any suggestions.
And if my thinking on the making is right?
And most importantly how was your DAY 6?
I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…