Welcome back, Pythonistas🙋♀️! I hope you’re all doing well.
Have you transferred data through the web🌐 as a programmer? If you have then you already know how important JSON is. If you don’t you will by the end of this article.😉
Today we will learn how to work with Javascript Object Notation files in Python. I will keep it crisp🤏 and precise so you can jump straight into using it.
So, without further ado, let’s dive💦🏊♀️ right in!
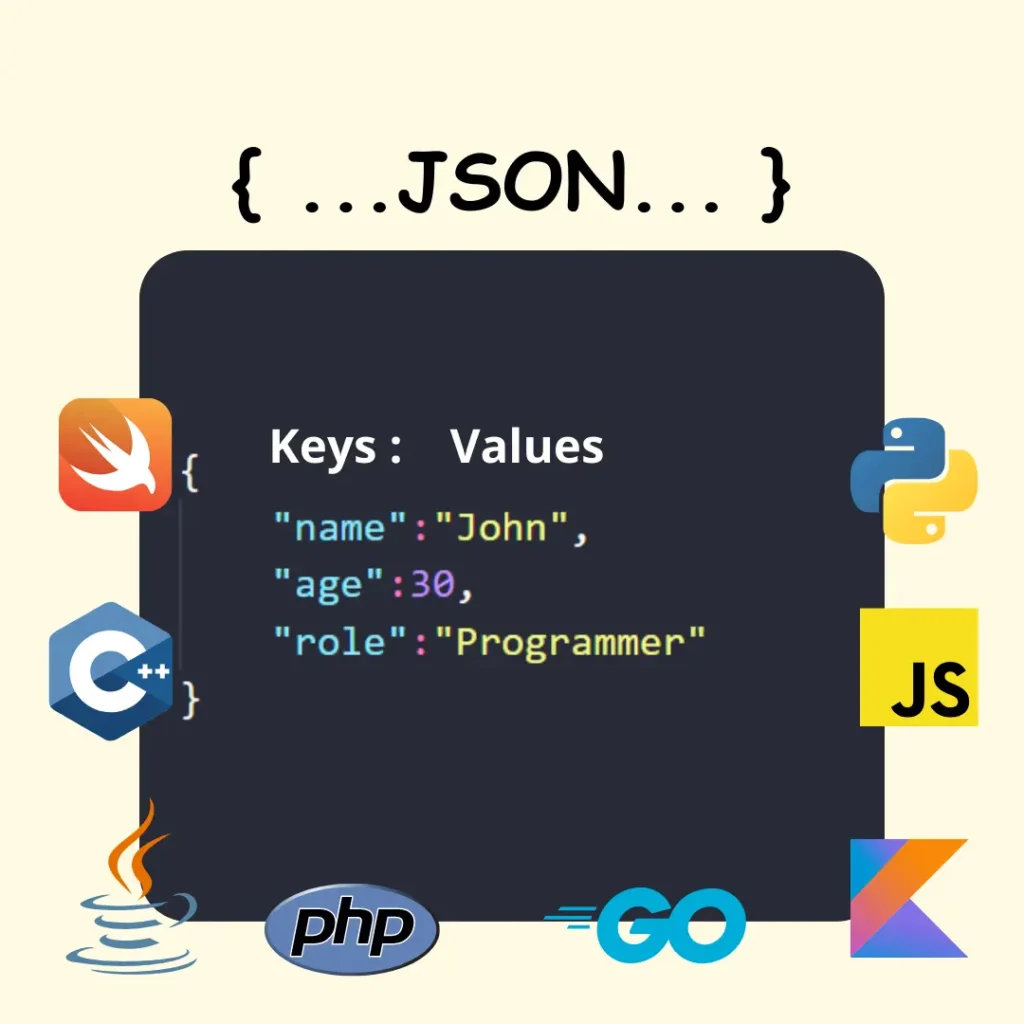
Contents
Previous post’s challenge’s solution
Here’s the solution 🧪 to the challenge provided in the last post:
def factory(task_name):
"""
Factory function that returns a decorator for adding a notification message after executing a task.
Args:
task_name (str): The name of the task to be displayed in the notification message.
Returns:
function: The notify decorator.
"""
def notify(fx):
"""
Decorator that adds a notification message after executing the decorated function.
Args:
fx: The function to be decorated.
Returns:
function: The wrapper function that includes the notification message.
"""
def wrapper(*args, **kwargs):
"""
Wrapper function that executes the decorated function and prints the notification message.
Args:
*args: Variable-length argument list.
**kwargs: Arbitrary keyword arguments.
"""
fx(*args, **kwargs)
print(f"{task_name} checked!")
return wrapper
return notify
Please read the comments in the code to understand it clearly.👍 If you still have any doubts ask them in the comment section below.👇
What is JSON?
The first and foremost question is what🤷♂️ is JSON? It is a data structure that enables one to store💾 data in a key🔑-value pair format. (like a dictionary)
And yeah, it stands for Javascript Object Notation.
- Javascript: is a lightweight programming language usually used as client-side scripting language in frontend web🌐 development. (not an important term though)
- Object: is a data structure in Javascript similar to a Python🐍 dictionary.
