Only two parts are there. This is the first. The second will be linked below.
Hello Pythonistas🙋♀️, welcome back to python-hub.com.
Do you know what python is like a powerful genie👼 it can help you create whatever you want.
Today we will discuss a powerful tool🛠 it provides you which is in python just like numbers are in maths. Most basic yet most important.
Along with this, I have given an exciting challenge at the end which will use all the python skills you have learned by now.
(if you want just the challenge go to the next part of this post.)
Contents
Previous post’s challenge’s solution
Before we get started here’s the solution to the challenge in the previous post:
Solution Link: Click here For the solution.
Did you notice something new about the string above?🤔
It is enclosed in triple quotes.
This is an example of a multiline string.
More on this further in this post.
Explanation of the code:-
- Now to create the required program we first went on to create the necessary variables.
- Next, we created a multiline string.
- Lastly, we made it an f-string and added the variables to that string.
OUTPUT:
Click here to run the code and view output.
What are strings in python?
You have been using strings since the very 👆first program.
There I simply stated that it is a data type that includes characters like a,b,c… and special characters like @,$,&… inside single or double quotations.
That’s completely correct.✔
Let’s understand strings more clearly in technical terms.
Do you remember 🤔everything in python is an object?
These objects belong to some class. Objects of the same class have the same properties.
The string is a class. And all the strings that you create are objects of that class.
This is similar to a normal class the room is a class and tables, chairs, lights💡, windows, blackboards, etc. are objects of that class. You will become more clear with this when you will understand OOP(object-oriented programming).
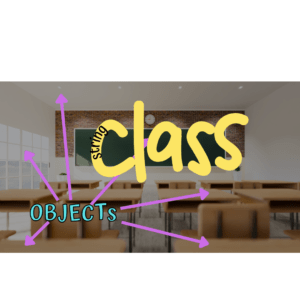
This might sound👂 different from the standard definition. Which is:- Strings in Python are arrays of bytes representing Unicode characters
Here, array means that python views the strings you give like this:-
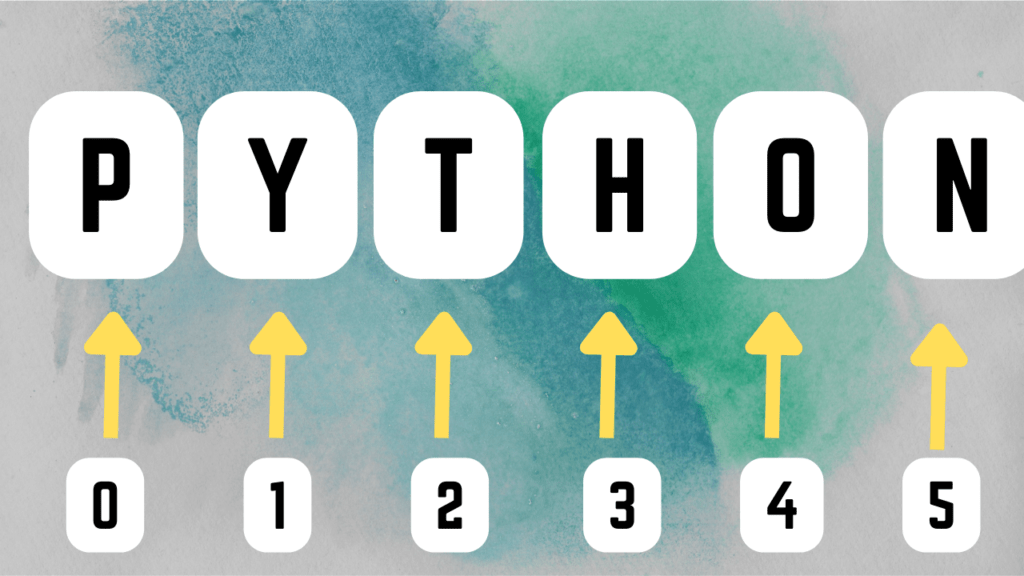
And Unicode is simply an encoding and decoding standard accepted worldwide by a number of programming languages.
If you want more details either watch this video or read this Wikipedia page I found both of them really useful.
types of strings
There are three3️⃣ types of strings in python:
- single line,
- multiline, and
- empty.
single-line
Consider this like a toothpaste box 📁that can contain only one tube.
Single line strings end in one single line, or even a single word or a character.
Example:
print("I love python") #here, I love python is a single line string.
print('l') #l
print('python') #python
Output:-
I love python
l
python
multiline
The second is multiline strings consider a carton📦 that can contain a lot of toothpaste tubes.
These occupy more than one line just like the one you saw in the beginning.
Here’s another example of it:
print('''this is a
multiline
string''')
Output:
this is a
multiline
string
One thing to note✍ here is you can also use double triple quotes for multiline strings(""" """
like this).
Do you remember I said(ok wrote) there is something called unassigned strings in this post?
That meant it is not compulsory to assign a string to a variable or use it inside of the print function. You can use it outside freely in the program.
"This is an unassigned string."
However, it won’t display any output and also wouldn’t be available to use until assigned to a variable or used inside the print function.
empty
Consider these as small showpiece box, that cannot contain anything.
Empty strings do not have any content inside of them that’s why they are called empty.
