a Random Password Generator in Python using CustomTkinter
Do you often find yourself using passwords like “123abc,” your name, or even your pet’s name?
If so, you’re unintentionally making things easier for hackers to crack your secrets!
But worry not—today, we’ll build a Random Password Generator using Python and CustomTkinter.
This tool will generate strong, unique passwords that are difficult to crack.
In the next article, we’ll also explore how to store these passwords securely, so you won’t even need to remember them.
Contents
Import the Required Modules
First, we need to import customtkinter
and other essential modules:
from customtkinter import *
import random
import string
Initialize the Main Application Window
Create the main application window and set its properties like title and size:
class PasswordGeneratorApp(CTk):
def __init__(self):
super().__init__()
self.title("Random Password Generator")
self.geometry("400x250")
self.configure(bg_color="white")
self.create_widgets()
def create_widgets(self):
# Password display label
self.password_label = CTkLabel(self, text="Generated Password", font=("Helvetica", 16))
self.password_label.pack(pady=10)
# Password entry
self.password_entry = CTkEntry(self, width=300)
self.password_entry.pack(pady=10)
# Password length label
self.length_label = CTkLabel(self, text="Password Length:", font=("Helvetica", 12))
self.length_label.pack(pady=5)
# Password length entry
self.length_entry = CTkEntry(self, width=50)
self.length_entry.insert(0, "12") # Default length
self.length_entry.pack(pady=5)
# Generate button
self.generate_button = CTkButton(self, text="Generate Password", command=self.generate_password)
self.generate_button.pack(pady=20)
Implement the Password Generation Logic
Next, let’s add the logic to generate a random password. We’ll create a method to handle this functionality:
def generate_password(self):
try:
# Get the desired password length from the entry
length = int(self.length_entry.get())
if length < 1:
raise ValueError("Password length must be at least 1")
# Define the character set
characters = string.ascii_letters + string.digits + string.punctuation
# Generate the password
password = ''.join(random.choice(characters) for _ in range(length))
# Display the generated password
self.password_entry.delete(0, ctk.END)
self.password_entry.insert(0, password)
except ValueError:
self.password_entry.delete(0, ctk.END)
self.password_entry.insert(0, "Invalid length")
Running the Application
Finally, we need to create an instance of our PasswordGeneratorApp
and start the main event loop:
if __name__ == "__main__":
app = PasswordGeneratorApp()
app.mainloop()
Sample Output: Random Password Generator in Python using CustomTkinter
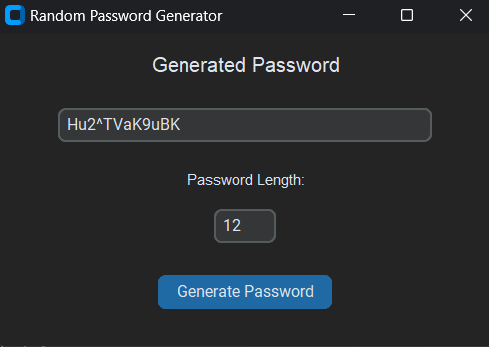
Full Source Code: a Random Password Generator in Python using CustomTkinter
Click for the full source code
from customtkinter import *
import random
import string
class PasswordGeneratorApp(CTk):
def __init__(self):
super().__init__()
self.title("Random Password Generator")
self.geometry("400x250")
self.configure(bg_color="white")
self.create_widgets()
def create_widgets(self):
# Password display label
self.password_label = CTkLabel(self, text="Generated Password", font=("Helvetica", 16))
self.password_label.pack(pady=10)
# Password entry
self.password_entry = CTkEntry(self, width=300)
self.password_entry.pack(pady=10)
# Password length label
self.length_label = CTkLabel(self, text="Password Length:", font=("Helvetica", 12))
self.length_label.pack(pady=5)
# Password length entry
self.length_entry = CTkEntry(self, width=50)
self.length_entry.insert(0, "12") # Default length
self.length_entry.pack(pady=5)
# Generate button
self.generate_button = CTkButton(self, text="Generate Password", command=self.generate_password)
self.generate_button.pack(pady=20)
def generate_password(self):
try:
# Get the desired password length from the entry
length = int(self.length_entry.get())
if length < 1:
raise ValueError("Password length must be at least 1")
# Define the character set
characters = string.ascii_letters + string.digits + string.punctuation
# Generate the password
password = ''.join(random.choice(characters) for _ in range(length))
# Display the generated password
self.password_entry.delete(0, END)
self.password_entry.insert(0, password)
except ValueError:
self.password_entry.delete(0, END)
self.password_entry.insert(0, "Invalid length")
if __name__ == "__main__":
app = PasswordGeneratorApp()
app.mainloop()
Challenge 🧗
Try adding one or more of the following features to improve your password generator:
Password Strength Indicator: Implement a password strength indicator that shows how strong the generated password is based on length and character diversity.
Include/Exclude Character Types: Add checkboxes to include or exclude certain character types, such as uppercase letters, digits, or special characters.
Copy to Clipboard: Add a button to copy the generated password to the clipboard for easy pasting.
a Random Password Generator in Python using CustomTkinter a Random Password Generator in Python using CustomTkinter