Welcome to Day Ten of my 21-day project series!
Today I have made for you (and me) Your Own Gallery in Python.
It’s a great relief to just scroll through your gallery when you need a break.
And when you can do that without losing any data to any external app. It’s even better.
Contents
Project Contents
This mini-project is Your Own Gallery in Python.
It will look like this: (I called it Reminisce beforehand that’s why)
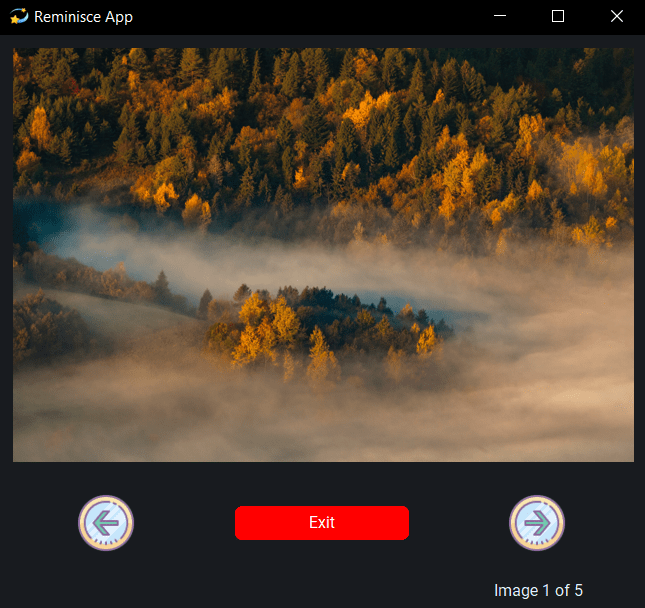
The user can keep going through their favorite photographs continuously.
It provides an interactive and convenient way to get lost in your memories…
I have used the customtkinter library for this GUI. And the Pillow library for images.
You can get the full explanation here:
Full Source Code
Here’s the full source code of A Random Wikipedia Article Generator GUI in Python.
from customtkinter import *
from PIL import Image
class App(CTk):
def __init__(self):
super().__init__()
self.title("Personal Gallery")
self.iconbitmap("images\\gallery.ico")
self.configure(fg_color="#181b1f")
# setting up counter and images
self.counter = 0
# getting all the images in a list
# If you have a lot of them, I suggest store them in a different file
self.images = [CTkImage(light_image=Image.open("images\\forest.jpg"),size=(496.9,331.3)),
CTkImage(light_image=Image.open("images\\moon.jpg"),size=(390.6,260.4)),
CTkImage(light_image=Image.open("images\\mountain.jpg"),size=(654.1,436.1)),
CTkImage(light_image=Image.open("images\\stone.jpg"),size=(593.7,416)),
CTkImage(light_image=Image.open("images\\tree.jpg"),size=(624,416))]
# images for buttons (forward and backward buttons)
self.forward_img = CTkImage(light_image=Image.open("images\\forward.png"), size=(50,50))
self.backward_img = CTkImage(light_image=Image.open("images\\backward.png"), size=(50,50))
# label to show images
self.il = CTkLabel(self,text="",image=self.images[self.counter])
self.il.grid(row=0, column=0, columnspan=3, padx=10, pady=10)
# buttons
self.front = CTkButton(self, text="", image=self.forward_img, fg_color="transparent", hover_color="#181b1f", command=self.next_command)
self.back = CTkButton(self, text="", image=self.backward_img, fg_color="transparent", hover_color="#181b1f", command=self.previous)
self.front.grid(row=1, column=2, padx=10, pady=10)
self.back.grid(row=1, column=0, padx=10, pady=10)
# exit button
self.qb = CTkButton(self, text="Exit", fg_color="red", hover_color="pink", text_color="white", command=self.quit)
self.qb.grid(row=1, column=1, padx=10, pady=10)
# status bar
self.status = CTkLabel(self, text=f"Image {self.counter + 1} of {len(self.images)}")
self.status.grid(row=2, column=2, padx=25)
def next_command(self):
# To keep counter from being more than 4
# (4+1)%5 = 0
# ensures cycle
self.counter = (self.counter + 1) % len(self.images)
self.update()
def previous(self):
# To keep counter from being less than 0
# (0-1)%5 = 4
# ensures cycle
self.counter = (self.counter - 1) % len(self.images)
self.update()
def update(self):
# removes label from the grid
self.il.grid_forget()
# updating image
self.il = CTkLabel(self,text="",image=self.images[self.counter])
self.il.grid(row=0, column=0, columnspan=3, padx=10, pady=10)
# updating status bar
self.status = CTkLabel(self, text=f"Image {self.counter + 1} of {len(self.images)}")
self.status.grid(row=2, column=2, padx=25)
if __name__ == '__main__':
app = App()
app.mainloop()
Your Own Gallery in Python is well documented however it has no docstring. Yet it is easy to understand. And I have provided a link to its explanation in case you face any trouble in understanding it.
Possible Improvements (That I’ll add after 21 days)
So, here are the possible improvements in Your Own Gallery in Python:
- Slideshow functionality
- Album selection
- Favorite images
- Social sharing functionality
- User authentication and authorization
- User Preferences (light-dark mode)
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today, my brain and PC both pledged to be hanged. They just didn’t seem to work.
And honestly, I don’t know why did that happen to my PC cause I haven’t done troubleshooting yet.
But, for my brain, I guess it’s cause I have a lot of options and a lot of things to do and I can’t decide what to do. Everything seems right and wrong.
Does that happen to you?
There’s a lot going on in my head today. And when something like this happens you always end up thinking about pleasant memories.
Not to find solutions just to have an escape so that you can simply get your head at peace. Just to know that it all gets solved in the end.
That’s why I made this gallery app to reminisce all the beautiful memories when I am confused. (For a trip every week is quite costly)
I have just added 5 sample images you can link up an entire folder and load it in a list using list comprehension to get more images.
Do let me know whether you liked this Your Own Gallery in Python. Also, let me know if there are any suggestions.
And most importantly how was your DAY 10?
Confused like me?
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…