Hello Pythonistas, welcome back.
Today, we will see if lists in python are mutable. And if yes, then how is that useful or possibly harmful?
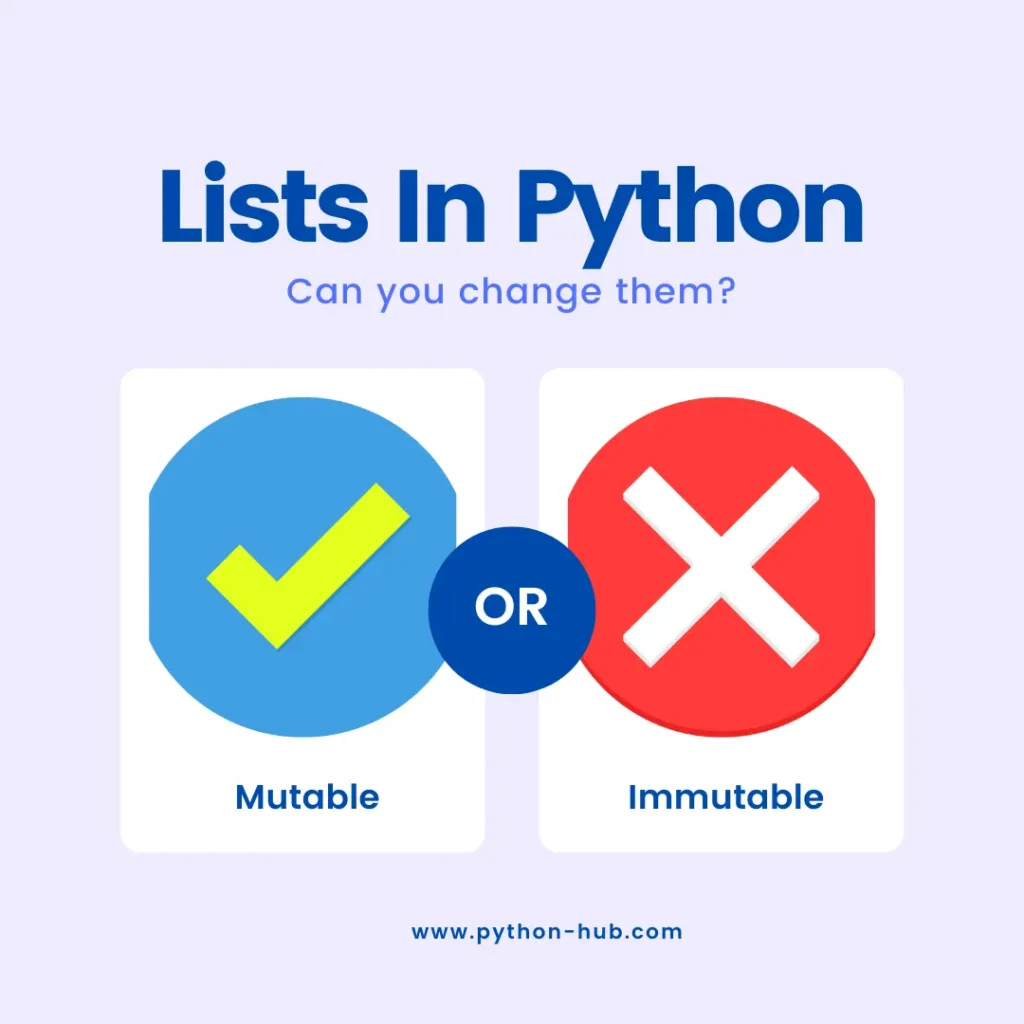
Contents
Are lists mutable
In one word yes they are. Lists in python are mutable.
This means that you can easily change the list once created.
Say for example you are a lazy, smart kid πΆπ©βπ» just like me, so you made a shopping list and stored it in python.
shopping_list = ["fruits", "veggies", "nuts", "milk"]
Now, you gave your drone that shopping list, so that it can give it to the shopkeeper π¬ as you usually do.
But, you added milk to the list instead of mayonnaise.
You are not worried about this because lists in python are mutable so you just did this:-π
#shopping list you created
shopping_list = ["fruits", "veggies", "nuts", "milk"]
print(f"list before:- {shopping_list}")
#changing the list
shopping_list[3]= "mayonnaise" #this simply means that you
#are changing the value of index 3 from milk to mayonnaise
print(f"list after:- {shopping_list}")
Output:-
list before:- ['fruits', 'veggies', 'nuts', 'milk']
list after:- ['fruits', 'veggies', 'nuts', 'mayonnaise']
Not just this, you can even add or remove elements at runtime.
How Is Mutability Helpful?
This feature helps you add, remove, and update items on runtime.
1. Simplifying Logic:
- Imagine updating a shopping list: adding items, changing quantities, marking things off. With mutability, you simply modify the existing list, mimicking real-world behavior.
- This is much simpler than creating new lists for every tweak.
2. Efficient Data Manipulation:
- Need to rearrange a list quickly?
- Mutability allows methods like
sort
orreverse
to modify the original list directly, saving memory and computation compared to recreating it from scratch.
3. Dynamic Algorithms:
- Mutability opens the door to algorithms that adapt and update themselves.
- Think of simulations constantly changing data or building blocks snapping together to form new structures. This flexibility wouldn’t be possible with immutable data.
4. Concise Code:
- Modifying existing data often requires fewer lines of code than creating new versions. This results in cleaner, more readable code, especially for iterative tasks.
However, remember that mutability is a double-edged sword. Unexpected changes can lead to errors or unintended consequences.
Therefore, it’s essential to use it with caution and employ strategies like clear variable names and data validation to maintain control and clarity.