Hello Pythonistas, welcome back.
Let’s take a quick walkthrough of the most used methods of list in Python.
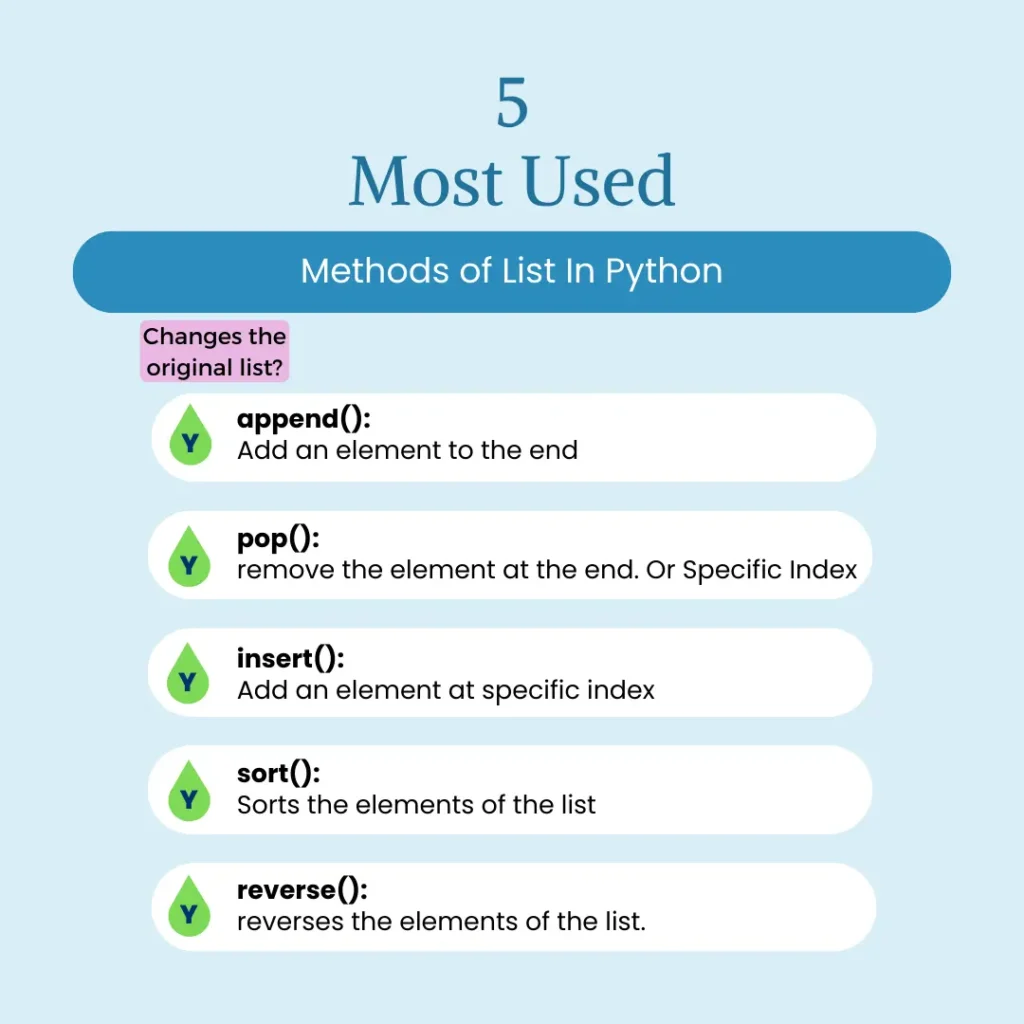
Contents
reverse()
Original list:
shopping_list = ['tomatoes', 'veggies', 'nuts', 'nutella']
The shopkeeper is quite mechanical. He does the stuff as ordered without giving any second thought.
Because you don’t want your Nutella jar to juice out your tomatoes you want to reverse the list.
shopping_list = ['nutella', 'nuts', 'veggies', 'tomatoes']
Here’s where the reverse()
function will give you a helping hand and save you from the typing efforts (because you are a lazy, smart kid 👶👩💻 ).
This is how to use this function:-
#shopping list you created
shopping_list = ['tomatoes', 'veggies', 'nuts', 'nutella']
print(f"original list:- {shopping_list}")
#reversing the list
shopping_list.reverse()
print(f"reversed list:- {shopping_list}")
Output:
original list:- ['tomatoes', 'veggies', 'nuts', 'nutella']
reversed list:- ['nutella', 'nuts', 'veggies', 'tomatoes']
methods of list
append()
This is one of the most used, among the methods of list.
Now, you suddenly realize that you are not a vegan🥦🍽🥦 and can drink milk 🥛 and you want to add milk to your list.
The append()
function will help you out here.
You just need to type this:-
shopping_list.append("milk")
print(f"list after appending:- {shopping_list}")
Output:-
list after appending:- ['mayonnaise', 'nuts', 'veggies', 'fruits', 'milk']
pop()
Once again a problem you hate milk 🥛 and want to remove ❌ it from your list. (I hope 🤞 you don’t get these many mood swings in real life😜)
But, no need to worry our superhero 🦸♂️ pop()
is here
shopping_list.pop()
print(f"list after pop's attack:- {shopping_list}")
Output:-
list after pop's attack:- ['mayonnaise', 'nuts', 'veggies', 'fruits']
pop(index)
pop()
by default removes the last index but if you want to remove a specific value then write its index number inside the function.
Like, say you have a nut allergy and want to remove nuts. Then just type:-
shopping_list.pop(1)
print(f"list after pop's attack:- {shopping_list}")
Output:-
list after pop's attack:- ['mayonnaise', 'veggies', 'fruits']
insert()
After so many changes in the list, you are feeling that the list has become too short and you won’t get any discount for this small amount of shopping.
So, you decide to add one more item to be able to qualify ☑ the minimum amount after which you can get a discount.
insert()
comes to help you here:-
shopping_list.insert(2, "chocolate")
print(f"list after insert's aid:- {shopping_list}")
Output:-
list after insert's aid:- ['mayonnaise', 'veggies', 'chocolate', 'fruits']
Inside the insert()
function first, you need to put the index number at which you want to insert the value, and then, you need to put a comma, and then, give the value you want to insert.
sort()
You just struck that the shopkeeper always takes the list in a sorted manner(first a, then, b then, c, and so on…). To solve this the sort()
function comes into the game.
shopping_list.sort()
print(f"list after sorting:- {shopping_list}")
Output:-
list after sorting:- ['chocolate', 'fruits', 'mayonnaise', 'veggies']
methods of list methods of list
If you want to know more methods of list read the official documentation.