- Notation: the specific syntax and rules used to represent data in a standardized format.
So, it is a data structure of Javascript written in a specific format. However, it is language-independent.
It is written something like this:
{
"name": "John",
"age": 30,
"city": "New York"
}
You must be wondering🤔 why a data structure of another language needs to be used in Python.
That too when we have dictionary📚 data structure in Python?
Why and When to Use It?
We hire Javascript’s data structure because it is a universal🌎 data interchange format.
Heavy word, I know. It means that Javascript Object Notation is used worldwide to exchange data.
It is used for transferring ↔️ and storing 💾 data for various purposes: configuring files, storing data, and working with web APIs.
The reason for this hype is two✌️-fold:
- The data format is easy to read 🤓 for humans,
- It is also efficient for systems 💻 to parse through.
Almost all programming languages provide built-in support for it.
Using it in Python offers several advantages:
- Speed 🏎️
- Language independence
- Easy dealing 🤝 with web technology.
Say you made a game 🎮 in Python and used JSON to store data. You might want to implement the same game in C++ as Python is not as fast as it is. In this case, you just need to write the game logic 🧠 in C++ syntax. You can use the same file.
These are just a few. There are ample benefits of using this data structure.
This means time to ditch 💔 the Python dictionary.
NO, it doesn’t.
Both of them have a different use case.
Use Dictionary | Use JSON |
---|---|
When temporary data storage is needed. | For long term data storage. |
In Python-centric programs. | When you have a language-independent program. |
If the data structure will be complex. | In web development as it is a web-friendly data structure. |
Let’s see how to handle this data structure using Python’s built-in JSON module.
JSON to Python (read)
The first thing that we are going to explore is how we can use existing JSON data.
The data can be:
- string 🧵, or
- file 📁
Let’s start out with a string🧵.
loads()
First of all, import the module:
import json
So, in some cases you might have JSON data in a Python string like this:
emp_data = '''
{
"employees": [
{
"name": "John",
"id": 1,
"Salary": 5000
},
{
"name": "Emily",
"id": 2,
"Salary": 3000
},
{
"name": "Michael",
"id": 3,
"Salary": 2000
},
{
"name": "Sophia",
"id": 4,
"Salary": 4000
}
]
}
'''
It is like a nested 📚 dictionary. Say, I gave you a task to give $100💵 as a bonus to all the employees.
One way could be changing by typing ⌨️ in the updated amounts.
Another and better one is to turn it into a Python dictionary and then run a loop ➰ to update values.
To load value from a JSON string🧵:
emp_dict = json.loads(emp_data)
Note: it is loads not load.
Now, we have the data in a dictionary📚 we can simply update the salaries💵 like this:
#going through all the employees
for i in range(len(emp_dict["employees"])): #list inside dictionary
#updating salary of all employees
emp_dict["employees"][i]["Salary"] += 100
# dict[list][index][key]
#printing the updated data
print(emp_dict)
Great we are done👍. But, what if we were given this data in a file📁?
load()
For this, open the file using the with open block:
with open('employee.json', 'r') as f:
Access and convert the data to a Python dictionary using the load()
function:
with open('employee.json', 'r') as f:
emp_dict= json.load(f)
Note: it is load not loads this time.
Now, you can do the same with this emp_dict
too.
Python to JSON (write)
What if I asked you to provide this data back to me in JSON format this time, instead of simply printing 🖨️ it?
You can give data in the form of:
- string, or
- file
dumps()
To return it in string format you can use the dumps()
function:
updated_emp_data = json.dumps(emp_dict, indent=2)
print(updated_emp_data)
Here the indent
parameter is to provide output in a properly spaced format.
Note: it is dumps not dump.
dump()
Now, to return data in file format:
We will again open a file but this time in write mode and then will use the dump()
function to write it in the file:
with open("employee.json", "w") as f:
json.dump(emp_dict, f, indent=2, sort_keys=True)
The sort_keys
parameter is to give the output data in sorted order. You can use it in dumps()
too.
Okay so with this we come to an end of this post.
Conclusion
In this article, we learned about JavaScript Object Notation and how to work with these files📁 in Python🐍.
We explored reading JSON data from strings and files using the loads()
and load()
functions respectively, and saw how to convert Python dictionaries to JSON using dumps()
and dump()
.
It’s essential to know that this is not all there’s more to it. You can explore more about it in the official doc. Official documentation.
Challenge 🧗♀️
Your challenge is to make a simple task manager🧑💼 app interface class using JSON.
The class should be like this:
class TaskManager:
def __init__(self):
pass
def add_task(self, task):
pass
def delete_task(self, task):
pass
def view_task(self, task):
pass
The file should be like this:
{
"make calculator": {
"deadline":"06-06-20xx",
"status":"completed"
},
"practice questions": {
"deadline":"012-06-20xx",
"status":"pending"
},
"TaskName": {
"deadline":"date",
"status":"completed|pending"
}
}
Happy solving…
Stay happy 😄 and keep coding and do suggest any improvements if there. Take care and have a great 😊 time I’ll see you soon in the next post…Bye Bye👋