For example:-
print("")
#NOTE: They don't even have white spaces.
Where to use single, double, and triple quotes
Now, you might be confused🤔 ok got this but I still doubt where to use single, double, and triple quotes.
The answer is pretty simple, you can use any of the quotes for a single line and an empty string in python.
It is compulsory to use triple quotes for multiline strings.
Letter = 's'
One_sentence_str = "Use double quotes for me"
Multi_str = '''you do not have
any other options for me'''
It is better if you use single quotes for empty and one-character strings. And use double quotes for a word or a sentence. The triple quotes should ideally be used for multi-line strings.
Indexing
This is a completely new concept for you right now. Understand this carefully as it would be useful in a lot more data types.
As I mentioned earlier python does not see the strings the same as you do. It views strings in the form of an array. Each element inside the array has a number that represents its position in the array. This is string indexing.
Giving every element in a string a position. These positions start from 0
for strings.
For example:
str1 = "I am happy"
print(str1[0]) # I
print(str1[1]) # (whitespace)
print(str1[2]) # a
print(str1[3]) # m
print(str1[4]) # (whitespace)
print(str1[5]) # h
print(str1[6]) # a
print(str1[7]) # p
print(str1[8]) # p
print(str1[9]) # y
Now I hope you got what’s string indexing. Always remember this, indexing in a string starts from 0
.
accessing and slicing
Accessing
All the elements in a string can be individually accessed this is called accessing strings. Sounds interesting? Let’s see how:
str1 = "I am happy"
print(str1[0]) # I
print(str1[1]) # (whitespace)
print(str1[2]) # a
print(str1[3]) # m
print(str1[4]) # (whitespace)
print(str1[5]) # h
print(str1[6]) # a
print(str1[7]) # p
print(str1[8]) # p
print(str1[9]) # y
Yes, you got it right you already know this, it is possible because of string indexing.
You just need to put the index number of the element you want to access inside square brackets after the variable name/string.
You can also do it without a variable like this:-
print("I am happy"[0]) # I
print("I am happy"[2]) # a
print("I am happy"[4]) # (whitespace)
slicing
But what if I want to access the word happy
out of it?🤔 No worries your genie python will bring 'happy'
for you using string slicing. This simply means getting a part of a string. This is how:-
str1 = "I am happy"
print(str1[5:10:1]) #happy
The syntax for this is inside the square brackets you first need to mention where to start, then where to stop, and finally how many letters are to be skipped in between.
A few things to remember here are:-
- Stop value should be given one step ahead of the actual one. Meaning in the above example
'y'
has index number 9 but we need to write 10 if we want to include'y'
. - The step value can not be zero. If don’t want to skip just give one or keep it empty. Like we can also write the code above as
print(str1[5:10])
- If you want to start from the beginning you can leave the first value empty. Like this:-
print(str1[:10])
OUTPUT:-I am happy
. - Also, if you want to end the slice at its actual ending you can keep the middle value empty. Like this:-
print(str1[5::2])
OUTPUT:-hpy
.
You can also give negative indexes it starts from the back. For example, I am happy
is a string then y
will have -1
as the index value which you can use accordingly.
Let’s see if there is any way to change the strings created.
are they mutable?
In one word no, strings aren’t mutable. They are immutable which means they cannot be changed.
This means if you give something like this:-
language = 'Pytjon'
language[3] = 'h'
It will give an error.
Traceback (most recent call last):
File "", line 1, in
language[3] = 'h'
TypeError: 'str' object does not support item assignment
However, there is no real necessity to change the strings already created.
And if in any way necessary you can use an in-built function of string replace
.
Here’s how:-
language = language.replace('j', 'h')
print(language) #'Python'
conclusion
Here in this post, we learned
- what are strings,
- their types,
- their use,
- their indexing,
- their accessing, and their slicing,
- and got to know that they are immutable.
We will continue the topic in the next post and the challenge will also be posted there. I did this because the post was getting way too long to read, understand, and apply.
Don’t dishearten yourself because here in this post I am giving you some challenges to practice your string slicing. Which will make you ready for the big challenge.
challenge🧗♀️
Today’s challenge would include a few questions to be solved. The questions are:-
Q1
Slice the word until first “e”.(Typ)
word = 'Typesetting'
ans1 =
print(ans1)
#Output should be Typ
Q2
Get “sum” out of the string. (sum)
find_sum = 'Lorem Ipsum'
ans2 =
print(ans2)
#Output should be sum
Q3
Get “can”.
wrd="Toscana"
ans3 =
print(ans3)
#Output should be can
Q4
Can you slice the word from beginning to the end with steps of 2 excluding the last character.)?
word="standard"
ans4 =
print(ans4)
#Output should be sad
Q5
Slice the word to get “tan”.
word="standard"
ans5 =
print(ans5)
#Output should be tan
Go fast. I am waiting. Comment your answers below.
This was it for the post. Comment below suggestions if there and tell me whether you liked it or not. Do consider sharing this with your friends.
See you in the next post till then have a great time.😊
To read the next post click here.
You are so awesome! I do not believe I have read anything like that before. So nice to discover another person with a few genuine thoughts on this topic. Really.. thanks for starting this up. This web site is something that’s needed on the internet, someone with some originality!
Thanks a lot, Dave Griego. Stay tuned for more