Welcome to Day Nine of my 21-day project series!
Today I have made A Random Wikipedia Article Generator GUI.
I am a curious kid so I like to read new stuff that is not based on my past preferences like in social media. That’s why I decided to make it.
Contents
Project Contents
This mini-project is a Random Wikipedia Article Generator GUI.
It will look like this:
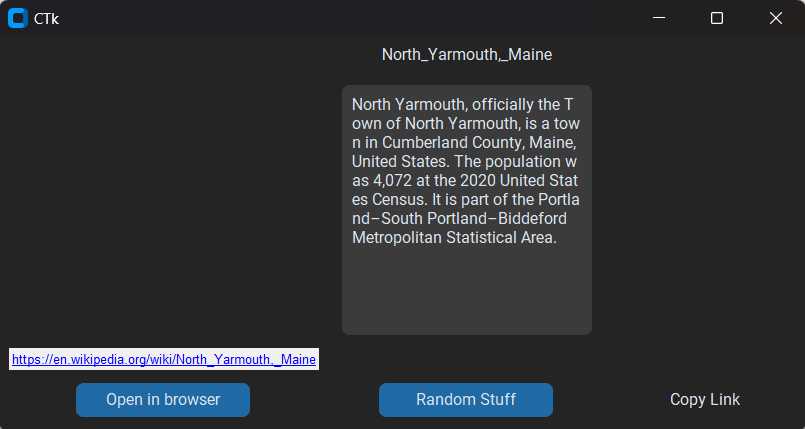
Users can generate a random article with the click of a button, view its title and summary, and even copy the article’s link or open it in a web browser.
It provides an interactive and convenient way to explore diverse topics and expand one’s knowledge using the extensive resources available on Wikipedia.
List of libraries:
- requests
- wikipedia
- customtkinter
- tkinter -> Label
- tkinter.messagebox
- tkinter.font
- webbrowser
I guess I did a lot of imports.
Full Source Code
Here’s the full source code of A Random Wikipedia Article Generator GUI in Python.
import requests
import wikipedia
from customtkinter import *
from tkinter import Label
import tkinter.messagebox as messagebox
import tkinter.font as tkfont
import webbrowser
class WikipediaArticle(CTk):
def __init__(self):
"""Initialize the WikipediaArticle application."""
super().__init__()
# font for link
self.custom_font = tkfont.Font(size=10, underline=True)
# setting an empty label to avoid errors
self.link = CTkLabel(self, text="")
# button for generating random article
self.get_article = CTkButton(self, text="Random Stuff", command=self.random_article_t_summary)
self.get_article.grid(row=3, column=1 ,padx=10,pady=10)
# button to copy article link
self.copy_t = CTkButton(self, text="Copy Link", command=self.copy_text, fg_color="transparent")
self.copy_t.grid(row=3, column=2 ,padx=10,pady=10)
# button to open link in browser
self.open_i = CTkButton(self, text="Open in browser", command=self.open_link)
self.open_i.grid(row=3, column=0 ,padx=10,pady=10)
def random_article_t_summary(self):
"""Generate a random Wikipedia article and display its title, summary, and link.
uses the self.summarize_wikipedia_article() method"""
url = requests.get("https://en.wikipedia.org/wiki/Special:Random")
article_link = url.url
title_and_summary = self.summarize_wikipedia_article(article_link)
if title_and_summary:
# getting link and title
self.link = Label(self, text=article_link, fg="blue", font=self.custom_font)
self.title = CTkLabel(self, text=title_and_summary[0])
# getting summary
self.random_article = CTkTextbox(self)
self.random_article.insert("0.0", title_and_summary[1])
self.random_article.configure(state="disabled")
# packing title, summary, and link
self.title.grid(row=0, column=1, padx=10)
self.random_article.grid(row=1, column=1 ,padx=10,pady=10)
self.link.grid(row=2, column=0, padx=10)
def summarize_wikipedia_article(self,article_link):
"""
Summarize a Wikipedia article based on its link.
Args:
article_link (str): The link to the Wikipedia article.
Returns:
list: A list containing the page title and summary of the article, or None if the article is not found.
"""
# Extract the page title from the article link
page_title = article_link.split("/")[-1]
try:
# Get the Wikipedia page based on the page title
page = wikipedia.page(page_title)
# Retrieve the summary of the page
summary = page.summary
return [page_title,summary]
except wikipedia.exceptions.PageError:
message = CTkLabel(self, text="Something went wrong! Try Again!")
message.grid(row=4, column=1,padx=10, pady=10)
return None
def copy_text(self):
# Get the text from the label
text = self.link.cget("text")
# Set the text to the clipboard
self.clipboard_clear()
self.clipboard_append(text)
messagebox.showinfo("Copy", "Text copied to clipboard!")
def open_link(self):
try:
webbrowser.open(self.link.cget("text"))
except Exception:
message = CTkLabel(self, text="Get the link first!")
message.grid(row=4, column=1,padx=10, pady=10)
if __name__ == "__main__":
app = WikipediaArticle()
app.mainloop()
Possible Improvements (That I’ll add after 21 days)
So, here are the possible improvements in the random Wikipedia article generator GUI in Python:
- Article Categories
- Article Images
- Favorite Articles
- Language Selection
- Article Sharing
- Article History
- User Preferences
And a lot more…
Experience of the day
In case you don’t know this project is a part of the brand new series that I have started. #21days21projects in python. To read more about it you can check out this page.
And for those who already know here’s my experience of the day.
Today I had a hard time thinking about what to make. I couldn’t understand what to do. Completely confused. So, I googled for ideas…
And I didn’t get anything good.
Somehow randomly I got a previous project list and decided to do this—a random Wikipedia article generator GUI.
It was an excellent experience. I thought I would need to iterate through something to get the randomization.
However, after some searching, I got to know that Wikipedia itself provided this facility.
All in all, it was a very good day. I had fun and did work too.
Do let me know whether you liked this Mini Calculator GUI with Python or not. Also, let me know if there are any suggestions.
And most importantly how was your DAY 9?
Chilled like mine?
Well, I hope it was fun. And remember it’s perfectly awesome if you missed out. Continue to work from tomorrow.
And today just write one line of code as simple as making a variable or printing something.
Your suggestions are welcomed!
Happy coding…
And wait, if you have some ideas for the upcoming projects do let me know that too. Even when the series ends do let me know what you like…