Tkinter Frame Explained With Example
Hey hey! 👋
So, you’ve been building with Tkinter—labels here, buttons there…
But suddenly, it’s all looking like spaghetti.
Let me introduce you to your new best friend: Frame
.
It helps you keep your layout neat and organized—just like folders on your desktop.
Contents
What is a Frame in Tkinter?
A Frame is like a box or container.
You can put widgets inside it, group them together, and control them as one unit.
It’s super handy when you have lots of stuff on your app.
Think of it as:
“I want all these widgets to stick together.”
So, you throw them into a Frame!
Tkinter Frame Explained With Example Tkinter Frame Explained With Example
Creating a Basic Tkinter Frame
Here’s a clean and simple way to use a frame:
import tkinter as tk
root = tk.Tk()
root.title("Using Frames")
root.geometry("300x200")
frame = tk.Frame(root, bg="lightblue")
frame.pack(pady=20)
label = tk.Label(frame, bg="lightblue", text="I am in a blue box")
label.pack(padx=20, pady=20)
root.mainloop()
What’s going on here?
- We created a main window.
- Then, we added a light blue frame to it.
- Inside that frame, we put a label that says “I am in a blue box.”
- The label has extra padding around it, so it doesn’t look squished.
This is the simplest way to group widgets and give them a cozy space to hang out.
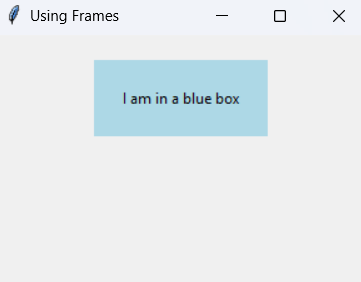
Why Use Frames?
Frames are perfect when:
- You want to group related widgets
- You’re building different sections (like a header, sidebar, footer)
- You want better control of layout inside layout
Let’s Make a Fancy Layout with Frames
Check this out—3 frames doing their thing:
import tkinter as tk
root = tk.Tk()
root.title("Using Frames")
root.geometry("300x200")
top = tk.Frame(root, bg="lightgray", height=50)
top.pack(fill="x")
left = tk.Frame(root, bg="lightgreen", width=100)
left.pack(side="left", fill="y")
main = tk.Frame(root, bg="white")
main.pack(side="left", expand=True, fill="both")
header_lbl = tk.Label(top, text="Header")
header_lbl.pack(pady=20)
side_lbl = tk.Label(left, text="Sidebar")
side_lbl.pack(padx=20)
main_lbl = tk.Label(main, text="Main")
main_lbl.pack()
root.mainloop()
Tkinter Frame Explained With Example Tkinter Frame Explained With Example
Boom! Now you’ve got a header, sidebar, and main content area.
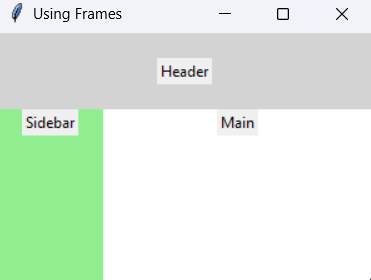
Nest Frames for Ultimate Control
Yes—you can put frames inside other frames.
Like folders in folders!
import tkinter as tk
root = tk.Tk()
root.title("Using Frames")
root.geometry("300x200")
outer = tk.Frame(root, bg="black", padx=10, pady=10)
outer.pack()
inner = tk.Frame(outer, bg="white", width=150, height=100)
inner.pack()
root.mainloop()
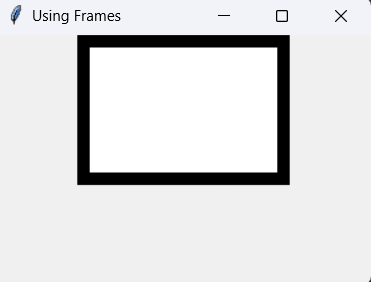
Using grid() or place() Inside a Frame
Frames don’t care how you layout their kids—you can use pack()
, grid()
, or place()
inside.
Example using grid()
inside a frame:
import tkinter as tk
root = tk.Tk()
root.title("Using Frames")
root.geometry("350x250")
form = tk.Frame(root)
form.pack(pady=10)
tk.Label(form, text="Frame 1 in Grid").grid(row=0, column=0, padx=5, pady=5)
tk.Label(form, text="Username").grid(row=1, column=0, padx=5, pady=5)
tk.Entry(form).grid(row=1, column=1, padx=5, pady=5)
tk.Label(form, text="Password").grid(row=2, column=0, padx=5, pady=5)
tk.Entry(form, show="*").grid(row=2, column=1, padx=5, pady=5)
form2 = tk.Frame(root)
form2.pack()
tk.Label(form2, text="Frame 2 with Pack").pack()
tk.Label(form2, text="Username").pack()
tk.Entry(form2).pack()
tk.Label(form2, text="Password").pack()
tk.Entry(form2, show="*").pack()
root.mainloop()
Tkinter Frame Explained With Example Tkinter Frame Explained With Example
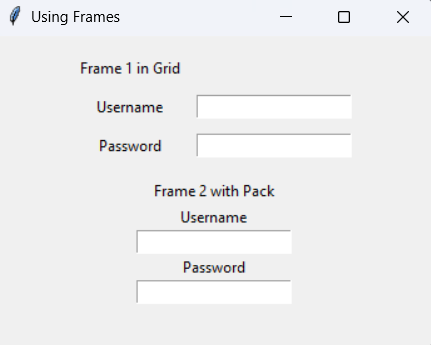
Frame Styling Tips
You can style your frames too:
bg
: background colorbd
: border sizerelief
: border style (flat
,sunken
,ridge
,groove
,raised
)width
andheight
: to set exact size
Wrap Up: Frame is Your Layout Superpower
You’ve now unlocked the secret sauce of clean Tkinter UIs.
With Frame
, your app won’t just work—it’ll look good too.
Stack ‘em, nest ‘em, style ‘em…
And watch your GUI go from messy to marvelous. 💪